Understanding Content-Type: Your Key to Effective Downloads
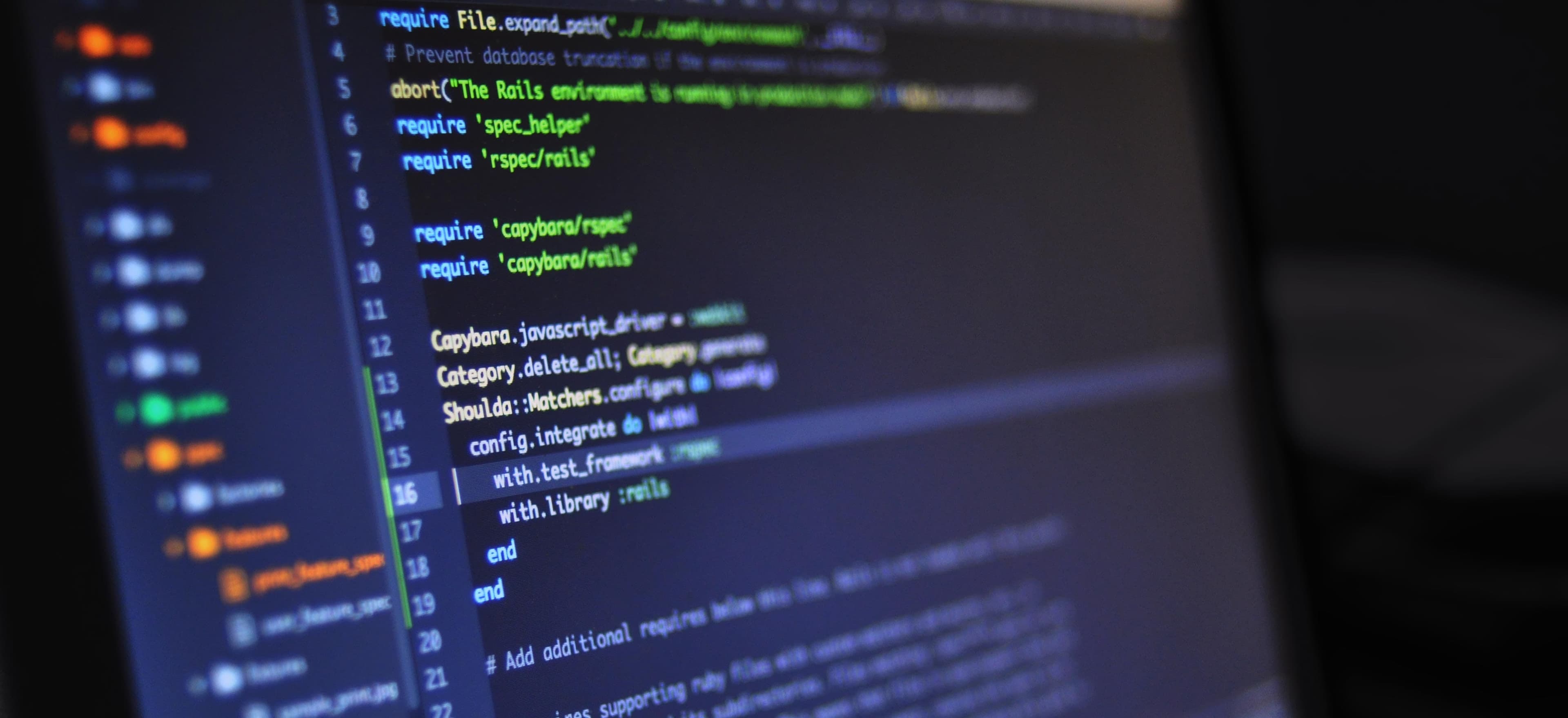
- Published on
Understanding Content-Type: Your Key to Effective Downloads
When dealing with web applications and APIs, understanding the Content-Type
header is crucial for ensuring that your files are delivered and handled correctly. This small but powerful HTTP header plays a significant role in the functioning of downloads, uploads, and content display. In this post, we will dive deep into the concept of Content-Type, how to effectively use it in your Java applications, and why it matters for file downloads.
What is Content-Type?
The Content-Type
header is part of the HTTP protocol. It indicates the media type of the resource being sent. For example, it tells the browser or client application how to handle the incoming data. The basic format of a Content-Type looks like this:
Content-Type: type/subtype
Some common examples include:
text/html
: for HTML documentsapplication/json
: for JSON dataimage/png
: for PNG imagesapplication/pdf
: for PDF documents
Understanding these types can significantly enhance user experience, particularly when downloading files.
Why is Content-Type Important for Downloads?
When a user attempts to download a file, the browser needs to know how to handle the file. Here are a few reasons why Content-Type is essential:
-
Determines File Handling: The browser decides whether to display or download the file based on its Content-Type.
-
Security Considerations: Browsers may block files if the Content-Type does not match the file's extension, helping to prevent potential security risks.
-
User Experience: Correctly setting the Content-Type ensures that the file is opened with the appropriate application, improving user satisfaction.
-
Interoperability: Different clients and browsers may handle files differently. Setting the correct Content-Type helps maintain consistency across various platforms.
How to Set Content-Type in Java
In Java, setting the Content-Type when serving files can be accomplished using the HttpServletResponse
class. Below are steps and code snippets to help you implement Content-Type effectively in your Java servlets.
Step 1: Create a Servlet
Here is a simple servlet that serves a PDF file:
import java.io.FileInputStream;
import java.io.IOException;
import java.io.OutputStream;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/download")
public class FileDownloadServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String filePath = "/path/to/document.pdf"; // Specify the file path
FileInputStream inputStream = new FileInputStream(filePath);
// Set content type
response.setContentType("application/pdf");
// Set content disposition for downloading
response.setHeader("Content-Disposition", "attachment; filename=\"document.pdf\"");
// Write the file to the response output stream
OutputStream outputStream = response.getOutputStream();
byte[] buffer = new byte[4096]; // Buffer size
int bytesRead;
// Read the file and write it to the output stream
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
inputStream.close();
outputStream.close();
}
}
Why Set Content-Type and Content-Disposition?
-
Content-Type: The application/pdf Content-Type informs the browser that it is receiving a PDF file, which helps it determine how to handle the download.
-
Content-Disposition: This header tells the browser that the content should be treated as an attachment, resulting in a download dialog rather than displaying the file.
Step 2: Compile and Deploy
Compile your servlet and ensure it is properly deployed in a servlet container (like Apache Tomcat). Once set up, you can access your servlet at the specified endpoint and test the file download functionality.
Handling Other File Types
You can apply the same principles to other file types. Here's a brief overview of how to handle some common file types:
1. Image Files
Serve a PNG image with the Content-Type image/png
:
response.setContentType("image/png");
response.setHeader("Content-Disposition", "inline; filename=\"image.png\"");
2. JSON Response
Return JSON data with the Content-Type application/json
:
response.setContentType("application/json");
response.getWriter().write("{\"key\": \"value\"}");
3. Excel Files
For Microsoft Excel sheets, use application/vnd.ms-excel
:
response.setContentType("application/vnd.ms-excel");
response.setHeader("Content-Disposition", "attachment; filename=\"data.xls\"");
Closing Remarks
Understanding and correctly implementing the Content-Type
header in your Java applications is critical for effective file downloads. It ensures that files are delivered correctly and enhances the overall user experience by providing appropriate file handling mechanisms.
Explore MDN's Content-Type documentation for a more thorough understanding of media types.
By keeping the Content-Type in mind, you can build robust applications that handle file downloads seamlessly, paving the way for a better user experience. Happy coding!
Checkout our other articles