Mastering Java EE: Overcoming Common Development Pitfalls
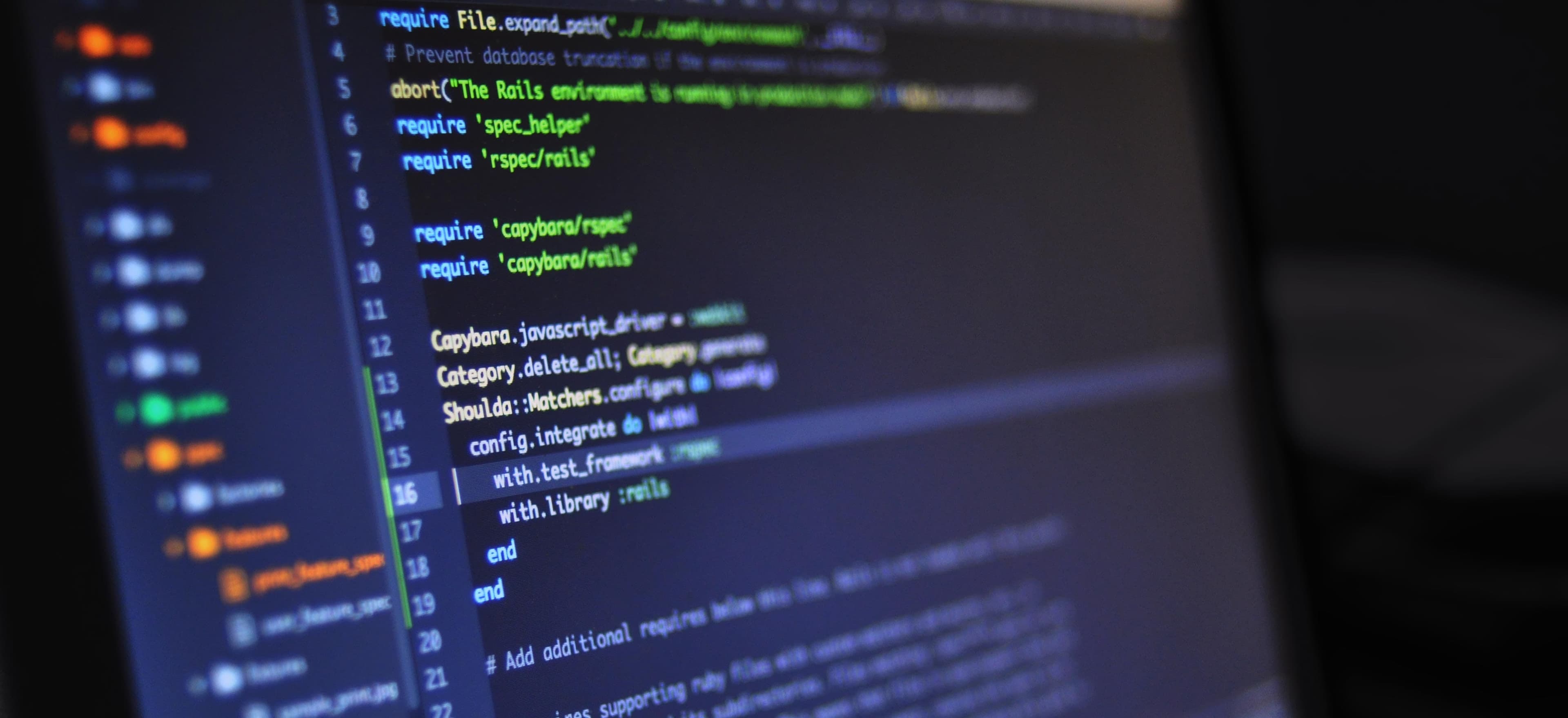
- Published on
Mastering Java EE: Overcoming Common Development Pitfalls
Java EE (Enterprise Edition), now known as Jakarta EE, provides a robust framework for building enterprise-level applications. With its powerful capabilities, it allows developers to create scalable, maintainable, and secure applications. However, like any technology, it presents challenges that developers must navigate. This article highlights common pitfalls in Java EE development and presents ways to overcome them, enabling you to master the framework effectively.
Understanding Java EE
Before diving into the pitfalls, it's essential to recognize what Java EE offers. Java EE is designed to be modular and scalable. Here are its main components:
- Servlets: Manage requests and responses in web applications.
- JavaServer Faces (JSF): A framework for building user interfaces.
- Enterprise JavaBeans (EJB): Handle business logic.
- Java Persistence API (JPA): Simplifies database interactions.
Understanding these components puts you on the right path to recognizing potential pitfalls.
