Top Challenges New Developers Face in App Development
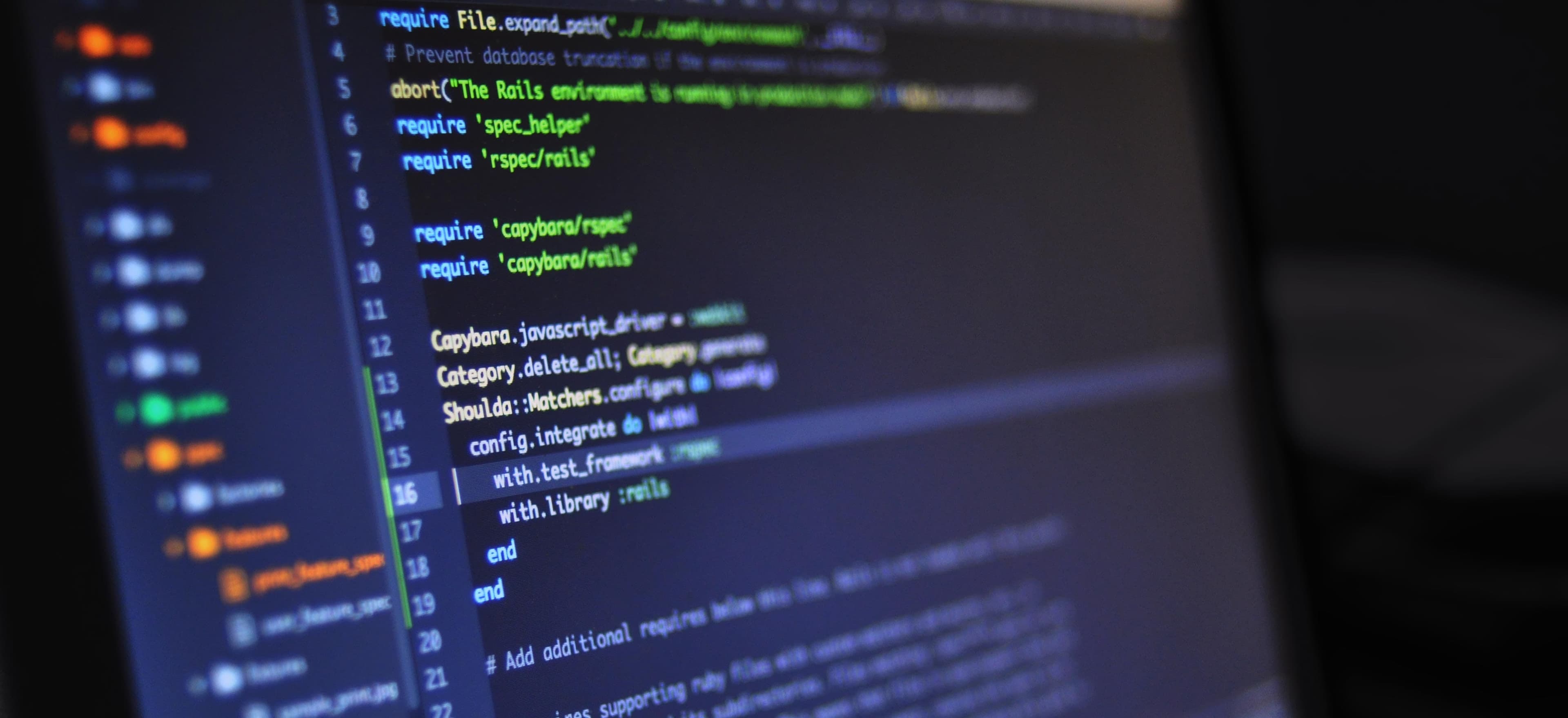
- Published on
Top Challenges New Developers Face in App Development
App development is an exciting yet demanding journey, especially for new developers. Learning to transform ideas into functional applications features both rewarding moments and significant hurdles. This blog post will identify and explore the top challenges new developers encounter in app development while providing insights that can help navigate the learning curve.
1. Understanding the Development Ecosystem
The Complexity of Frameworks and Tools
The app development ecosystem comprises various programming languages, frameworks, and tools. New developers often feel overwhelmed by the plethora of choices. Should they use React Native, Flutter, or native development in Swift or Kotlin? Each option has its own learning curve, advantages, and drawbacks.
Key Takeaway:
Research and choose a framework that aligns with your project needs and personal development style. For instance, if you are looking to create cross-platform apps, frameworks like React Native offer great flexibility.
