Resolving Join Table Alias Issues in Hibernate SQLRestrictions
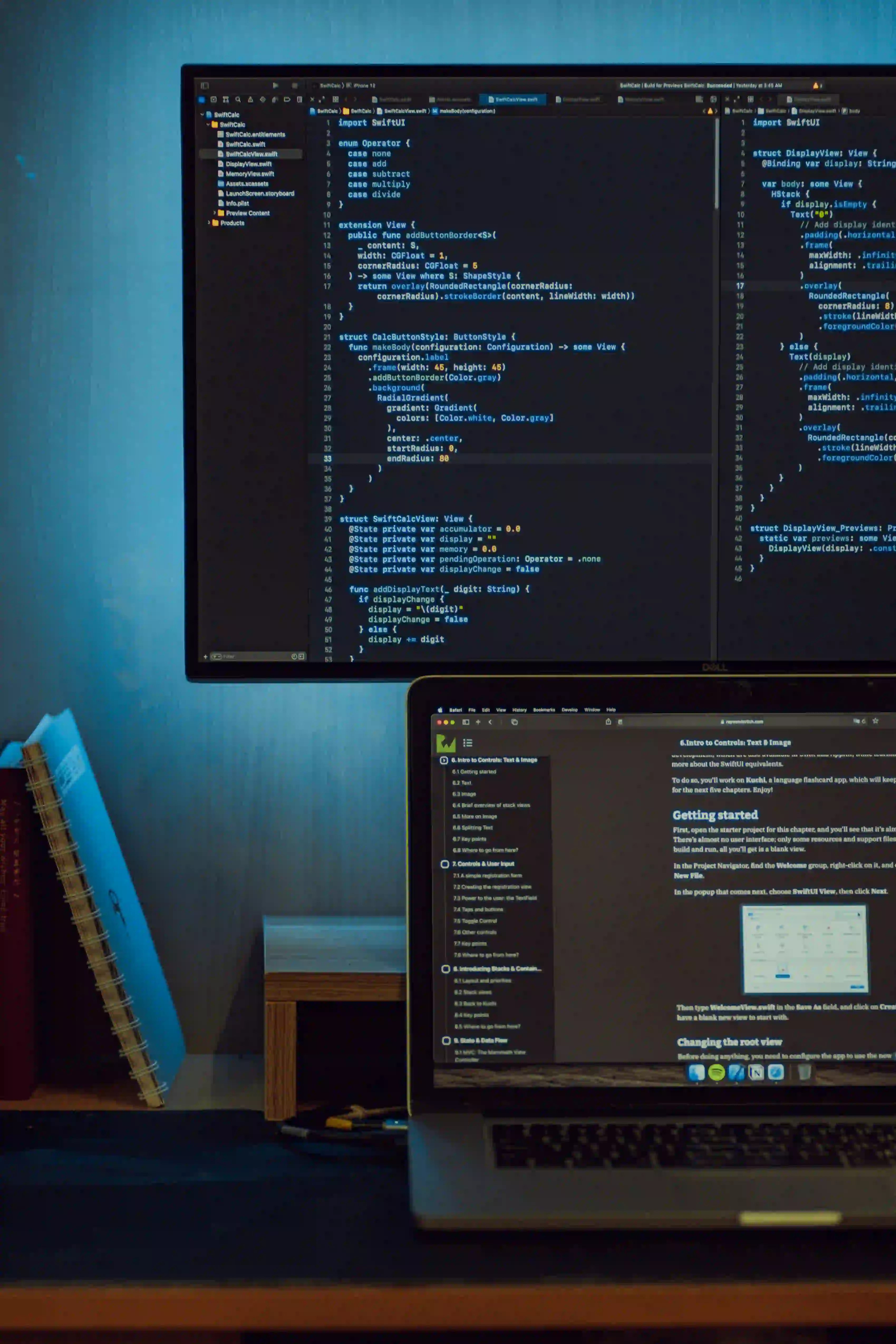
Resolving Join Table Alias Issues in Hibernate SQLRestrictions
Hibernate is a powerful Object-Relational Mapping (ORM) tool that simplifies database interactions in Java applications. However, it can sometimes get tricky, especially when it comes to using SQL restrictions with joined tables. This blog post explores common issues related to join table aliases in Hibernate SQL restrictions and how to resolve them effectively.
What Are SQLRestrictions?
