Top 7 Spring Challenges When Creating RESTful Services
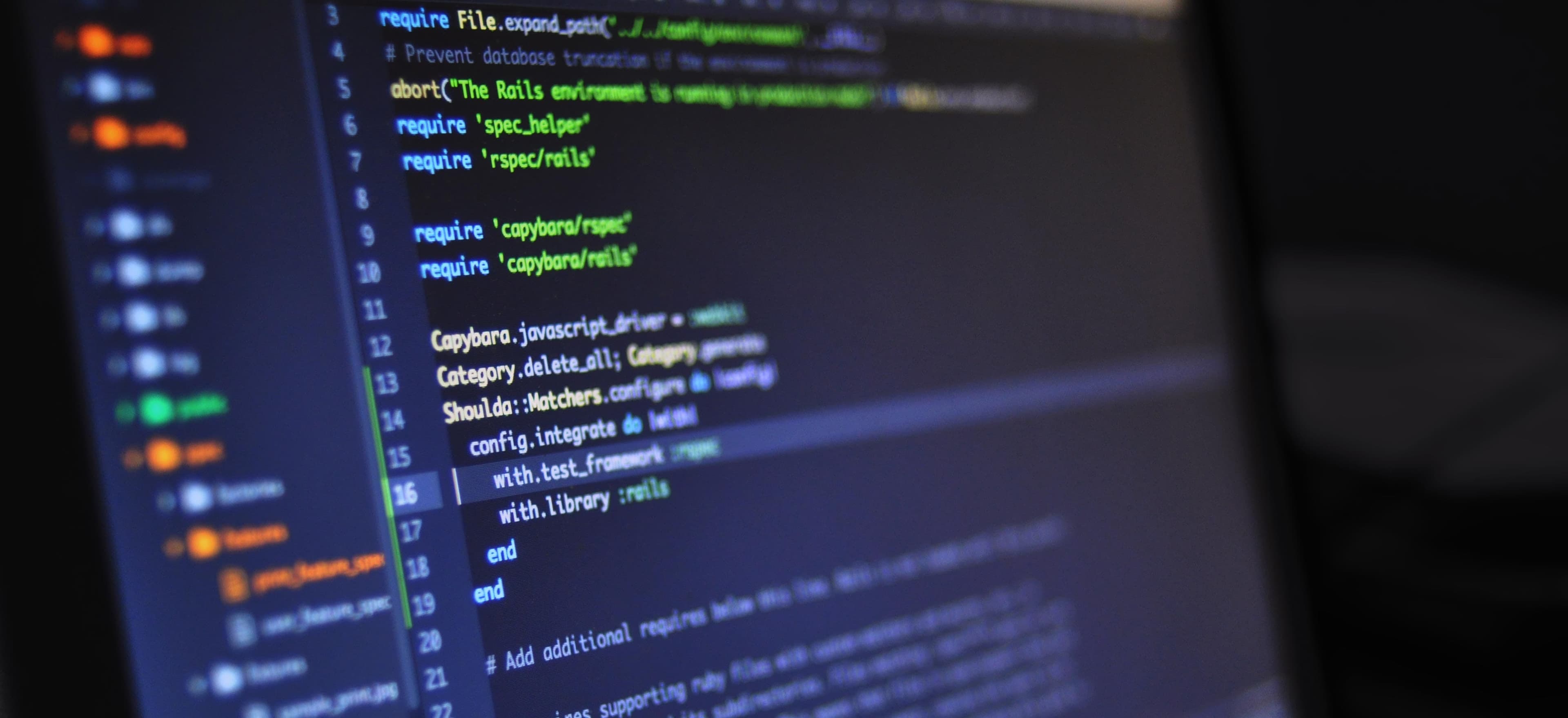
- Published on
Top 7 Spring Challenges When Creating RESTful Services
Creating RESTful services with Spring Framework can be a rewarding but challenging endeavor. With its robust features, Spring allows developers to create scalable and maintainable applications. However, along the way, there are common pitfalls and challenges that you might encounter. In this post, we will explore the top seven challenges when developing RESTful services in Spring, along with code snippets and detailed explanations.
