Top 5 Security Risks in RESTful Web Services
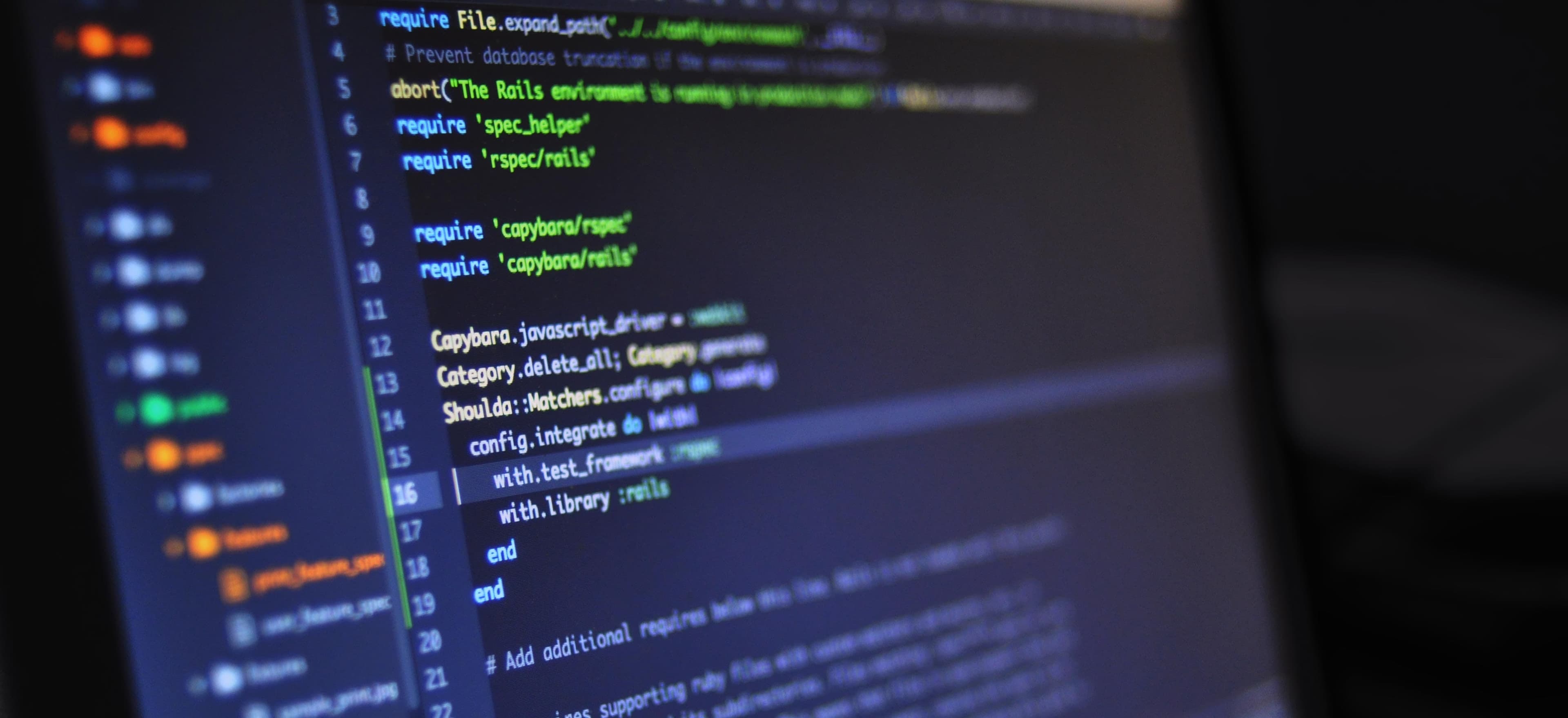
- Published on
Top 5 Security Risks in RESTful Web Services
In the modern age of interconnected applications and services, RESTful web services have become a cornerstone for building scalable and efficient web applications. However, with their high accessibility, they also introduce various security risks. Understanding these vulnerabilities is critical for developers.
In this blog post, we will discuss the top five security risks associated with RESTful web services, practical code snippets, and protective measures you can implement to mitigate these risks.
1. Injection Attacks
One of the most prevalent security risks is injection attacks, where an attacker injects malicious code into a web service. This could include SQL injection, XML injection, and more.
