Spring DI vs Dagger 2: Choosing the Right Tool for Java Apps
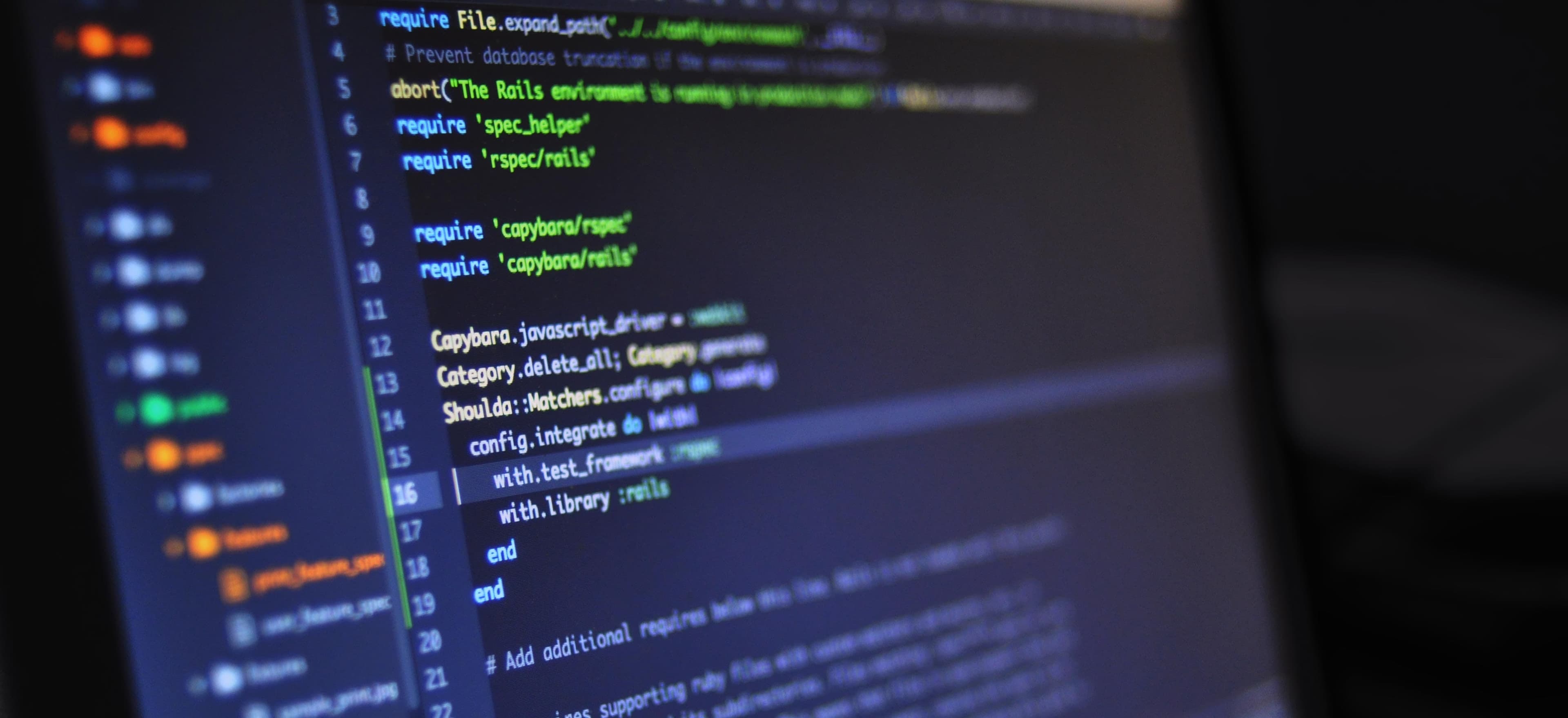
- Published on
Spring DI vs Dagger 2: Choosing the Right Tool for Java Apps
In the vast realm of Java development, Dependency Injection (DI) has emerged as a powerful pattern that promotes loose coupling, enhances testability, and encourages clean architecture. Among the most popular DI frameworks, Spring and Dagger 2 stand out. Each offers unique features tailored to specific use cases. This blog post will explore the strengths and weaknesses of both frameworks, helping you make an informed choice for your next Java application.
What is Dependency Injection?
Dependency Injection is a design pattern that allows a class to receive its dependencies from an external source rather than creating them itself. This separation of concerns leads to better maintainability and easier unit testing.
Benefits of Dependency Injection
- Loose Coupling: Classes are less dependent on concrete implementations.
- Easier Testing: Mock dependencies can be injected easily during testing.
- Configuration Flexibility: Change underlying implementations without affecting the class using them.
