Mastering JUnit: Handling Static Method Exceptions with Ease
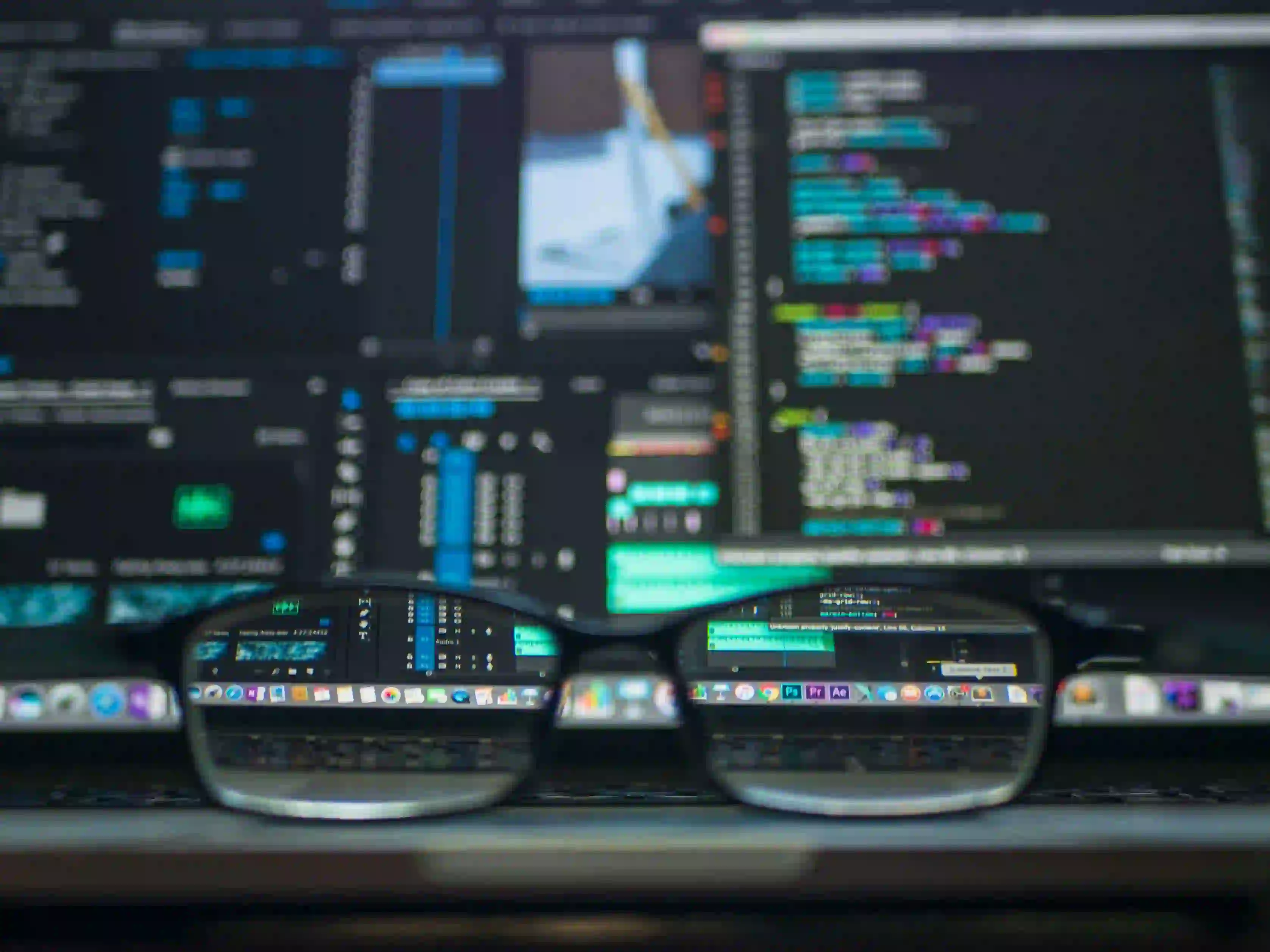
Mastering JUnit: Handling Static Method Exceptions with Ease
When it comes to testing in Java, JUnit is the most widely used framework. It allows developers to write and run tests effectively. One of the common challenges developers face is dealing with exceptions thrown by static methods during unit tests. This blog post will guide you through the various ways to handle static method exceptions in JUnit, ensuring that your tests are robust and maintainable.