Example: SQL Injection
When user inputs are concatenated directly in SQL statements without proper validation, an attacker can manipulate these inputs to execute arbitrary SQL commands.
String userId = request.getParameter("userId");
String query = "SELECT * FROM users WHERE id = '" + userId + "'";
Why This is Dangerous:
The above code is vulnerable because it directly includes userId
input into the SQL query.
Prevention:
To guard against SQL injection, use prepared statements.
PreparedStatement stmt = connection.prepareStatement("SELECT * FROM users WHERE id = ?");
stmt.setString(1, userId);
ResultSet rs = stmt.executeQuery();
By using prepared statements, the database separates the query from the data, significantly reducing the risk of SQL injection.
2. Insufficient Authentication and Authorization
When RESTful services do not implement proper authentication and authorization, unauthorized users can access sensitive endpoints.
Example: Lack of Token Verification
If your application allows access to certain endpoints without verifying a user's token, then anyone can exploit this loophole.
@RequestMapping("/api/data")
public ResponseEntity<Data> getData() {
// No token validation
return ResponseEntity.ok(dataService.getSensitiveData());
}
Why This is Dangerous: In the example above, any user can access sensitive data without having valid credentials.
Prevention:
Always implement authentication checks for sensitive endpoints.
@RequestMapping("/api/data")
public ResponseEntity<Data> getData(@RequestHeader("Authorization") String token) {
if (!isValidToken(token)) {
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).build();
}
return ResponseEntity.ok(dataService.getSensitiveData());
}
3. Sensitive Data Exposure
Failing to encrypt sensitive information can expose critical user data, including personal identifiable information (PII) and payment details.
Example: Unencrypted Data Transfer
If a RESTful service sends sensitive data without encryption, it can easily be intercepted.
@POST
@Path("/api/submit")
public Response submitData(@Body Data data) {
// Process data
return Response.ok().entity("Data Received").build();
}
Why This is Dangerous: Sending data in plain text can lead to data breaches if intercepted.
Prevention:
Use HTTPS to encrypt data transmitted over the network and apply encryption methods for sensitive data at rest.
// Ensure that your API is served over HTTPS
4. Cross-Site Request Forgery (CSRF)
CSRF is an attack that tricks a user into unintentionally submitting a request, usually by clicking a malicious link.
Example: CSRF Vulnerability
A RESTful service that relies solely on cookie-based authentication can fall victim to CSRF.
@POST
@Path("/api/logout")
public Response logout() {
// Log out user based on session cookie
return Response.ok().entity("Logged out").build();
}
Why This is Dangerous: If a malicious site can issue POST requests to this endpoint, it may log out users without their knowledge.
Prevention:
Utilize anti-CSRF tokens to ensure that requests are made intentionally by authenticated users.
// Include a CSRF token in request headers
5. Misconfigured Security Settings
Improperly configured security settings can drastically weaken the security of your RESTful services. For instance, exposing detailed error messages can provide attackers with useful hints.
Example: Exposing Stack Traces
When exceptions occur, if stack traces are returned to clients without validation, attackers can gain insights into your application.
@ExceptionHandler(Exception.class)
public ResponseEntity<Object> handleExceptions(Exception ex) {
return ResponseEntity.badRequest().body(ex.toString());
}
Why This is Dangerous: This can reveal backend implementation details that help attackers strategize their attacks.
Prevention:
Always show user-friendly error messages, and log full error details server-side.
@ExceptionHandler(Exception.class)
public ResponseEntity<Object> handleExceptions(Exception ex) {
logger.error("Error occurred: ", ex);
return ResponseEntity.badRequest().body("An error occurred, please try later.");
}
To Wrap Things Up
While RESTful web services offer substantial benefits, they also introduce several security risks. By understanding and mitigating these threats—such as injection attacks, inadequate authentication and authorization, sensitive data exposure, CSRF attacks, and misconfigured settings—you can build resilient web applications that prioritize security.
Don’t wait until a security incident occurs; proactively implement these best practices to safeguard your RESTful web services. For a deeper understanding, consider researching OWASP's Top Ten Web Application Security Risks and integrating their recommendations into your development processes.
This structured approach will help you navigate the intricacies of RESTful web service security, enabling you to deliver secure, reliable applications to your users.
Checkout our other articles