Unlocking Pitfalls: Common Mistakes with Functional Interfaces
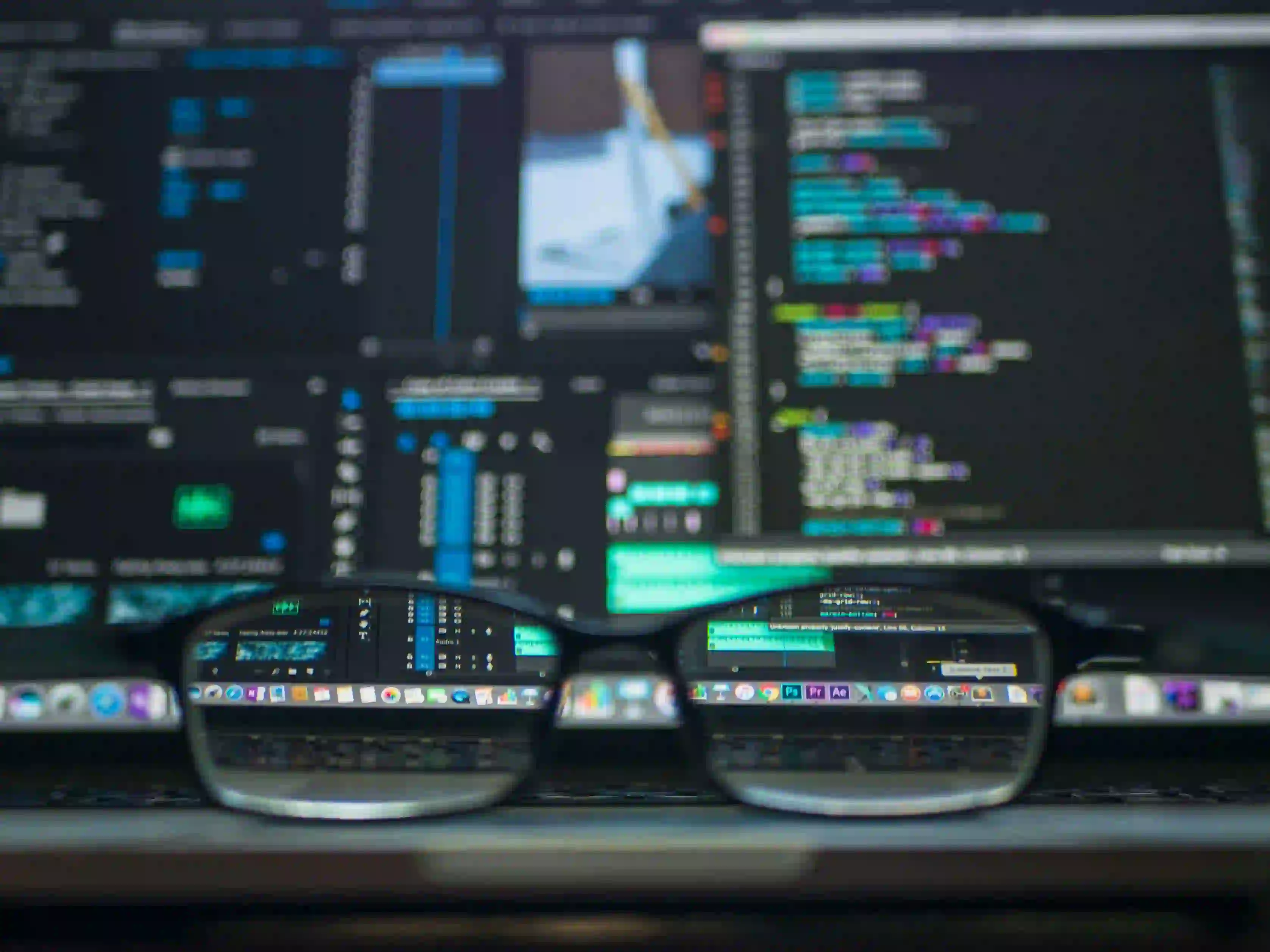
Unlocking Pitfalls: Common Mistakes with Functional Interfaces in Java
Functional programming has gained traction in the world of Java, especially since the introduction of lambda expressions and functional interfaces with Java 8. Although these features have enriched the language, some pitfalls may trip you up. In this blog post, we’ll dive deep into the common mistakes developers make when working with functional interfaces and how to avoid them.
What is a Functional Interface?
A functional interface is any interface that contains exactly one abstract method. They serve as the baseline for lambda expressions, enabling developers to write cleaner and more concise code. Common examples include Runnable
, Callable
, and Comparator
.
Here’s a simple example of a functional interface:
@FunctionalInterface
public interface MyFunction {
int apply(int x);
}
This interface defines a single method, apply
, which takes an integer and returns an integer.
