Mastering SummaryStatistics in JDK 8: Common Pitfalls
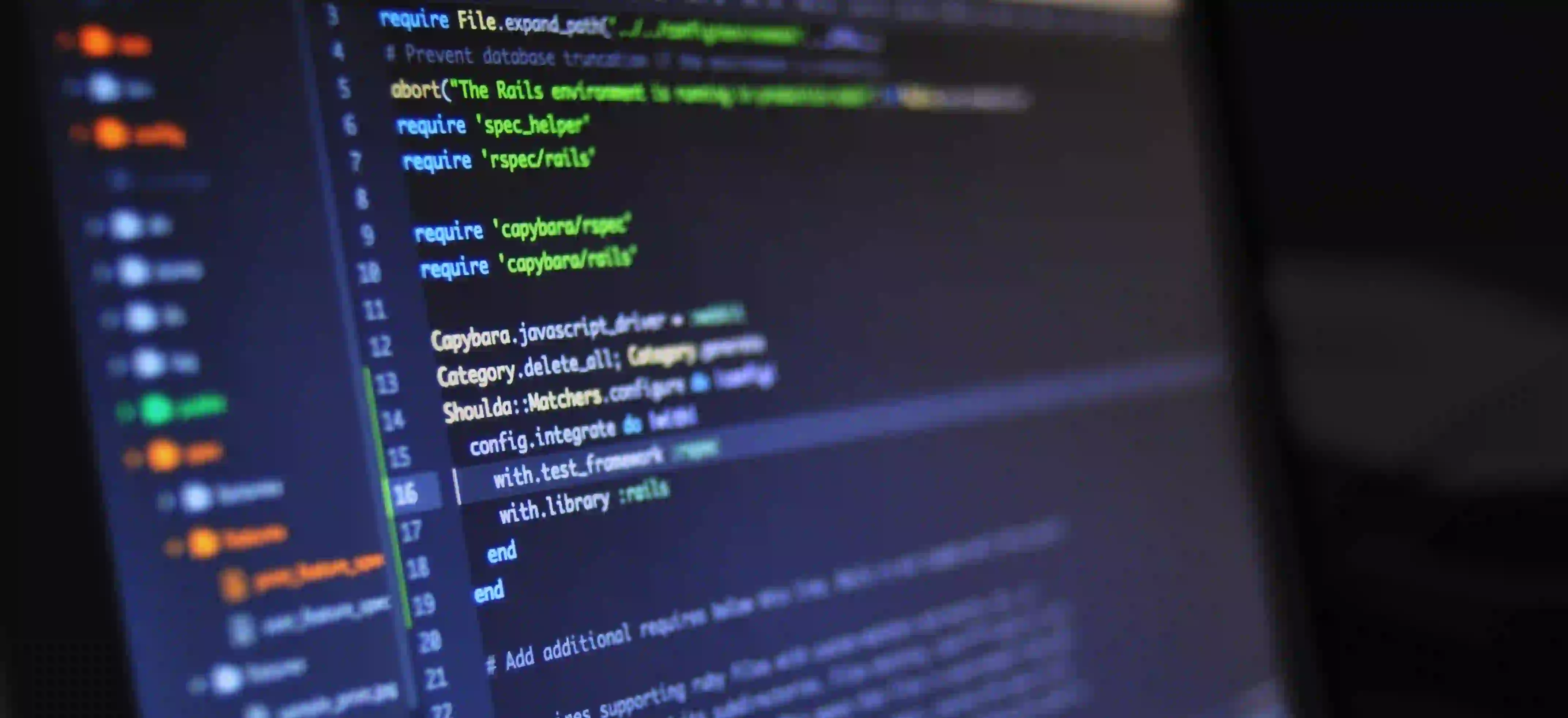
Mastering SummaryStatistics in JDK 8: Common Pitfalls
Java has long been a staple in the programming universe, delivering robust solutions across various domains. With the introduction of Java Development Kit (JDK) 8, the language brought in an array of new features, one of which is the SummaryStatistics
class. This class, positioned under the java.util
package, provides a straightforward mechanism to compute various summary statistics for a stream of numeric values efficiently. Whether you are handling large datasets or simply analyzing input from user applications, SummaryStatistics
can significantly ease your workload.
However, it’s not all sunshine and rainbows. Many Java developers, especially those new to JDK 8's stream API, often fall into common pitfalls when using SummaryStatistics
. This blog post will elucidate these pitfalls, enhance your understanding of the SummaryStatistics
class, and share solutions that can help you master this powerful feature.
What is SummaryStatistics?
Before diving into common pitfalls, let’s clarify what SummaryStatistics
is.