Common Development Pitfalls
1. Misunderstanding the Architecture
Java EE operates on a multi-tier architecture: Presentation, Business Logic, and Data Access layers. A common mistake is conflating responsibilities among these layers.
Tip: Adhere to the Single Responsibility Principle. Each layer should perform only its intended functions.
Code Example: Here's how to separate concerns in a simple Java EE application:
// Presentation Layer
@WebServlet("/user")
public class UserServlet extends HttpServlet {
@Inject
private UserService userService;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
List<User> users = userService.getAllUsers();
request.setAttribute("users", users);
request.getRequestDispatcher("/user.jsp").forward(request, response);
}
}
In this example, the UserServlet
handles the presentation logic, while the actual user logic is moved to a UserService
, adhering better to the architecture.
2. Ignoring Dependency Injection
Java EE provides a powerful Dependency Injection (DI) mechanism to manage application components. Developers often neglect DI, resulting in tight coupling and diminished testability.
Tip: Always use the DI framework. This will simplify your code and enhance its readability.
Code Example:
// EJB with Dependency Injection
@Stateless
public class UserService {
@Inject
private UserDAO userDAO;
public List<User> getAllUsers() {
return userDAO.findAll();
}
}
Using DI here ensures that the UserService
class is modular and testable.
3. Improper Transaction Management
Java EE facilitates transaction management, but developers often misuse it, leading to data inconsistencies.
Tip: Use container-managed transactions (CMT) instead of programmatic transactions when possible.
Code Example:
@Stateless
public class AccountService {
@TransactionAttribute(TransactionAttributeType.REQUIRED)
public void transferFunds(Account from, Account to, double amount) {
from.debit(amount);
to.credit(amount);
}
}
With @TransactionAttribute
, the container manages the transaction's lifecycle. This results in more maintainable code.
4. Failing to Use Contexts and Dependency Injection (CDI) Properly
CDI plays a crucial role in creating a well-structured Java EE application. Many developers either underutilize or misuse it.
Tip: Familiarize yourself with scopes like @RequestScoped, @SessionScoped, and @ApplicationScoped.
Code Example:
@RequestScoped
public class UserBean {
private String username;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
}
By using @RequestScoped
, this bean is instantiated per HTTP request, optimizing resource management.
5. Not Leveraging JPA Effectively
JPA simplifies database operations. However, a frequent oversight is the inefficient use of entity management and queries.
Tip: Utilize the criteria API or JPQL for dynamic queries rather than constructing raw SQL strings.
Code Example:
public List<User> findUsersWithCriteria(String username) {
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<User> query = cb.createQuery(User.class);
Root<User> user = query.from(User.class);
query.select(user).where(cb.equal(user.get("username"), username));
return entityManager.createQuery(query).getResultList();
}
This style provides both readability and safety against SQL injection attacks.
6. Overcomplicating Configurations
Java EE provides XML descriptors for configurations, but many developers choose to overcomplicate them. This leads to confusion and errors.
Tip: Prefer annotations over XML configurations where possible. They streamline configurations and reduce boilerplate code.
Code Example:
@ApplicationScoped
public class ConfiguredBean {
// No need for XML configuration.
}
By avoiding XML, you focus more on the codebase instead of configuration files.
7. Neglecting Unit Testing
Testing is paramount in software development. Unfortunately, Java EE developers often overlook unit tests due to tight coupling between components.
Tip: Implement mock objects and leverage testing frameworks like JUnit and Mockito.
Code Example:
@RunWith(MockitoJUnitRunner.class)
public class UserServiceTest {
@InjectMocks
private UserService userService;
@Mock
private UserDAO userDAOMock;
@Test
public void testGetAllUsers() {
when(userDAOMock.findAll()).thenReturn(new ArrayList<>());
List<User> users = userService.getAllUsers();
assertTrue(users.isEmpty());
}
}
In this example, mocking the UserDAO
allows for effective testing of UserService
without depending on the actual database.
8. Overusing Application Servers
While application servers like WildFly and GlassFish provide powerful Java EE environments, over-reliance can be a pitfall.
Tip: When developing, consider using lightweight frameworks like MicroProfile for microservices.
Final Considerations
Mastering Java EE involves understanding its architecture, effective use of its features, and staying mindful of common pitfalls. By addressing these issues early in your development process, you will enhance your productivity and ensure your applications are robust, maintainable, and scalable.
For more information on mastering Java EE, consider visiting the Jakarta EE website for guidelines and resources. You can also check out Baeldung's comprehensive tutorials on Java EE to deepen your understanding.
Implement these strategies in your next project and watch as the complexity simplifies, paving the way for clearer code and smoother development cycles. Happy coding!
Checkout our other articles