Dealing with Memory Issues When Testing Large Input Code
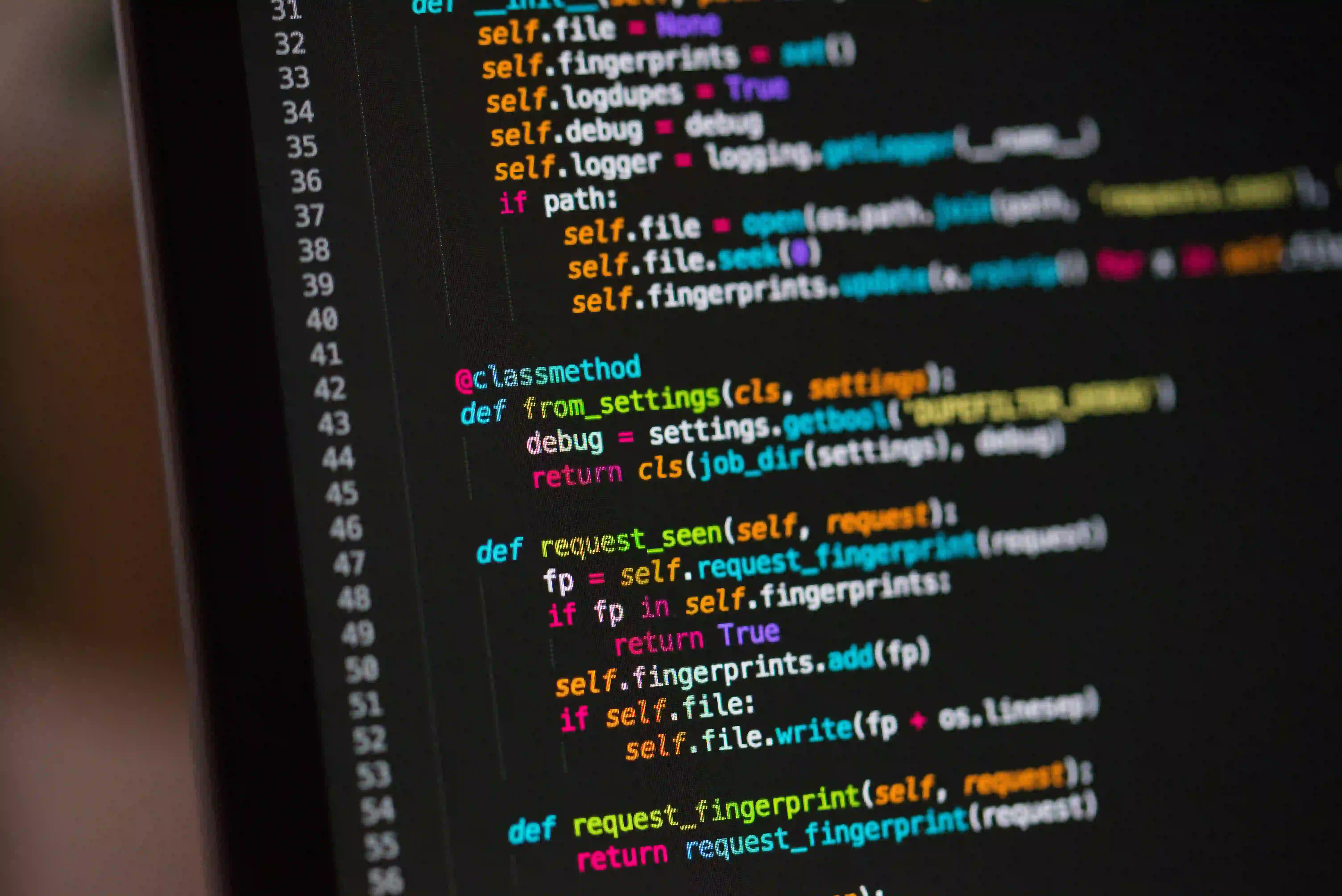
Dealing with Memory Issues When Testing Large Input Code in Java
When it comes to Java programming, tackling memory issues during testing is a pervasive challenge, particularly when dealing with large input sizes. This blog post will walk you through the best practices for handling memory effectively, common pitfalls to avoid, and specific strategies that can save your application from running out of memory.
Understanding Java Memory Management
Java manages memory through a system known as the Java Virtual Machine (JVM). Understanding how the JVM allocates and manages memory is critical when working with large data inputs.
JVM Memory Structure
The JVM's memory is divided into the following areas:
- Heap Memory: This is where all the Java objects are allocated. It's further divided into the young generation and old generation.
- Stack Memory: Each thread gets its own stack space for storing method calls and local variables.