SummaryStatistics
is a utility class designed to hold statistics such as count, sum, min, average, and max values from a stream of numeric data. This is particularly helpful when working with numbers, as it saves you the overhead of manually calculating these metrics.
Here’s a code snippet demonstrating its basic use:
import java.util.IntSummaryStatistics;
import java.util.stream.IntStream;
public class SummaryStatisticsExample {
public static void main(String[] args) {
IntSummaryStatistics stats = IntStream.of(1, 2, 3, 4, 5)
.summaryStatistics();
System.out.println("Count: " + stats.getCount());
System.out.println("Sum: " + stats.getSum());
System.out.println("Min: " + stats.getMin());
System.out.println("Average: " + stats.getAverage());
System.out.println("Max: " + stats.getMax());
}
}
In this example, we are calculating the summary statistics for a stream of integers from 1 to 5. The IntSummaryStatistics
class takes care of the calculations in an efficient manner.
Common Pitfalls in Using SummaryStatistics
Despite its simplicity, developers may encounter some typical issues when using SummaryStatistics
. Below are some of these pitfalls along with explanations and recommended solutions.
Pitfall 1: Forgetting to Import the Right Class
One of the most straightforward mistakes is failing to import the correct SummaryStatistics
class. Java has several classes related to statistics, and it's easy to get mixed up.
Solution: Always ensure you're importing the right class based on your data type. For integer data, use IntSummaryStatistics
. For double values, opt for DoubleSummaryStatistics
.
Example for importing IntSummaryStatistics
:
import java.util.IntSummaryStatistics;
Pitfall 2: Using Streams Incorrectly
Another frequent issue arises when specifying streams in a way that isn't clear or efficient. For instance, some developers may try to accumulate values using additional methods after using summaryStatistics()
.
Solution: Use streams efficiently. You should collect your stream data before calculating summary statistics. Here’s an example of proper usage:
import java.util.DoubleSummaryStatistics;
import java.util.stream.DoubleStream;
public class DoubleSummaryExample {
public static void main(String[] args) {
DoubleSummaryStatistics stats = DoubleStream.of(1.5, 2.3, 3.8, 4.0)
.summaryStatistics();
System.out.println("Average: " + stats.getAverage());
// Continue with additional operations as needed
}
}
In this case, we are using DoubleStream
directly to work with double values, ensuring that we select the appropriate stream type.
Pitfall 3: Not Resetting Statistics
In scenarios where SummaryStatistics
calculations are repeated, it’s easy to forget that statistics accumulate over time. If you reuse an instance without resetting, you’ll get inaccurate results from previous calculations.
Solution: Always reset statistics when you want to start fresh with new data. You can use clear()
method to clear the statistics:
stats.clear(); // Resets the statistics
Pitfall 4: Neglecting Edge Cases
We often focus on the data we expect but can overlook edge cases. For example, what happens when no values are fed into SummaryStatistics
?
Solution: Always check statistics before utilizing them. Calling methods like getMin()
or getMax()
on an empty summary may lead to unexpected results or exceptions.
if (stats.getCount() > 0) {
System.out.println("Min: " + stats.getMin());
} else {
System.out.println("No data available.");
}
Pitfall 5: Misunderstanding the getAverage()
Method
The getAverage()
method can be misleading. It returns 0.0
if no values have been collected, but it does not throw an exception. Therefore, developers may incorrectly assume that an average of 0.0
is a valid average for existing data.
Solution: Always check if any values were collected before making assumptions based on the average:
if (stats.getCount() > 0) {
System.out.println("Average: " + stats.getAverage());
} else {
System.out.println("Average cannot be calculated without data.");
}
Pitfall 6: Ignoring Performance Testing
Similar to when using collections or algorithms, SummaryStatistics
can also lead to performance concerns, especially when handling large data sets. Developers sometimes neglect to benchmark their implementation.
Solution: Always conduct some metrics or performance testing to ensure that your use-case is optimized. The Stream
API should help, but it doesn’t mean that you shouldn’t measure.
In Conclusion, Here is What Matters
Summary statistics represent a powerful addition to the Java Development Kit with significant potential for optimizing data computation. However, as we've seen, common pitfalls abound for both novice and experienced developers. By being aware of these pitfalls and utilizing the tips provided, you can leverage SummaryStatistics
to its fullest, ensuring accurate and efficient computations in your applications.
By incorporating only a few lines of code while simultaneously avoiding common errors, Java developers can effortlessly gather statistics from their data streams. This not only enhances productivity but also leads to writing cleaner and more efficient code.
For further reading on JDK 8 features, consider checking out the Java SE 8 Documentation or Baeldung's comprehensive guide on Java Streams.
Happy coding!