Transforming Futures into Observables: Common Pitfalls
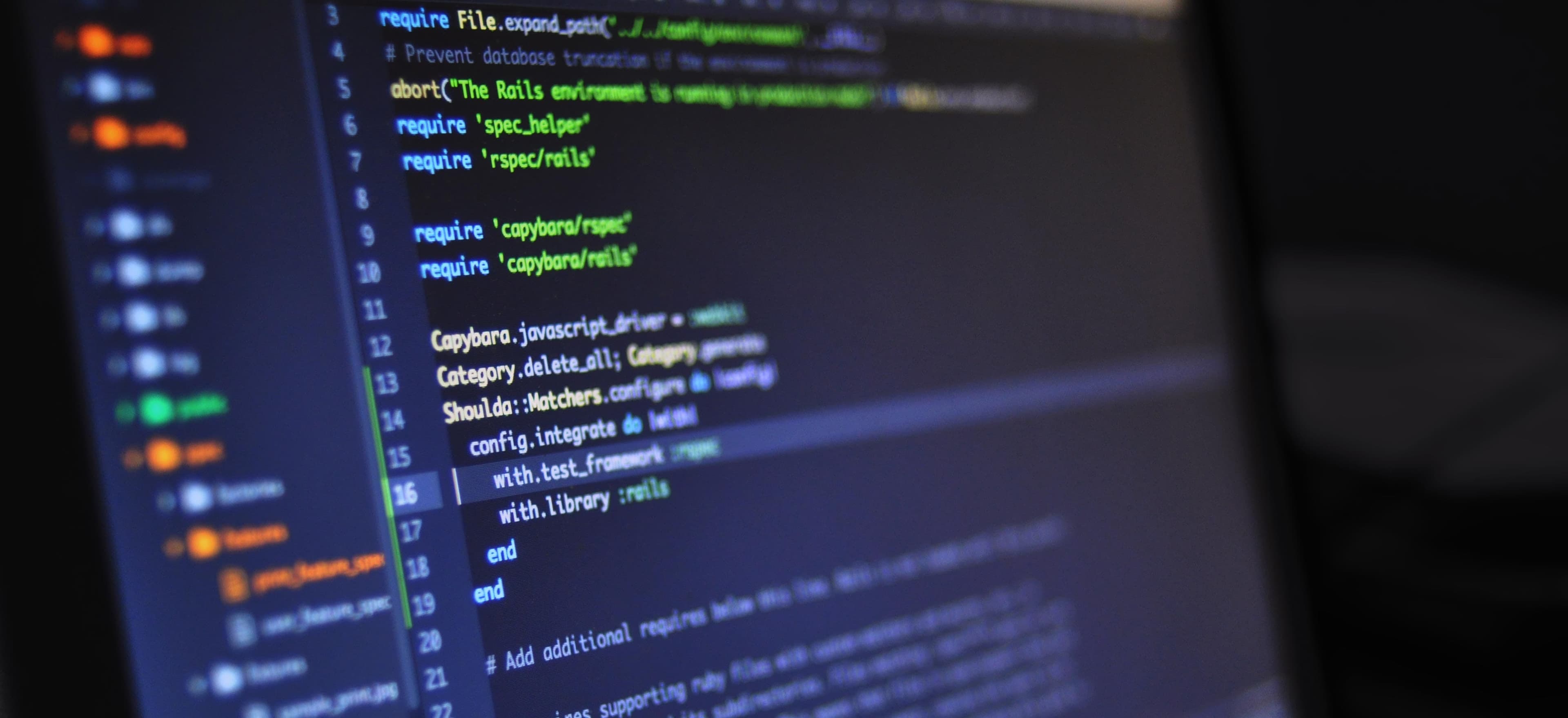
- Published on
Transforming Futures into Observables: Common Pitfalls
In the realm of asynchronous programming in Java, developers often find themselves needing to transform Future
tasks into Observable
streams. This transformation allows for more reactive programming paradigms that can simplify complex event handling and asynchronous processing. However, this transition can introduce several common pitfalls that can lead to unexpected behavior and increased complexity.
In this blog post, we’ll explore the nuances of transforming Futures
into Observables
, identify common pitfalls, and demonstrate best practices with code examples. Our goal is to equip you with the knowledge to avoid these traps and utilize the power of reactive programming effectively.
What Are Futures and Observables?
Before diving into the specifics, let's clarify what Future
and Observable
providers are in Java.
-
Future: A
Future
represents the result of an asynchronous computation. It provides methods to retrieve the result once it's available and can be used to check if the computation is complete or whether it was canceled. -
Observable: An
Observable
is part of the reactive programming paradigm, which allows you to work with streams of data. It enables you to emit multiple items over time and allows subscribers to react to changes when new items are emitted or when an event occurs.
