Solving Common JavaFX UI Design Challenges
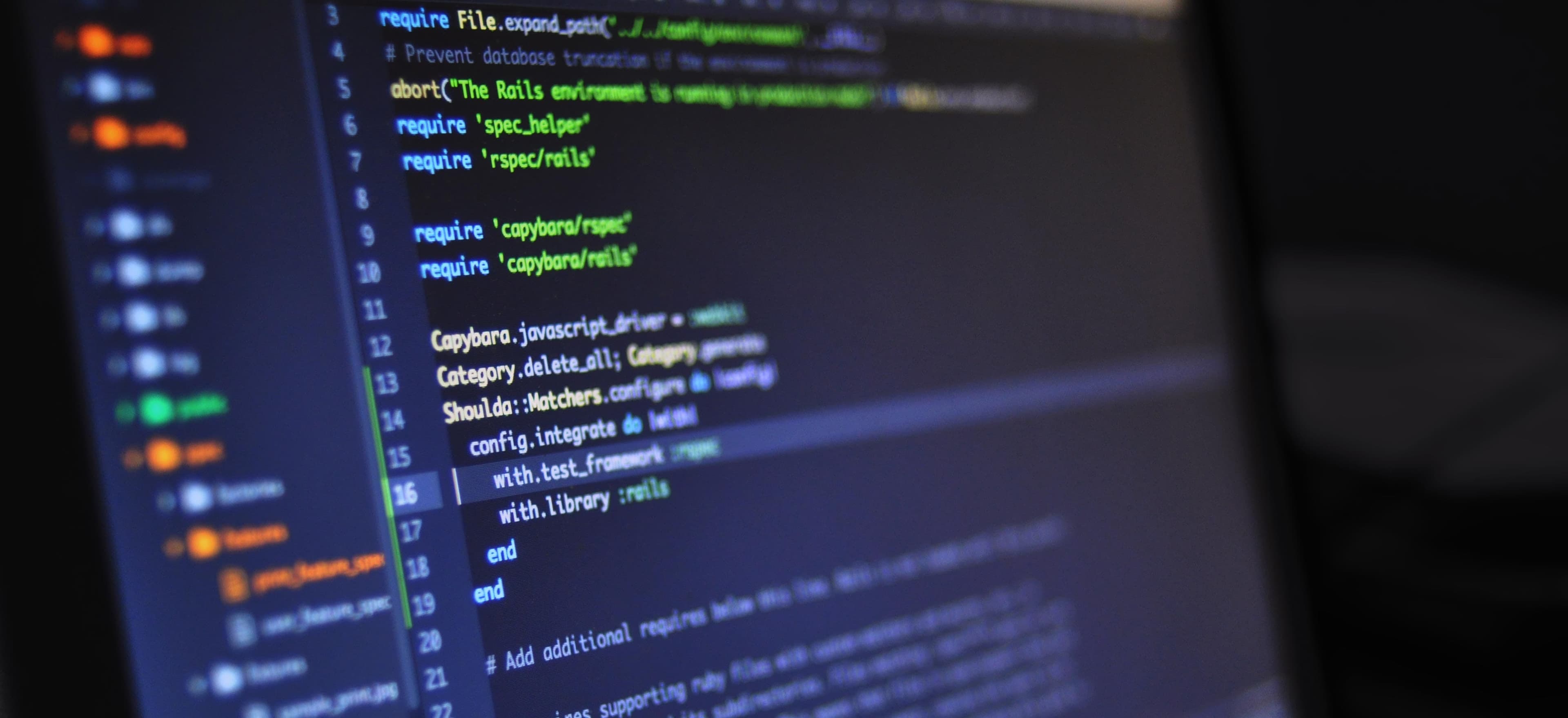
- Published on
Solving Common JavaFX UI Design Challenges
JavaFX is a robust framework for building rich desktop applications in Java. While it empowers developers to create visually appealing applications with ease, it also presents several design challenges. Understanding how to navigate these challenges is crucial for delivering high-quality user interfaces (UIs).
In this blog post, we explore common JavaFX UI design challenges and provide solutions that are both efficient and elegant. We will cover layout management, styling, responsiveness, and handling user input effectively. By the end, you will have a toolkit to tackle these issues in your own JavaFX applications.
Understanding Layout Management
The Challenge
One of the biggest challenges in JavaFX development is managing layouts. With a myriad of layout containers available, developers often struggle to find the most suitable one for their application needs. If not handled well, this can lead to disorganized and unintuitive UIs.
Solution
JavaFX offers several layout panes, such as VBox
, HBox
, GridPane
, and BorderPane
. Here's a brief overview:
- VBox: Stacks nodes vertically.
- HBox: Stacks nodes horizontally.
- GridPane: Organizes nodes in a flexible grid.
- BorderPane: Divides the scene into five regions: top, bottom, left, right, and center.
Use the right container to keep your UI clean and organized. For instance, if you want a form layout, GridPane
is an excellent choice.