Why Use Functional Interfaces?
Functional interfaces promote higher-order functions, where you can pass functions as parameters, return them from other functions, or store them in variables. This leads to more flexible and reusable code.
For a deeper understanding of functional features, consider exploring the Java Documentation.
Common Mistakes
1. Missing the @FunctionalInterface Annotation
Pitfall: One of the most frequently made mistakes is not using the @FunctionalInterface
annotation. While it's not mandatory, this annotation acts as a safeguard. It alerts the compiler if another abstract method is accidentally added.
Why It Matters: Using the annotation ensures that your intention is clear and helps catch errors early in development.
Example:
@FunctionalInterface
public interface MyFunctionalInterface {
void execute();
// Uncommenting the next line will cause a compilation error
// void doAnotherThing();
}
If you add another abstract method, the compiler will throw an error, guiding you to adhere to the functional interface contract.
2. Overlooking Serialization
Pitfall: Some developers overlook serialization when using lambdas or functional interfaces. If you attempt to serialize an object that stores a lambda expression, it might lead to NotSerializableException
.
Solution: Implement the Serializable
interface on your functional interface. Just make sure that any variables captured by your lambda are also serializable.
Example:
import java.io.Serializable;
@FunctionalInterface
public interface SerializableFunction<T, R> extends Serializable {
R apply(T t);
}
This inclusion helps you avoid serialization errors when using lambdas.
3. Using Non-Functional Interfaces
Pitfall: Confusing functional interfaces with regular interfaces can create unexpected results, especially when multiple abstract methods exist.
Why It Matters: Regular interfaces can lead to cognitive overload, defeating the purpose of functional programming.
Example:
public interface NotFunctional {
void method1();
void method2();
}
// This will not work with a lambda
NotFunctional nf = () -> System.out.println("Hello"); // Compilation error!
Always ensure that the interfaces you are using are indeed functional, with a single abstract method.
4. Neglecting Type-Safety
Pitfall: Another common error is ignoring type parameters in functional interfaces. Java’s type system can guide you, but if you omit generics, you may run into issues.
Why It Matters: This can lead to ClassCastException or can prevent your code from being as reusable as it can be.
Example:
@FunctionalInterface
public interface StringToInt {
int convert(String str);
}
// Using it appropriately enhances type safety
StringToInt lengthFunction = str -> str.length();
int length = lengthFunction.convert("Hello"); // Returns 5
Here, the generic type ensures you can only pass a String
, promoting more reliable code.
5. Immutability Confusion
Pitfall: Functional interfaces often promote immutability. However, developers might mistakenly use mutable state within a lambda, leading to unexpected side effects.
Why It Matters: A key principle in functional programming is maintaining state without side effects. Mutability contradicts this and can make debugging challenging.
Example:
public class Example {
private static int number = 0;
public static void main(String[] args) {
Runnable addOne = () -> number++;
addOne.run();
System.out.println(number); // Outputs 1
}
}
// Better way
public class ImmutableExample {
private static int number = 0;
public static void main(String[] args) {
int incrementedValue = increment(number);
System.out.println(incrementedValue); // Outputs 1
}
private static int increment(int num) {
return num + 1;
}
}
In the first example, number
is modified, whereas in the second, we maintain immutability, passing the value instead.
6. Overusing Functional Interfaces
Pitfall: It’s easy to fall into the trap of overusing functional interfaces, leading to code that's difficult to read and maintain. While functional programming can simplify code, it shouldn’t come at the cost of clarity.
Why It Matters: Striking a balance between functional and procedural programming styles is crucial to creating maintainable software.
Consider this example of excessive chaining:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.map(String::toUpperCase)
.sorted()
.collect(Collectors.toList());
While this is perfectly valid, excessive chaining can reduce code clarity. Sometimes, breaking functions into different methods can improve readability:
public List<String> getFilteredNames(List<String> names) {
List<String> filteredNames = filterNames(names);
return toUpperCaseAndSort(filteredNames);
}
private List<String> filterNames(List<String> names) {
return names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
}
private List<String> toUpperCaseAndSort(List<String> names) {
return names.stream()
.map(String::toUpperCase)
.sorted()
.collect(Collectors.toList());
}
Splitting logic into smaller methods often makes code easier to understand.
The Closing Argument
Functional interfaces in Java have revolutionized the way developers write code, offering cleaner and more maintainable solutions. However, like any powerful tool, they come with their set of challenges. By being aware of the common mistakes—like neglecting the @FunctionalInterface
annotation, overlooking serialization issues, or confusing immutability—you can harness the power of functional programming without falling into these traps.
Always remember that well-written code is clear. Prioritize readability and understanding without sacrificing the benefits that functional programming offers.
For further reading, check out Baeldung's guide on Functional Interfaces, which provides additional examples and explanations.
Happy coding!