Understanding JUnit Basics
Before we dive into exceptions handling, let's cover some basics of JUnit. JUnit is a simple framework to write repeatable tests. It is an instance of the xUnit architecture for unit testing frameworks. The main annotations you will encounter in JUnit include:
@Test
: Marks a method as a test method.@Before
: Runs before each test method.@After
: Runs after each test method.@BeforeClass
: Runs once before any of the test methods in the current class.@AfterClass
: Runs once after all the tests in the current class have run.
Being familiar with these annotations is crucial for effective testing.
Dealing with Static Methods in Java
Static methods pose unique challenges during unit testing. They cannot be mocked easily using traditional mocking frameworks like Mockito. However, understanding how to handle exceptions thrown by these methods can set the foundation for writing robust tests.
Example Scenario
Let's say we have a utility class MathUtils
with a static method divide
:
public class MathUtils {
public static int divide(int numerator, int denominator) {
if (denominator == 0) {
throw new IllegalArgumentException("Denominator cannot be zero.");
}
return numerator / denominator;
}
}
In this method, an IllegalArgumentException
is thrown when the denominator
is zero. Now, let’s write a JUnit test to handle this scenario.
Writing the JUnit Test
Test Code Snippet
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class MathUtilsTest {
@Test
public void testDivide_ThrowsIllegalArgumentException_WhenDenominatorIsZero() {
IllegalArgumentException exception = assertThrows(IllegalArgumentException.class, () -> {
MathUtils.divide(10, 0);
});
assertEquals("Denominator cannot be zero.", exception.getMessage());
}
}
Commentary
-
assertThrows: This method is vital as it verifies that a particular piece of code (in this case,
MathUtils.divide(10, 0)
) throws the expected exception. If the exception is thrown, it returns the exception; if not, the test fails. -
Message Verification: After ensuring the exception is thrown, we verify the exception message with
assertEquals
. This adds an additional layer of verification to our test, ensuring that not only does the exception get thrown, but it also conveys the correct message.
More Complex Scenarios
What if you want your tests to handle multiple exceptions or encapsulate more complex logic? Let's look at a variation that adds more scenarios.
Multiple Exception Handling Test Code Snippet
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class MathUtilsTest {
@Test
public void testDivide() {
// Testing valid division
assertEquals(2, MathUtils.divide(10, 5));
// Testing division by zero
IllegalArgumentException exception = assertThrows(IllegalArgumentException.class, () -> {
MathUtils.divide(10, 0);
});
assertEquals("Denominator cannot be zero.", exception.getMessage());
// Testing negative numerator
assertEquals(-2, MathUtils.divide(-10, 5));
// Testing negative denominator
assertEquals(-2, MathUtils.divide(10, -5));
// Testing both negative
assertEquals(2, MathUtils.divide(-10, -5));
}
}
Commentary
The testDivide
method in this instance:
- Tests valid division scenarios alongside zero division handling.
- Includes edge cases with negative numbers, which is often necessary depending on the logic of your application.
- Promotes thoroughness across various situations that could arise during runtime.
Benefits of Exception Handling in JUnit Tests
- Improved Code Quality: Properly handling exceptions leads to more reliable and secure code, as unintended crashes can be caught during testing.
- Enhanced Readability: Tests that clearly specify what exceptions are expected enhance maintainability and readability.
- Better Debugging: When tests fail, knowing the expected exceptions can help pinpoint the cause more swiftly.
Bringing It All Together
Mastering exception handling in JUnit, especially around static methods, is essential for building resilient applications. By effectively testing and handling exceptions, you ensure that your code behaves as expected even under adverse conditions.
To further enhance your understanding, consider exploring the following:
The world of testing is vast and often daunting, but by focusing on key techniques like those discussed here, you can enhance your skills. Happy testing!