Before We Begin to Spring Framework
Spring is a comprehensive framework that provides a myriad of features beyond just DI—such as web applications, data access, and messaging. Its core DI container uses annotations and XML configuration, making it immensely versatile.
Basic Spring Dependency Injection Example
Here’s a basic example demonstrating how Spring handles DI.
// Service interface
public interface GreetingService {
String greet();
}
// Service implementation
public class GreetingServiceImpl implements GreetingService {
@Override
public String greet() {
return "Hello, World!";
}
}
// Spring Configuration
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class SpringConfig {
@Bean
public GreetingService greetingService() {
return new GreetingServiceImpl();
}
}
// Main Application
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(SpringConfig.class);
GreetingService service = context.getBean(GreetingService.class);
System.out.println(service.greet());
}
}
Commentary on Spring Example
- Easy Configuration: The
@Configuration
annotation allows you to define beans with simplicity. This streamlined approach is ideal for configuring complex applications without clutter. - Management of Application Context: Spring manages the lifecycle of beans, making it easy to handle dependencies, which reduces boilerplate code.
For more detailed guides on Spring DI, you can refer to Spring's Official Documentation.
Before We Begin to Dagger 2
Dagger 2 is a fast dependency injector for Java and Android specifically designed to be lightweight and efficient. Unlike Spring, Dagger 2 does not contain runtime reflection or code generation, which makes it an excellent choice for resource-constrained environments.
Basic Dagger 2 Dependency Injection Example
Here's an introductory example of how Dagger 2 works:
// Service interface
public interface GreetingService {
String greet();
}
// Service implementation
public class GreetingServiceImpl implements GreetingService {
@Override
public String greet() {
return "Hello from Dagger!";
}
}
// Module class to provide dependencies
import dagger.Module;
import dagger.Provides;
@Module
public class GreetingModule {
@Provides
GreetingService provideGreetingService() {
return new GreetingServiceImpl();
}
}
// Component interface to link module and consumer
import dagger.Component;
@Component(modules = GreetingModule.class)
public interface AppComponent {
GreetingService getGreetingService();
}
// Main Application
public class Main {
public static void main(String[] args) {
AppComponent appComponent = DaggerAppComponent.create();
GreetingService service = appComponent.getGreetingService();
System.out.println(service.greet());
}
}
Commentary on Dagger 2 Example
- Annotation-Based Configuration: Dagger uses annotations to define modules and components. This minimizes the boilerplate compared to traditional DI frameworks.
- Compile-Time Checking: Dagger performs dependency resolution at compile time, which reduces runtime errors and leads to potential performance benefits.
For more in-depth information about Dagger 2, consider exploring Dagger's Official Documentation.
Performance Considerations
When choosing between Spring and Dagger 2, performance can be a key factor:
- Spring is powerful and feature-rich, but can introduce overhead due to its extensive capabilities.
- Dagger 2, on the other hand, is designed for performance and does not require runtime reflection, making it especially suitable for Android apps.
Benchmarking Performance
Performance can vary widely based on your specific application needs. A typical approach is to measure the startup time and memory usage in both frameworks. Each project has its own requirements; thus, you should consider using tools such as JMH (Java Microbenchmark Harness) for precise benchmarking.
Use Cases
When to Use Spring
- Enterprise Applications: Spring's vast ecosystem offers solutions for every layer of your application.
- Microservices: Spring Boot offers quick microservice creation, allowing rapid development.
- Integration Needs: If you require integration with various databases, messaging systems, and cloud services, Spring provides built-in solutions.
When to Use Dagger 2
- Android Development: With its lightweight nature, Dagger is more suited for mobile environments.
- Performance-Critical Applications: If your application necessitates minimal overhead, Dagger 2 is a safer bet.
- Simple Dependency Needs: For smaller applications, Dagger’s simpler model can aid rapid development without the extras Spring provides.
In Conclusion, Here is What Matters
Both Spring and Dagger 2 have their respective strengths and are tailored for different scenarios. Spring is a comprehensive framework perfect for enterprise-level applications with extensive DI needs, whereas Dagger 2 shines in performance-critical and simpler use cases, especially for Android.
In the end, the best choice depends on your specific application requirements, team expertise, and future scalability needs. Whether you lean toward Spring or Dagger 2, mastering Dependency Injection is vital for any Java developer looking to write clean, maintainable, and testable code.
Further Reading
- Understanding Dependency Injection with Spring
- Getting Started with Dagger
Now that you have a clearer perspective on both tools, it’s time to dive into your next project with confidence. Happy coding!
Checkout our other articles