Overcoming Event Handling Complexity in Guava's EventBus
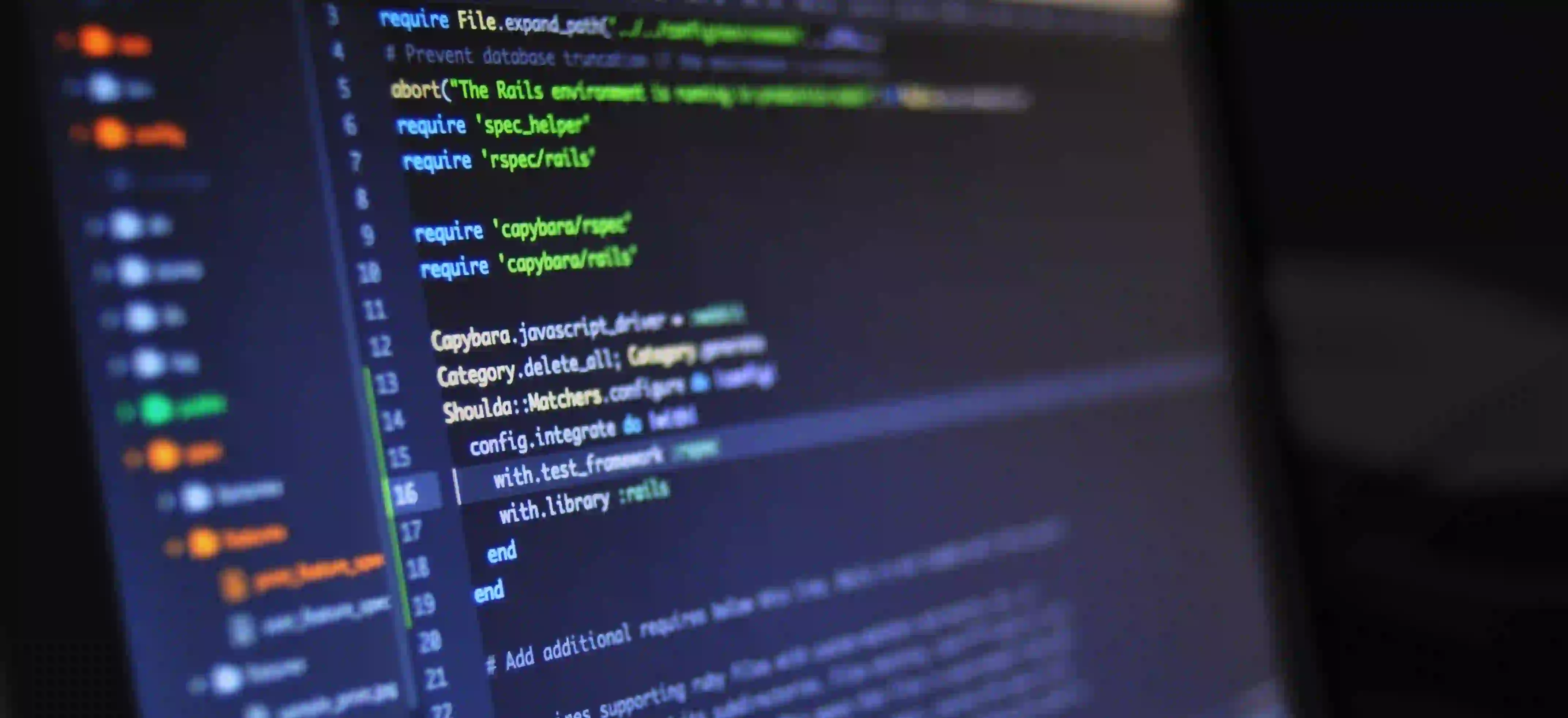
Overcoming Event Handling Complexity in Guava's EventBus
When building applications in Java, particularly those that adopt an event-driven architecture, managing events effectively becomes crucial. Among various libraries, Guava's EventBus
stands out as a powerful tool for simplifying the complexity of event handling. However, leveraging this feature requires a nuanced understanding of its mechanisms, potential pitfalls, and effective strategies for overcoming complexity.
What is Guava's EventBus?
Guava's EventBus
is an event dispatching system that allows for loose coupling between event producers and consumers. It enables publishers to send events without needing to know who will handle them, thus promoting a clean separation of concerns.
Key Features of EventBus
- Loose Coupling: No need for producers to reference consumers directly.
- Type-Safe: Ensures that consumers can subscribe only to events of types they are interested in.
- Easy to Use: Simple API that makes it easy to publish and subscribe to events.
- Asynchronous Processing: Support for asynchronous event handling to improve performance and usability.