Why Transform Futures into Observables?
The primary benefit of transforming a Future
into an Observable
is to take advantage of the reactive programming model, which offers more flexibility, improves code readability, and enhances data handling. By converting Futures
to Observables
, you can:
-
Handle Multiple Events: Unlike
Futures
, which produce a single result,Observables
can emit multiple values over time. -
Chaining Operations: This allows for easy chaining of operations such as filtering, mapping, or reducing.
-
Better Error Handling:
Observables
can handle errors more gracefully thanFutures
, allowing a more declarative way to manage exceptions.
Transforming a Future to an Observable
Let's take a closer look at how you can convert a Future
into an Observable
using RxJava (a popular Java framework for reactive programming).
Here's a simple example to illustrate this transformation:
import io.reactivex.Observable;
import java.util.concurrent.CompletableFuture;
public class FutureToObservableExample {
public static void main(String[] args) {
// Create a CompletableFuture
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(1000); // Simulate a long-running task
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Hello from Future!";
});
// Transform the Future into an Observable
Observable<String> observable = Observable.fromFuture(future);
// Subscribe to the Observable
observable.subscribe(
result -> System.out.println("Received: " + result),
Throwable::printStackTrace,
() -> System.out.println("Completed!")
);
}
}
Commentary on the Code
-
CompletableFuture: This class is used here to simulate a task that completes in the future. We have a long-running task that represents a common asynchronous operation.
-
Observable.fromFuture(): This method converts the
Future
into anObservable
, allowing us to handle the result reactively. -
Subscription: By subscribing to the
Observable
, we define what to do when a value is emitted, when an error occurs, and when the observable sequence is finished.
Common Pitfalls When Transforming Futures into Observables
While the transformation seems straightforward, several pitfalls can emerge:
1. Ignoring Completion States
One of the biggest mistakes is failing to handle CompletableFuture's completion states properly. Futures
can be completed exceptionally, and you need to ensure that your observable account for this as well.
CompletableFuture<String> futureWithError = CompletableFuture.supplyAsync(() -> {
throw new RuntimeException("Error occurred!");
});
// Transform and subscribe
Observable<String> observableWithError = Observable.fromFuture(futureWithError);
observableWithError.subscribe(
System.out::println,
e -> System.err.println("Error: " + e.getMessage()), // Handle error gracefully
() -> System.out.println("Completed!")
);
2. Blocking the Main Thread
If a Future
takes a long time to complete, it might block your main thread if you are not handling it asynchronously. Always ensure that you are running blocking calls on a separate thread.
3. Using Unbounded Resources
When dealing with observables from Futures
, it's easy to lose track of resource management. Ensure you are properly disposing of subscriptions and managing memory. Failing to do so can lead to memory leaks.
Disposable disposable = observable.subscribe(
result -> System.out.println("Got: " + result),
Throwable::printStackTrace,
() -> System.out.println("Done!")
);
// Dispose of when no longer needed
disposable.dispose();
4. Error Handling: Misuse of Try-Catch
When converting a Future
to an Observable
, try-catch blocks may not catch exceptions thrown in an asynchronous context. You should utilize onError
callbacks to manage errors in a reactive way.
5. Concurrency Issues
If your Future
performs writes to shared resources, you may run into concurrency issues. Proper synchronization mechanisms such as synchronized
blocks or Locks
should be used.
Best Practices for Transformation
To avoid pitfalls, here are some best practices:
-
Always Handle Errors: Use appropriate onError callbacks in subscriptions to gracefully handle any errors that might arise.
-
Avoid Blocking: Use asynchronous methods and avoid blocking patterns in reactive programming.
-
Manage Resources Carefully: Ensure subscriptions are disposed of properly to free up resources.
-
Be Mindful of Concurrency: Protect shared resources using synchronization when necessary.
-
Testing: Regularly test both
Future
andObservable
logic to ensure they behave as expected in different conditions.
In Conclusion, Here is What Matters
Transforming Futures
into Observables
can significantly enhance the capabilities of your Java applications, offering a smoother, more reactive programming model. However, navigating the common pitfalls associated with this transformation is crucial to ensuring your code remains efficient and free from unexpected behaviors.
By understanding the intricacies of both paradigms and adhering to best practices, you can transform asynchronous tasks into reactive streams without falling prey to common pitfalls.
Further Reading
- RxJava Documentation
- CompletableFuture Documentation
- Reactive Programming in Java
By mastering these concepts, you're not just coding; you're innovating ways of thinking about asynchronous tasks in Java programming. Happy coding!
Checkout our other articles