Troubleshooting Common Issues in Concurrent Application Testing
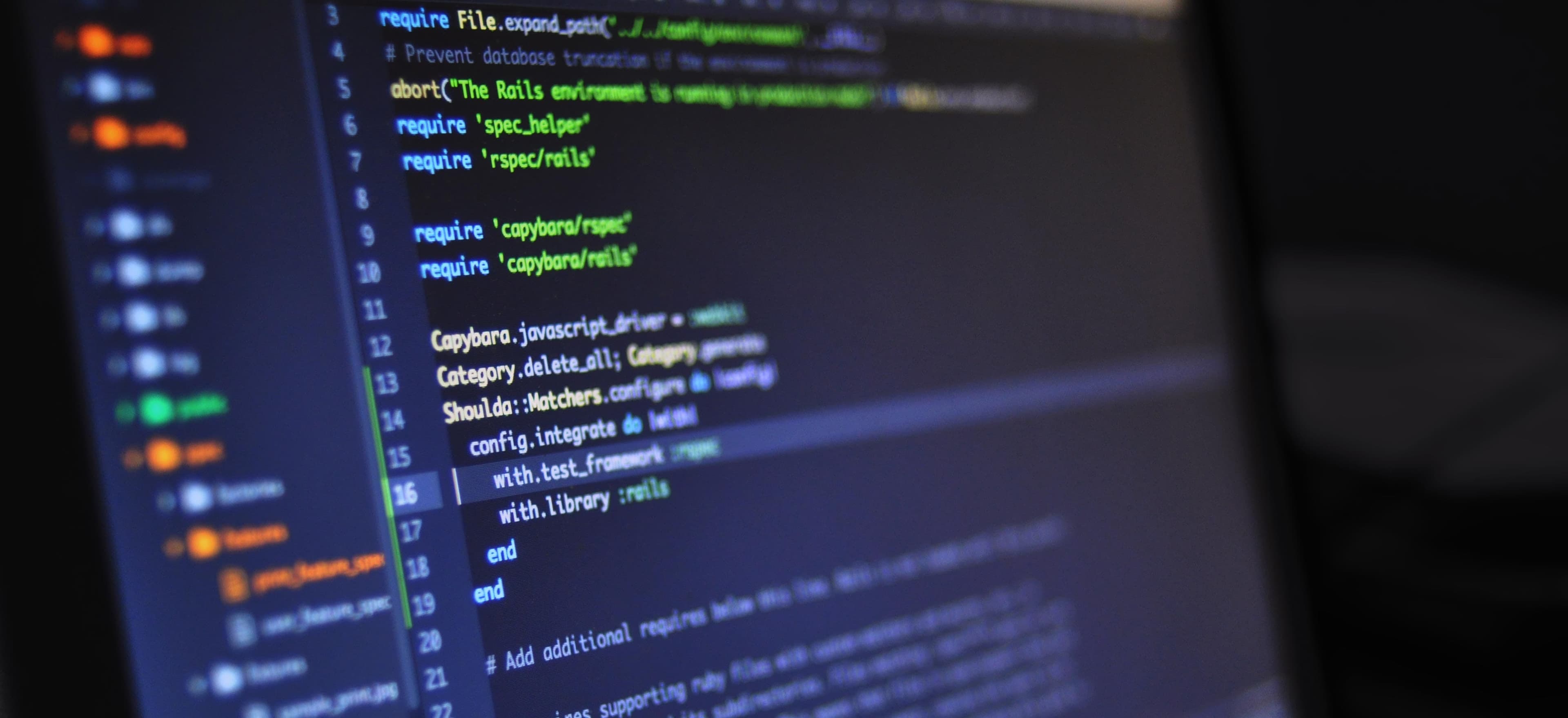
- Published on
Troubleshooting Common Issues in Concurrent Application Testing
In today's fast-paced world of web applications and interactive software, concurrency is paramount. It enables multiple processes to run at the same time, improving resource efficiency and user experience. However, it also brings a set of challenges, especially when it comes to testing. Concurrent application testing can lead to various issues that developers need to troubleshoot effectively. This blog post aims to highlight common problems encountered during concurrent application testing and provide actionable solutions.
Understanding Concurrency
Concurrency involves executing multiple tasks simultaneously. In programming, this can be achieved using threads, processes, or asynchronous programming. Java, a widely-used programming language, has robust support for concurrency through the Java Concurrency framework.
If your application uses threads, you should explore the Java Concurrency API. It provides a powerful abstraction for managing concurrency in Java applications, making it easier and safer.
Quick Example of Thread Creation
class MyRunnable implements Runnable {
@Override
public void run() {
System.out.println("Thread is running: " + Thread.currentThread().getName());
}
}
public class ThreadDemo {
public static void main(String[] args) {
Thread thread1 = new Thread(new MyRunnable());
Thread thread2 = new Thread(new MyRunnable());
thread1.start();
thread2.start();
}
}
Why this matters: The above code demonstrates how to create and start threads in Java. Understanding how to manage thread execution is crucial for troubleshooting potential issues in concurrent applications.