Table of Contents
- Lack of Error Handling
- Managing Versioning
- Documentation and API Discovery
- Security Concerns
- Performance Optimization
- Proper Testing
- Handling CORS Issues
1. Lack of Error Handling
A common oversight in RESTful service creation is the lack of proper error handling. When an application runs into issues, it is essential to provide meaningful error responses to help clients diagnose problems effectively.
Solution
Spring provides a convenient way to handle exceptions globally using @ControllerAdvice
. Here's an example:
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<String> handleResourceNotFoundException(ResourceNotFoundException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleGeneralException(Exception ex) {
return new ResponseEntity<>("An unexpected error occurred", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Why This Matters
Using a global exception handler simplifies endpoint code, centralizes error handling, and makes it easier to debug issues.
2. Managing Versioning
As requirements evolve, API versioning becomes necessary to maintain backward compatibility. A poorly managed versioning strategy can lead to confusion for consumers of your API.
Solution
Versioning can be done in several ways: through URL path, request parameters, or request headers. The path-based versioning is most commonly used. Here’s how you can achieve it:
@RestController
@RequestMapping("/api/v1/products")
public class ProductController {
@GetMapping
public List<Product> getAllProducts() {
// return a list of products
}
}
To version up to v2, simply create another controller:
@RequestMapping("/api/v2/products")
public class ProductV2Controller {
@GetMapping
public List<ProductV2> getAllProducts() {
// return a list of version 2 products
}
}
Why This Matters
Versioning ensures your API can grow while still catering to existing clients, allowing for a smooth transition over time.
3. Documentation and API Discovery
Documentation is critical to ensure users of your API understand how to interact with it. Without it, the usability of your RESTful service decreases dramatically.
Solution
Springfox is a popular library for generating Swagger documentation for your Spring REST APIs. Here’s how to get started:
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
Enable Swagger in your Spring configuration:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.example"))
.paths(PathSelectors.any())
.build();
}
}
After this setup, your API documentation will be available at /swagger-ui.html
.
Why This Matters
Proper documentation fosters user engagement and helps developers integrate your API seamlessly into their applications.
4. Security Concerns
Security is paramount in any application, especially when exposing endpoints through a RESTful API. Leaving APIs unprotected opens the door for unauthorized access.
Solution
Spring Security can help secure your application with several configurations. Here’s an example of securing your API using basic authentication:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
Why This Matters
Securing your API helps protect sensitive data and resources from malicious actors and ensures a safe experience for your users.
5. Performance Optimization
Performance issues can severely affect user experience. Understanding how to diagnose and optimize your application is crucial.
Solution
Monitoring and profiling tools like Spring Boot Actuator can help identify bottlenecks in your services. You can enable it through:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
You can monitor various metrics using endpoints like /actuator/metrics
.
Why This Matters
Performance tuning helps reduce the load times and enhances the overall stability of your application, leading to higher user satisfaction.
6. Proper Testing
Testing your API is essential to ensure it's working as expected. Neglecting testing can lead to undetected bugs and unpredictable behavior upon deployment.
Solution
Spring provides built-in support for testing RESTful APIs with MockMvc
. Here's an example of testing a simple endpoint:
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
@SpringBootTest
@AutoConfigureMockMvc
public class ProductControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testGetAllProducts() throws Exception {
mockMvc.perform(get("/api/v1/products"))
.andExpect(status().isOk());
}
}
Why This Matters
Testing verifies the functionality of your RESTful services, helps uncover edge cases, and builds confidence in the stability of your application.
7. Handling CORS Issues
Cross-Origin Resource Sharing (CORS) issues can block your API from being accessed by web applications running on different origins.
Solution
Spring makes it straightforward to configure CORS globally or on specific endpoints. Here’s how you can configure it globally:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**")
.allowedOrigins("http://localhost:3000")
.allowedMethods("GET", "POST", "PUT", "DELETE", "OPTIONS");
}
}
Why This Matters
Proper CORS configuration is critical for ensuring that your API can be accessed without issues across different domains, especially for web applications.
To Wrap Things Up
While creating RESTful services with Spring Framework can present various challenges, being aware of these common issues helps you approach the development process more effectively. Proper error handling, versioning, documentation, and security significantly influence the usability and longevity of your API. Incorporating these best practices will enhance not just your API’s quality but also the overall developer experience.
You can delve deeper into Spring Framework and RESTful service development through the official Spring documentation or explore community tutorials. Happy coding!
Checkout our other articles