Setting up Guava's EventBus
To begin using Guava's EventBus
, you need to include it in your project. If you are using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>31.0.1-jre</version> <!-- Check for the latest version -->
</dependency>
After setting up the dependency, you can start utilizing the EventBus
.
Basic Usage
Let's define a simple event and demonstrate how to use EventBus
to handle this event efficiently.
Step 1: Define an Event
An event can be any class that holds data. Here's a simple example of a UserLoginEvent
class:
public class UserLoginEvent {
private final String username;
public UserLoginEvent(String username) {
this.username = username;
}
public String getUsername() {
return username;
}
}
Step 2: Create a Subscriber
Next, we create a class that will listen for our UserLoginEvent
. We will annotate the subscriber method with @Subscribe
to indicate that it should be called when an UserLoginEvent
is published.
import com.google.common.eventbus.EventBus;
import com.google.common.eventbus.Subscribe;
public class UserLoginListener {
@Subscribe
public void handleUserLogin(UserLoginEvent event) {
System.out.println("User logged in: " + event.getUsername());
}
}
Step 3: Create and Publish Events
Now, you can create an instance of EventBus
, register the listener, and publish events.
public class EventBusExample {
public static void main(String[] args) {
EventBus eventBus = new EventBus();
UserLoginListener listener = new UserLoginListener();
// Register the listener
eventBus.register(listener);
// Publish an event
eventBus.post(new UserLoginEvent("john_doe"));
}
}
Here, when the UserLoginEvent
is published, the handleUserLogin
method gets invoked, demonstrating the decoupled nature of event handling.
Overcoming Complexity in Event Handling
While EventBus
simplifies event handling, developers may still face challenges. Below are some strategies to overcome common complexities.
Understand Thread Safety
One common pitfall when using EventBus
is understanding its threading model. By default, events are dispatched synchronously, meaning the posts block the current thread until all subscribers have processed the event.
In scenarios requiring asynchronous processing, you can create an asynchronous EventBus
like so:
import com.google.common.eventbus.AsyncEventBus;
import java.util.concurrent.Executors;
public class AsyncEventBusExample {
public static void main(String[] args) {
AsyncEventBus asyncEventBus = new AsyncEventBus(Executors.newCachedThreadPool());
UserLoginListener listener = new UserLoginListener();
// Register the listener
asyncEventBus.register(listener);
// Publish an event
asyncEventBus.post(new UserLoginEvent("john_doe"));
}
}
Using an AsyncEventBus
allows your application to continue processing while events are handled, enhancing responsiveness.
Prioritizing Events
In larger applications, you may need a priority system for events. Guava’s EventBus
doesn’t inherently support priorities, but you can achieve this functionality by creating a custom dispatcher. Here’s a simplistic approach:
- Create a wrapper for your events that includes a priority.
- Sort the events by priority before dispatching.
Handling Exceptions Gracefully
Exception handling in an event-driven system can be challenging. An exception thrown in a subscriber will propagate up and can disrupt the event processing flow. You can handle this by employing a try-catch block in your subscriber method:
@Subscribe
public void handleUserLogin(UserLoginEvent event) {
try {
System.out.println("User logged in: " + event.getUsername());
// Simulating exception
if ("john_doe".equals(event.getUsername())) {
throw new RuntimeException("Unexpected login!");
}
} catch (Exception e) {
// Log the exception or handle it accordingly
System.err.println("Error during event processing: " + e.getMessage());
}
}
This approach ensures that one failing subscriber doesn't halt processing for other registered subscribers.
Best Practices
To maximize the effectiveness of Guava's EventBus
, consider the following best practices:
-
Maintain a Single EventBus Instance: Having a singleton instance of
EventBus
promotes shared use across your application. -
Limit Event Payload Size: Keeping events lightweight ensures that your subscribers can handle them quickly.
-
Decouple Processing Logic: Move complex logic out of your subscriber methods to maintain readability and separation of concerns.
-
Document Your Events: Providing clear documentation for each event enhances maintainability and understanding across your development team.
For more in-depth discussions surrounding best practices in event-driven architecture, you might find this resource on Effective JavaScript insightful.
Final Considerations
Guava's EventBus
provides a robust framework for event handling in Java applications, but mastering its use involves understanding its capabilities and limitations. By employing effective strategies, you can simplify the complexity of event-driven programming, creating a maintainable and dynamic architecture. As you build out your Java applications, keep these principles in mind to enhance both functionality and developer experience.
In an ever-evolving landscape where software complexity is on the rise, adopting clean patterns for event handling like Guava's EventBus
will undoubtedly pay dividends in the long run. Happy coding!