Overcoming Complexity in Microservices with Immutable Pointers
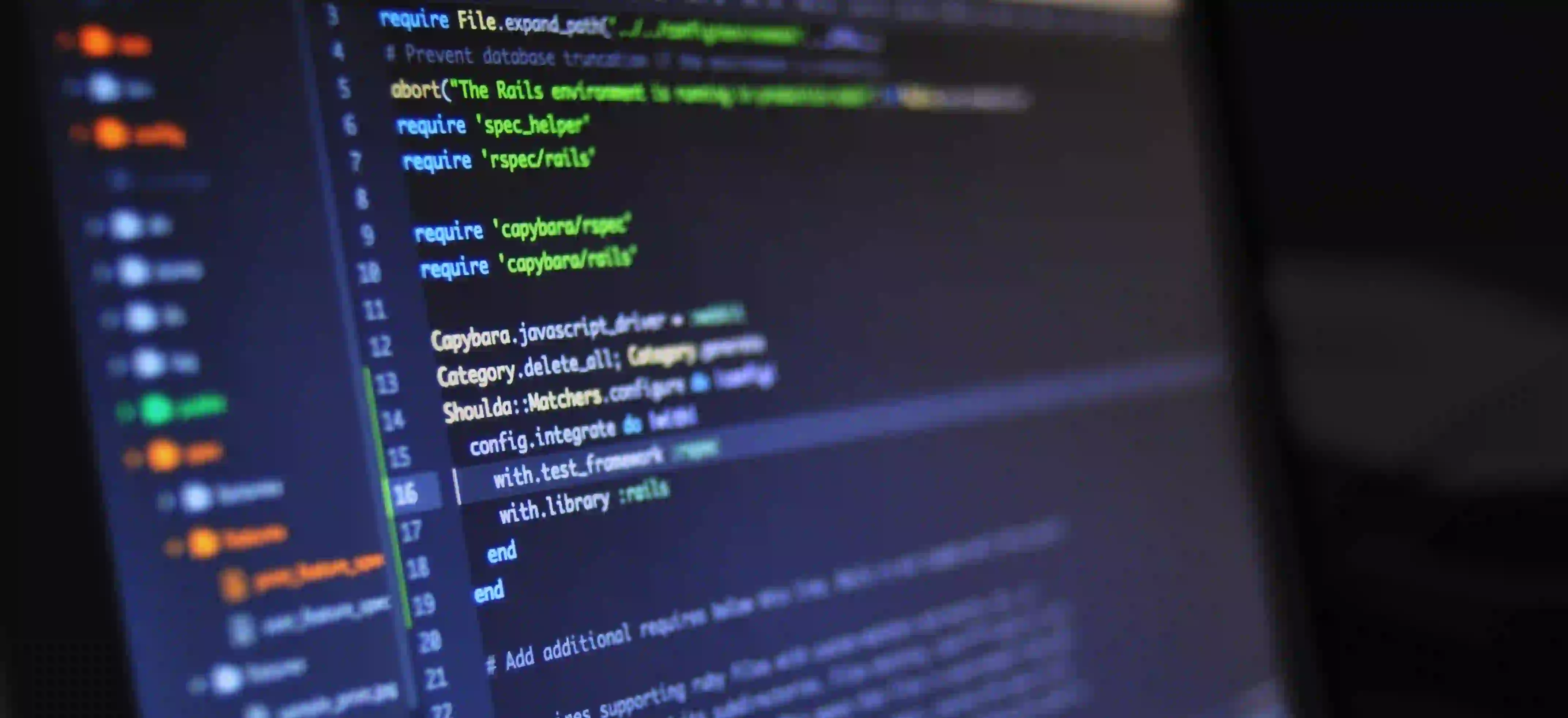
Overcoming Complexity in Microservices with Immutable Pointers
Microservices architecture offers a promising approach to developing scalable and maintainable software. Each service can be written in different languages, deployed independently, and scaled as needed. However, with the independence of services comes complexity, especially concerning state management and data consistency. In this blog post, we will discuss how immutable pointers can help in managing these complexities effectively.