Example Code: Using GridPane
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class FormExample extends Application {
@Override
public void start(Stage primaryStage) {
GridPane grid = new GridPane();
grid.setHgap(10);
grid.setVgap(10);
// Adding controls to the grid
grid.add(new Label("Name:"), 0, 0);
grid.add(new TextField(), 1, 0);
grid.add(new Label("Email:"), 0, 1);
grid.add(new TextField(), 1, 1);
grid.add(new Button("Submit"), 1, 2);
Scene scene = new Scene(grid, 300, 200);
primaryStage.setTitle("JavaFX Form Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this code:
- We create a simple form using
GridPane
. - The
setHgap
andsetVgap
methods provide spacing between elements. - Each control is added with specific grid coordinates for precise placement.
Best Practices
- Choose layout panes based on content type and user interaction.
- Keep in mind the visual hierarchy.
- Regularly test the interface as you build to avoid misalignments.
Enhancing UI with CSS
The Challenge
While JavaFX allows for some basic styling, developers often find it challenging to create professional-looking applications without a good understanding of CSS.
Solution
CSS (Cascading Style Sheets) can be applied in JavaFX to enhance the look and feel of your application. This allows for separation of design from logic, promoting cleaner code.
Example Code: Styling with CSS
/* styles.css */
.label {
font-size: 14px;
text-fill: #333;
}
.button {
background-color: #4CAF50;
border: none;
color: white;
padding: 10px 20px;
}
.button:hover {
background-color: #45a049;
}
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class CSSExample extends Application {
@Override
public void start(Stage primaryStage) {
VBox vbox = new VBox(new Label("Welcome to JavaFX"), new Button("Click Me"));
vbox.setSpacing(10);
Scene scene = new Scene(vbox, 300, 200);
scene.getStylesheets().add("styles.css");
primaryStage.setTitle("CSS Styling Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In the above example:
- We define a CSS file that styles labels and buttons.
- JavaFX allows us to attach this stylesheet to the scene.
- It’s important to follow best practices in naming CSS classes to facilitate scalability.
Best Practices
- Keep styling rules simple and logical.
- Utilize classes for reusable styles.
- Regularly test designs on different platforms if your application supports cross-platform usage.
Building Responsive UIs
The Challenge
With the diverse range of screen sizes and resolutions, creating a responsive UI that adapts to different platforms can be a daunting task.
Solution
Use layout managers that facilitate flexibility. For instance, VBox
, HBox
, and BorderPane
automatically adjust their children based on the current window size. Setting minimum and maximum sizes can also help control layout behavior effectively.
Example Code: Responsive UI
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class ResponsiveUIExample extends Application {
@Override
public void start(Stage primaryStage) {
BorderPane borderPane = new BorderPane();
borderPane.setCenter(new Button("Center Button"));
borderPane.setTop(new Button("Top Button"));
borderPane.setLeft(new Button("Left Button"));
borderPane.setRight(new Button("Right Button"));
borderPane.setBottom(new Button("Bottom Button"));
Scene scene = new Scene(borderPane, 400, 300);
primaryStage.setTitle("Responsive UI Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this example:
- The
BorderPane
automatically arranges all buttons based on the window size. - This responsive design keeps the UI manageable as the app scales.
Best Practices
- Design with scalability in mind.
- Regularly test on various screen sizes and resolutions.
- Minimize fixed dimensions; favor relative positioning.
Handling User Input
The Challenge
Handling user input gracefully, including validation and feedback, is essential for creating user-friendly interfaces. Invalid inputs or lack of user feedback can lead to a frustrating experience.
Solution
JavaFX provides various input controls, from TextField
to ComboBox
, that can make capturing user input smooth. Implementing validators helps in ensuring data integrity.
Example Code: Input Validation
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class InputValidationExample extends Application {
@Override
public void start(Stage primaryStage) {
TextField textField = new TextField();
Button button = new Button("Submit");
Label feedbackLabel = new Label();
button.setOnAction(e -> {
String input = textField.getText();
if (input.isEmpty()) {
feedbackLabel.setText("Input cannot be empty.");
} else {
feedbackLabel.setText("Input received: " + input);
}
});
VBox vbox = new VBox(textField, button, feedbackLabel);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setTitle("Input Validation Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this code snippet:
- We create a simple input form with a validation response.
- If the text field is empty upon submission, feedback is provided.
- This enhances user experience and encourages user engagement.
Best Practices
- Validate input promptly and clearly.
- Provide contextual feedback that helps the user correct errors.
- Use dialog boxes or alerts to inform users of significant issues, as seen in JavaFX documentation on Alerts for more advanced feedback handling.
My Closing Thoughts on the Matter
JavaFX UI design presents its own set of challenges, but with a strategic approach, these challenges can be overcome. By understanding layout management, enhancing UIs with CSS, creating responsive designs, and effectively handling user input, you can create applications that are not only functional but also aesthetically pleasing and user-friendly.
As you embark on your JavaFX journey, remember that the key to mastery is practice and continuous learning. For further reading, check out JavaFX Official Documentation to deepen your understanding and explore more capabilities of this exceptional framework. Happy coding!
Checkout our other articles