InputStream vs InputStreamReader: When to Use Each?
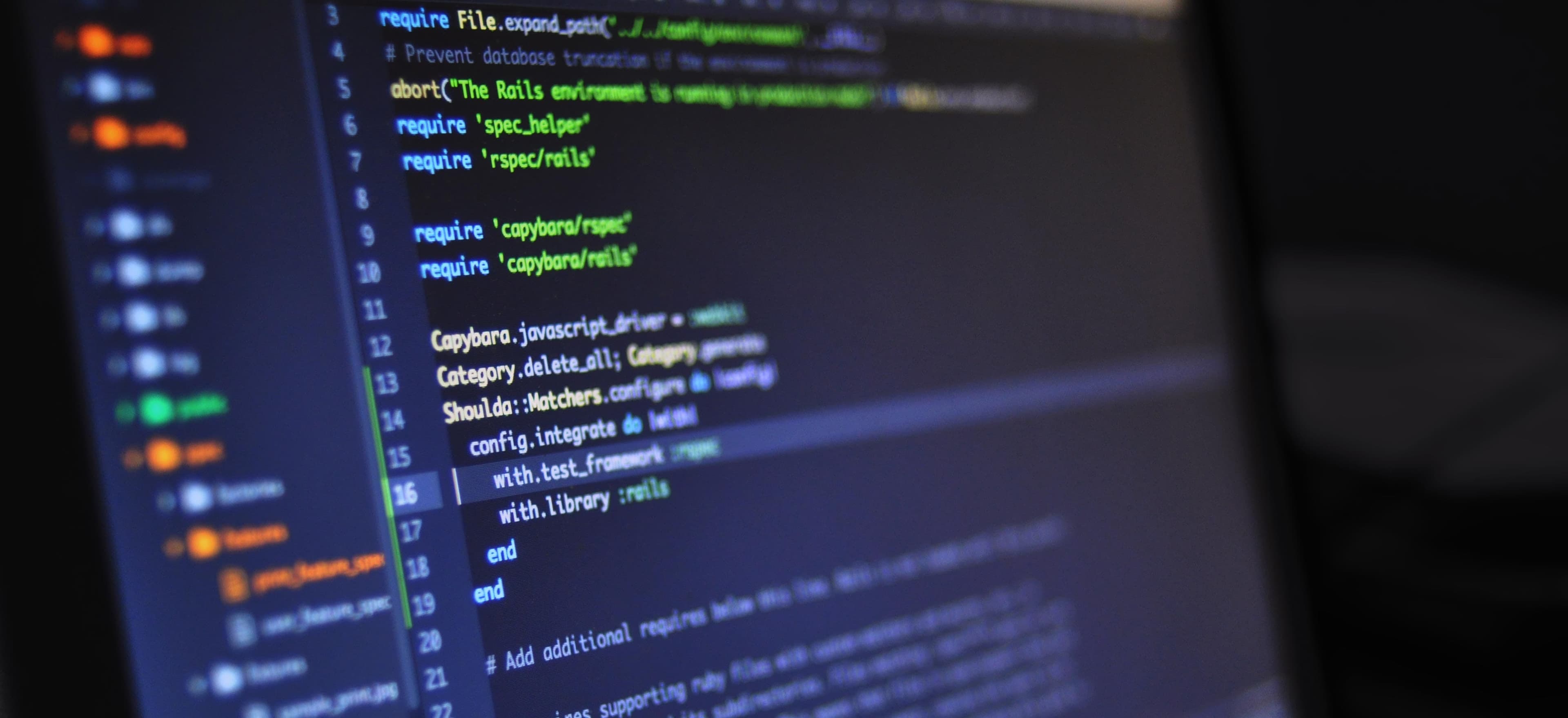
- Published on
InputStream vs InputStreamReader: When to Use Each?
In the world of Java programming, handling input data is an essential skill. Two fundamental classes that often come into play are InputStream
and InputStreamReader
. Understanding the distinction between these classes and when to utilize each can significantly impact the efficiency and effectiveness of your code. In this blog post, we’ll dive deep into both classes, their uses, and real-world implementations, while giving you the insights you need to make informed decisions.
Understanding InputStream
InputStream
is an abstract class that serves as the foundation for handling byte streams in Java. This class provides a way to read bytes from a source, which can be a file, network connection, or any other input source.
Key Characteristics of InputStream
- Byte-Oriented: The primary purpose of
InputStream
is to read raw byte data. This makes it ideal for binary data but less suitable for text. - Abstract Class: Being abstract means it cannot be instantiated directly. Subclasses like
FileInputStream
,ByteArrayInputStream
, etc., provide implementations for specific data sources. - Efficiency: It provides efficient methods for reading bytes and can handle large amounts of data without significant memory overhead.