SQLRestrictions in Hibernate allow you to specify criteria for queries. They are particularly useful for filtering data using raw SQL expressions. However, using SQLRestrictions with joins can be confusing due to aliasing. Understanding how aliases work and how to effectively use them is essential for successful querying.
Understanding Aliases in Joins
In SQL, when you join tables, you often use aliases to make your queries cleaner and to prevent ambiguity. For instance, consider this simple join between two tables: Authors
and Books
.
SELECT a.name, b.title
FROM Authors a
JOIN Books b ON a.id = b.author_id
In this query, a
and b
are aliases for the Authors
and Books
tables, respectively. This approach enhances readability and avoids collisions, particularly in complex queries.
In Hibernate, you might encounter issues when trying to apply SQLRestrictions due to how the framework interprets these aliases.
Common Issues with Join Table Aliases
1. Misaligned Aliases
One of the most prevalent issues is misaligning the alias defined in the Hibernate query and the one used in the SQLRestrictions. If you specify a join with an alias but refer to it incorrectly, Hibernate may throw an exception or return unexpected results.
2. Lack of Alias in SQLRestrictions
When SQLRestrictions are applied, if the condition references the main entity without specifying the join alias, you can end up with ambiguous queries. This can lead to performance issues or even runtime errors.
3. Complex Queries with Multiple Joins
As the number of joins increases, so does the complexity of managing aliases. Keeping track of which alias belongs to which table becomes a daunting task.
Resolving Alias Issues in Hibernate
Example Scenario
Assume we have two entities: Author
and Book
. The Book
entity has a many-to-one relationship with the Author
. We need to fetch books authored by a specific author with the last name "Smith".
Setting Up the Entities
First, let's set up our Hibernate entity classes.
@Entity
@Table(name = "authors")
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String firstName;
private String lastName;
// Getters and Setters
}
@Entity
@Table(name = "books")
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "author_id")
private Author author;
// Getters and Setters
}
Creating a Query with SQLRestrictions
Here’s an example of how to create a query using SQLRestrictions, where we specify the join alias correctly.
import org.hibernate.Criteria;
import org.hibernate.Session;
import org.hibernate.criterion.Restrictions;
// Assuming session is already created
Session session = HibernateUtil.getSessionFactory().openSession();
Criteria criteria = session.createCriteria(Book.class, "b")
.createAlias("b.author", "a")
.add(Restrictions.eq("a.lastName", "Smith"));
List<Book> booksBySmith = criteria.list();
Explanation of the Code
-
Setting up a Criteria Object: We create a
Criteria
object for theBook
entity, using "b" as an alias. -
Creating an Alias for Author: The
createAlias
method allows us to define an alias for theAuthor
entity. We use "a" for the author alias, which illustrates how linkage works within Hibernate. -
Using SQLRestrictions: The
add
method adds the SQL restriction, where we specify the last name condition with the defined alias.
Avoiding Common Pitfalls
-
Always Use Aliases: When dealing with join entities, always reference them with their respective aliases.
-
Check for Typos: Ensure that there are no typos in the alias names used in your SQLRestrictions.
-
Use Logging: Enable SQL logging for Hibernate to see the actual queries being generated. This helps in diagnosing issues related to aliases.
Advanced Example with Multiple Joins
If your application requires fetching data from more complex structures, e.g., a many-to-many relationship with an additional criteria, you might encounter even more complexity.
Here’s how to handle it:
Criteria criteria = session.createCriteria(Book.class, "b")
.createAlias("b.author", "a")
.createAlias("b.genre", "g") // assuming we added genre relationship
.add(Restrictions.eq("a.lastName", "Smith"))
.add(Restrictions.eq("g.name", "Fiction")); // Assuming genre name is a string
List<Book> fictionBooksBySmith = criteria.list();
Code Explanation
In this scenario:
- We create another alias for the
Genre
entity with "g". - Additional restrictions are applied to filter results based on multiple conditions.
Closing Remarks
Resolving join table alias issues in Hibernate SQLRestrictions doesn't have to be a daunting task. By keeping track of your aliases, ensuring they are consistently used across your queries, and following best practices, you can leverage Hibernate’s powerful querying capabilities effectively.
For more insights on Hibernate, you can check out Hibernate Documentation.
By following these guidelines, you can overcome the common pitfalls and ensure that your database interactions are both efficient and error-free, allowing you to focus more on business logic rather than query intricacies.
Feel free to leave comments below to share your experiences or any puzzles you have faced with Hibernate!