Understanding Microservices
Microservices are small, independent, and loosely coupled services that communicate over a network. This structure promotes a single responsibility principle, allowing teams to work independently on different services. However, the common challenge these services face is data management. Services often need to share or exchange data, and ensuring consistent, thread-safe, and synchronized data between them can be challenging.
Problems of State and Data Management
-
Data Consistency: As independent services store their state, maintaining a consistent view of the data across services becomes a daunting task.
-
State Mutation: Mutable state can lead to unexpected side effects. One service's change can inadvertently affect another service, leading to hard-to-trace bugs.
-
Concurrency Issues: Microservices often face concurrent requests. Managing state without a solid concurrency control mechanism can lead to data corruption.
Introducing Immutable Pointers
Immutable pointers allow for a design where data once created cannot be modified. Instead, any changes create a new instance of the data. This paradigm shifts how we think about state management in microservices.
Advantages of Immutable Pointers
-
Predictability: Since data cannot change, the behavior of services becomes predictable. This is invaluable in a distributed system.
-
Easier Debugging: With immutable data structures, tracing issues becomes more manageable. If an error occurs, you can check previous states of the data without worrying about unintended mutations.
-
Concurrency-Friendly: Immutable structures help in managing concurrency issues. Since the state can't change, multiple threads can read the data without the need for locks.
-
Functional Programming Paradigm: Many developers are familiar with functional programming which emphasizes immutability. Using immutable pointers can align with these principles, making it easier for teams trained in both paradigms.
Implementing Immutable Pointers in Java
To illustrate how to incorporate immutable pointers into your microservices, we can use Java—a language well-suited for microservices due to its rich ecosystem and robust frameworks like Spring Boot.
Example of an Immutable Class
Let’s start with a simple Java example of an immutable class.
public final class Person {
private final String name;
private final int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
// Creating a new instance with modified age
public Person withAge(int newAge) {
return new Person(this.name, newAge);
}
}
Commentary on the Code
- The class
Person
is declared asfinal
to prevent subclassing. This ensures that its behavior remains unchanged. - Fields are
final
to ensure they are assigned only once. - The
withAge
method doesn't modify the existing instance; it creates and returns a new instance ofPerson
with the updated age. - This pattern promotes immutability and allows for functional-style programming, making the class easier to work with in a microservices context.
Using Immutable Collections in Java
Java also has collections that can be made immutable. The Java Collections Framework provides tools for creating immutable collections.
import java.util.Collections;
import java.util.List;
public class ImmutableExample {
private final List<String> items;
public ImmutableExample(List<String> items) {
this.items = Collections.unmodifiableList(items); // Wraps the list to prevent modification
}
public List<String> getItems() {
return items;
}
}
Why Use Immutable Collections?
Using immutable collections provides several benefits:
- Thread Safety: Immutable collections are inherently thread-safe. Data can be shared across services without needing to synchronize.
- No Side Effects: When a collection is used, there are no side effects, as the data cannot be modified from anywhere else.
Challenges and Considerations
While immutable pointers provide many advantages, they are not without challenges:
-
Memory Usage: Immutable objects can lead to increased memory usage, as every modification creates a new object. However, the benefits often outweigh these costs.
-
Learning Curve: Developers accustomed to mutable states may find transitioning to an immutable paradigm challenging.
-
Performance Concerns: In highly dynamic systems, constantly creating new instances can lead to performance slowdowns. Profiling and testing in your specific context are critical.
Wrapping Up
Incorporating immutable pointers into your microservices architecture can help mitigate the complexities of state management and data consistency. By promoting predictability, improving debugging capabilities, and enhancing concurrency handling, immutable pointers offer a robust solution in a microservices paradigm.
For further reading on microservices design patterns and best practices, consider exploring Martin Fowler's Patterns of Enterprise Application Architecture and Microservices.io.
The shift towards functional programming paradigms, particularly using immutable data structures, is gaining traction for a reason. As microservices continue to dominate modern software architecture, leveraging immutable pointers could be a step towards more resilient and maintainable systems.
Engaging with these concepts not only equips developers with the necessary tools to tackle complexity but also paves the way for scalable and efficient software development. Start implementing immutable pointers in your services today and see the difference it makes.