Example Use Case of InputStream
import java.io.FileInputStream;
import java.io.IOException;
public class InputStreamExample {
public static void main(String[] args) {
FileInputStream fileInputStream = null;
try {
// Open a FileInputStream to read the specified file
fileInputStream = new FileInputStream("exampleFile.txt");
int byteData;
// Read byte by byte until the end of the stream
while ((byteData = fileInputStream.read()) != -1) {
// Process the byte data (for example, print it)
System.out.print((char) byteData);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
// Always close the InputStream to free resources
if (fileInputStream != null) {
try {
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
Commentary on InputStream Example
In the above example, we open a FileInputStream
to read a text file byte by byte. While this method can read text, it's crucial to remember that it reads data as bytes, which can be inefficient and problematic for text data that requires encoding.
Enter InputStreamReader
InputStreamReader
, on the other hand, is a bridge from byte streams to character streams. It can be used to read bytes and decode them into characters using a specified character set.
Key Characteristics of InputStreamReader
- Character-Oriented: Unlike
InputStream
,InputStreamReader
is designed for reading text data, making it the go-to option for processing strings. - Decoding Capabilities: It translates bytes into characters, respecting character encodings (like UTF-8, ISO-8859-1, etc.).
- Ease of Use: It simplifies the reading of character data from various byte streams.
Example Use Case of InputStreamReader
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
public class InputStreamReaderExample {
public static void main(String[] args) {
FileInputStream fileInputStream = null;
InputStreamReader inputStreamReader = null;
try {
// Instantiate a FileInputStream to read a file
fileInputStream = new FileInputStream("exampleFile.txt");
// Use InputStreamReader to convert byte stream to character stream
inputStreamReader = new InputStreamReader(fileInputStream, "UTF-8");
int charData;
// Read character by character until the end of the stream
while ((charData = inputStreamReader.read()) != -1) {
// Process the character data (for example, print it)
System.out.print((char) charData);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
// Close the readers in reverse order of their opening
try {
if (inputStreamReader != null) {
inputStreamReader.close();
}
if (fileInputStream != null) {
fileInputStream.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Commentary on InputStreamReader Example
In this example, we read from a text file using InputStreamReader
. By specifying "UTF-8" as the character encoding, the reader accurately converts byte sequences into the corresponding characters. This is especially essential when dealing with languages that utilize various character sets or encoding forms.
When to Use Each
Now that we’ve explored both InputStream
and InputStreamReader
, let's look at some guidelines to help you decide which one to use:
-
Use InputStream When:
- You need to handle raw byte data (like images, audio files, etc.).
- Performance is a critical factor and you are sure of the data type you are processing.
- There’s no need for any character encoding and you don’t care about text representation.
-
Use InputStreamReader When:
- You are dealing with text data that must be processed as characters.
- Proper character encoding is necessary to preserve the integrity of your text data.
- You want to take advantage of Java's character stream capabilities, like reading lines of text more easily.
Converting Between InputStream and InputStreamReader
There are scenarios when you may need to convert from an InputStream
to an InputStreamReader
, or vice versa, perhaps when the type of data changes during processing. Below is a short example demonstrating this conversion.
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.charset.StandardCharsets;
public class ConversionExample {
public static void main(String[] args) {
String data = "Hello, World!";
// Convert the string to a byte array
byte[] byteArray = data.getBytes(StandardCharsets.UTF_8);
// Create an InputStream from the byte array
ByteArrayInputStream byteArrayInputStream = new ByteArrayInputStream(byteArray);
try (InputStreamReader reader = new InputStreamReader(byteArrayInputStream, StandardCharsets.UTF_8)) {
int charData;
while ((charData = reader.read()) != -1) {
System.out.print((char) charData); // Reconstruct the original string
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Key Takeaways
- Performance & Type Handling: Choose
InputStream
for byte-level input andInputStreamReader
for character-level input. - Encoding Matters: The use of
InputStreamReader
is vital when working with textual data that contains special characters or when the encoding must be explicitly defined.
Lessons Learned
Understanding and choosing between InputStream
and InputStreamReader
is pivotal in Java programming. While InputStream
provides a foundation to handle raw byte data, InputStreamReader
extends those capabilities to read text correctly, taking encoding into account. Your choice hinges upon the type of data you are processing. By selecting the appropriate class, you can significantly enhance the robustness and reliability of your Java applications.
For further reading, check out the official Java InputStream Documentation and Java InputStreamReader Documentation. Happy coding!
Checkout our other articles