Common Issues in Concurrent Application Testing
- Race Conditions
Race conditions occur when two or more threads access shared data and try to change it simultaneously. The timing of thread execution can lead to unpredictable outcomes. This is often due to a lack of proper synchronization.
Example of a Race Condition
public class RaceConditionDemo {
private int counter = 0;
public void increment() {
counter++; // This line can cause a race condition
}
public static void main(String[] args) {
RaceConditionDemo demo = new RaceConditionDemo();
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
demo.increment();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
demo.increment();
}
});
thread1.start();
thread2.start();
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Final counter value: " + demo.counter);
}
}
Why this matters: Without proper synchronization mechanisms, you could end up with inconsistent data. To avoid race conditions, make use of synchronization blocks or the synchronized
keyword.
- Deadlocks
A deadlock occurs when two or more threads are blocked forever, each waiting on the other to release a lock. This situation typically arises from poor resource management.
Example of a Deadlock
public class DeadlockDemo {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void method1() {
synchronized (lock1) {
synchronized (lock2) {
System.out.println("Method 1 executed");
}
}
}
public void method2() {
synchronized (lock2) {
synchronized (lock1) {
System.out.println("Method 2 executed");
}
}
}
public static void main(String[] args) {
DeadlockDemo demo = new DeadlockDemo();
Thread thread1 = new Thread(demo::method1);
Thread thread2 = new Thread(demo::method2);
thread1.start();
thread2.start();
}
}
Why this matters: In this example, each thread is waiting for the other to release the locks—resulting in a deadlock. To avoid this, you can enforce a consistent lock order or use timeouts.
- Starvation
Starvation occurs when a thread does not get CPU time because other threads are continuously consuming resources. This is often seen in scenarios involving priority scheduling.
How to Identify: You can monitor thread states and resource usage statistics.
Example of Starvation
public class StarvationDemo {
public static void main(String[] args) {
Runnable lowPriorityTask = () -> {
while (true) {
// Low-priority task
}
};
Runnable highPriorityTask = () -> {
while (true) {
// High-priority task
}
};
Thread lowPriorityThread = new Thread(lowPriorityTask);
Thread highPriorityThread = new Thread(highPriorityTask);
lowPriorityThread.setPriority(Thread.MIN_PRIORITY);
highPriorityThread.setPriority(Thread.MAX_PRIORITY);
lowPriorityThread.start();
highPriorityThread.start();
}
}
Why this matters: The low-priority task may never get CPU time if high-priority tasks keep executing. Balancing thread priorities and using methods to yield CPU cycles can be effective strategies.
- Livelocks
In a livelock situation, threads are not blocked, but they keep changing states in response to each other without making progress. This often appears as a situation where threads are “alive” but not performing useful work.
Example of Livelock
public class LivelockDemo {
private int count = 0;
public void increment() {
while (count < 10) {
if (Math.random() > 0.5) {
count++;
System.out.println("Incremented: " + count);
}
}
}
public static void main(String[] args) {
LivelockDemo demo = new LivelockDemo();
Thread thread1 = new Thread(demo::increment);
Thread thread2 = new Thread(demo::increment);
thread1.start();
thread2.start();
}
}
Why this matters: Both threads are reacting to each other's actions, which can lead to a situation where neither makes progress. Identifying such patterns is key to troubleshooting and resolving these issues.
Testing Strategies for Concurrent Applications
-
Use Thread Safety Libraries: Libraries such as
java.util.concurrent
make it easier to manage threads and avoid issues like race conditions and deadlocks. -
Stress Testing: This involves pushing your application beyond its limits to observe how it behaves under extreme conditions. Use tools like JMeter or Gatling to simulate concurrent user access.
-
Lock Ordering: Enforce a consistent order in which locks are acquired to avoid deadlocks. This method ensures threads acquire locks in a predictable manner.
-
Instrumentation and Monitoring: Use tools like VisualVM or JConsole to monitor running threads and their states. This can help in diagnosing performance bottlenecks.
-
Static Code Analysis: Employ tools like SonarQube to analyze code quality and detect concurrency issues before they make it to production.
-
Automated Testing Frameworks: Utilize frameworks designed for testing concurrent behaviors, such as JUnit with additional libraries for threading.
Bringing It All Together
Troubleshooting common issues in concurrent application testing is crucial for ensuring the robustness and reliability of your software. By understanding potential pitfalls such as race conditions, deadlocks, starvation, and livelocks, developers can implement effective strategies to prevent them.
Applying good practices, such as using proper synchronization, ensuring fair scheduling, and leveraging testing tools, will enhance your concurrent application’s stability. Ultimately, thorough testing and proactive troubleshooting can lead to a smoother user experience and a more robust application.
For further reading on concurrency in Java and its testing, consider checking the official Java Concurrency Documentation.
Happy coding!
Checkout our other articles