- Method Area: This area stores class-level information.
- Native Method Stack: This is used for native method calls using Java Native Interface (JNI).
Understanding these areas plays a crucial role in effectively managing memory consumption in your Java applications and tests.
Common Memory Issues
When testing code with large inputs, developers frequently face issues like:
- OutOfMemoryError: This error occurs when the JVM attempts to allocate an object but runs out of memory.
- High Garbage Collection (GC) Overhead: Excessive CPU time spent on GC can significantly slow down an application.
- Memory Leaks: These occur when objects are held in memory unnecessarily, thereby consuming resources without being utilized.
Profiling Memory Usage
Before we dive into solutions, it's worthwhile to profile your application's memory usage. This can be done using tools like:
- VisualVM: A monitoring and performance tool for Java applications.
- YourKit: A commercial Java profiler for analyzing memory usage.
Using these tools, you can gain insights into memory consumption, identify memory leaks, and troubleshoot out-of-memory errors.
Strategies to Deal with Memory Issues
1. Optimize Data Structures
Selecting the right data structure is pivotal in saving memory. For example, if you are utilizing an ArrayList
, consider using an LinkedList
if you require frequent insertions.
List<Integer> list = new ArrayList<>(); // Memory inefficient for frequent inserts
list.add(1);
list.add(2);
// Alternative
List<Integer> linkedList = new LinkedList<>(); // More efficient for insertions
linkedList.add(1);
linkedList.add(2);
2. Use Primitive Data Types
When possible, prefer primitive data types over wrapper classes to save memory. For instance, using int
instead of Integer
reduces overhead.
int[] numbers = new int[1000000]; // Efficient
Integer[] numbersWrapper = new Integer[1000000]; // More overhead
3. Utilize Streaming
Java 8 introduced the Stream API, which processes large datasets in a memory-efficient manner. This avoids loading the entire dataset into memory all at once.
List<Integer> largeList = IntStream.rangeClosed(1, 1_000_000)
.boxed()
.collect(Collectors.toList());
// Use Stream API to process without holding all in memory
long countOfEvenNumbers = largeList.stream()
.filter(n -> n % 2 == 0)
.count();
4. Implement Lazy Loading
Lazy loading defers the initialization of an object until it is needed. This technique can be beneficial for large datasets that might not always be necessary.
class LazyLoader {
private List<String> data;
public List<String> getData() {
if (data == null) {
loadData();
}
return data;
}
private void loadData() {
this.data = new ArrayList<>();
// Load data from a database or a file
}
}
5. Increase Heap Size
In cases where the application legitimately requires more memory, consider increasing the heap size. Use the -Xms
and -Xmx
flags when launching your application.
java -Xms512m -Xmx2048m -jar myapp.jar
-Xms
specifies the initial heap size.-Xmx
specifies the maximum heap size.
6. Analyze Heap Dumps
If you encounter an OutOfMemoryError
, analyze the heap dump generated by the JVM. Use tools like Eclipse Memory Analyzer (MAT) to analyze and identify memory leaks or analyze memory allocation patterns.
7. Use Weak References
If certain objects can be recreated when needed, consider using WeakReferences, which allow for more flexible garbage collection.
WeakReference<MyObject> weakReference = new WeakReference<>(new MyObject());
MyObject obj = weakReference.get(); // Will return null if GC has cleared it
This allows the garbage collector to reclaim the memory when the reference is not strongly reachable.
8. Limit Input Size in Tests
In testing environments, particularly when using unit tests, be aware of input sizes. Use mocks or smaller subsets of larger datasets to avoid overwhelming the JVM.
9. Employ Pagination for Large Datasets
Implement pagination when dealing with vast amounts of data. This can dramatically reduce memory consumption and increase performance.
public List<String> getPagedData(int pageNumber, int pageSize) {
int offset = (pageNumber - 1) * pageSize;
return database.query("SELECT * FROM large_table LIMIT ? OFFSET ?", pageSize, offset);
}
Using pagination allows only a portion of the dataset to be loaded at a time.
My Closing Thoughts on the Matter
Handling memory issues when testing code that processes large inputs in Java may seem daunting, but with a thorough understanding of Java’s memory management, proper coding practices, and the right tools, it's entirely feasible. By optimizing data structures, implementing lazy loading, and profiling memory usage, you can keep your applications running smoothly.
For more information on Java memory management, check out the official Java Documentation.
By incorporating these strategies, your application won't just run efficiently, but also maintainability becomes easier as your project evolves.
Feel free to explore deeper insights on Java Memory Management and refine your understanding of how to tackle memory issues effectively in your Java applications!