Code Snippet: Simple React Native Component
import React from 'react';
import { Text, View } from 'react-native';
const App = () => {
return (
<View>
<Text>Hello, World!</Text>
</View>
);
};
export default App;
This basic example demonstrates how React Native allows you to create simple UI elements using JavaScript and JSX. Understanding the syntax is key to mastering more complex functionalities as you progress.
2. Debugging Problems
The Nightmare of Bugs
Every developer knows that bugs are an inevitable part of coding. However, for beginners, debugging can be a daunting task. Identifying the source of bugs and applying the appropriate fixes often requires a deep understanding of the codebase and experience.
Key Takeaway:
Don’t get discouraged by bugs. Utilize debugging tools available in your chosen IDE. For example, Chrome DevTools can significantly enhance your debugging process when developing web applications.
Code Snippet: Debugging with Console.log()
function add(a, b) {
console.log('Adding:', a, b); // Debugging step
return a + b;
}
const result = add(5, '10'); // Issues arise due to type coercion
console.log('Result:', result); // Check output
In this snippet, we leverage console.log()
as a debugging method. It helps ascertain the input values, so you can track where issues develop, particularly during type conversions.
3. Managing Version Control
The Importance of Collaboration
As projects grow, so does the need for effective collaboration. Understanding version control systems like Git can be overwhelming for newcomers. Learning commands, managing branches, and resolving merge conflicts can feel insurmountable.
Key Takeaway:
Start with the basics of Git to manage your codebase efficiently. Familiarizing yourself with version control fundamentals is essential not only for solo projects but also for teamwork.
Code Snippet: Basic Git Commands
# Initialize a new Git repository
git init
# Stage changes
git add .
# Commit changes
git commit -m "Initial commit"
# Create a new branch
git checkout -b feature-branch
# Merge a branch
git merge feature-branch
Understanding these commands lays the groundwork for successful version control. It allows you to track changes and manage different project versions efficiently.
4. User Interface (UI) and User Experience (UX) Challenges
Designing an Intuitive Interface
Creating an intuitive and visually appealing user interface is crucial for any app’s success. New developers may struggle with design principles and how to create a layout that enhances user experience.
Key Takeaway:
Invest time in learning UI/UX principles. Even a basic understanding of user-centered design can dramatically enhance the quality of your application. Websites like Dribbble and Behance offer a wealth of inspiration.
Code Snippet: Basic CSS for Styling
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f0f0f0;
}
.button {
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
Using CSS effectively can transform a dull layout into an engaging user interface. These styles enhance visual appeal and usability, potentially improving user retention.
5. Learning How to Test
The Testing Conundrum
Testing is an essential part of the software development lifecycle, yet many new developers overlook its importance. Understanding how to write test cases, incorporate unit testing, and execute performance testing can be challenging.
Key Takeaway:
Integrate testing from the beginning of the development process. It not only catches bugs but also enhances the overall quality of your code. Comprehensive testing frameworks like JUnit for Java and Jest for JavaScript are great tools for new developers.
Code Snippet: Simple Test Example in Jest
function multiply(a, b) {
return a * b;
}
test('multiplies 2 and 3 to equal 6', () => {
expect(multiply(2, 3)).toBe(6);
});
This snippet showcases how to create a simple test using Jest. Testing ensures that your functions behave as expected, helping identify errors early in the development process.
6. Keeping Up with the Latest Trends
The Fast-Paced Development Landscape
Technology evolves rapidly, and new tools, frameworks, and best practices emerge almost daily. New developers may find it challenging to keep abreast of these changes, which can hinder their growth and adaptation in this competitive field.
Key Takeaway:
Stay updated through online communities, forums, and resources. Platforms like Stack Overflow and GitHub can be invaluable in learning and sharing knowledge.
7. Time Management and Productivity
Balancing Development Time
Finally, managing time effectively can present a challenge. New developers often struggle to balance learning new concepts, implementing features, and dealing with deadlines. Properly managing time is crucial to avoid burnout and maintain productivity.
Key Takeaway:
Adopt methodologies such as Agile or Kanban. Tools like Trello or Asana can help you prioritize tasks and improve productivity.
In Conclusion, Here is What Matters
Navigating the challenges of app development as a newcomer requires resilience, patience, and continuous learning. The hurdles may seem daunting, but each challenge also presents a valuable opportunity for growth. By arming yourself with the right tools, frameworks, and knowledge, you can smoothly transition from a novice to a proficient developer. Remember, every expert was once a beginner!
For further reading and resources on overcoming these challenges, you can explore articles on Codecademy and freeCodeCamp. Embrace the journey, and happy coding!