Why Java Developers Struggle to Transition to Kotlin
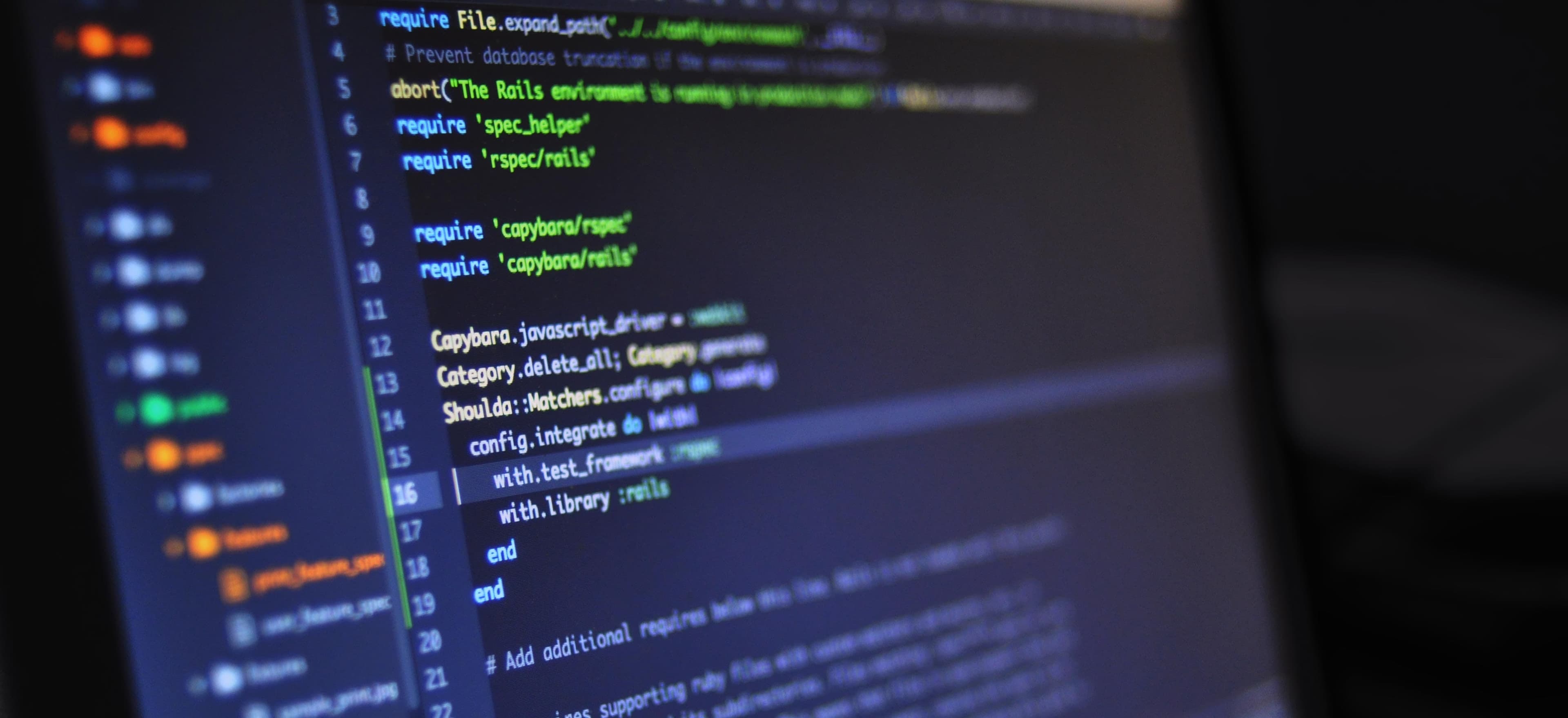
- Published on
Why Java Developers Struggle to Transition to Kotlin
Kotlin has gained remarkable popularity among developers, especially within the Android development community. Its clean syntax, concise code, and full interoperability with Java make it an attractive alternative. However, many Java developers face challenges when transitioning to Kotlin. In this post, we will explore the reasons behind these struggles and offer valuable insights for a smoother transition.
Understanding the Gap: Kotlin vs. Java
Before diving deep into the struggles, it's essential to understand the fundamental differences between Java and Kotlin. Both languages share a common lineage, but they cater to different coding paradigms and principles.
Syntax Differences
Kotlin's syntax is more expressive than Java's. For instance, in Kotlin, you can define a data class in just a line using data class
. In contrast, a similar Java implementation requires boilerplate code.
Kotlin Example:
data class User(val name: String, val age: Int)
Java Equivalent:
public class User {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and Setters
}
The reduced boilerplate in Kotlin may initially seem less intimidating but often leaves Java developers uncertain about the implicit behaviors and features such as data classes, primary constructors, and more.
Null Safety
One of Kotlin's standout features is its null safety. In Java, null references have been a common source of bugs, often leading to NullPointerExceptions
. Kotlin mitigates this issue through nullable and non-nullable types.
Java Example:
String maybeNull = null;
int length = maybeNull.length(); // Throws NullPointerException
Kotlin Equivalent:
val maybeNull: String? = null
val length = maybeNull?.length ?: 0 // Safe call with Elvis operator
The unfamiliarity with managing nullability can confuse Java developers. They may struggle to adjust to Kotlin's type system and syntax, leading to unintended mistakes.
Paradigm Shift: Functional Programming
Kotlin encourages a functional programming approach. For Java developers accustomed to object-oriented programming, this shift can be overwhelming. In Kotlin, you can treat functions as first-class citizens, utilize lambda expressions, and employ collection functions like map
, filter
, and reduce
.
Kotlin Example:
val numbers = listOf(1, 2, 3, 4)
val doubled = numbers.map { it * 2 }
Java Equivalent Using Streams:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4);
List<Integer> doubled = numbers.stream().map(n -> n * 2).collect(Collectors.toList());
While the above code may seem similar, Kotlin's lambda syntax tends to be more concise. Java developers often find themselves grappling with how to leverage these functional features effectively.
Learning Curve and Ecosystem
Transitioning to any new language requires time and effort. Kotlin has a smaller ecosystem compared to Java, which can present a learning curve as developers seek out libraries, frameworks, and community resources. Java's mature ecosystem provides ample resources for guidance, while Kotlin’s documentation, though robust, is comparatively less extensive.
When learning any new programming paradigm, it is crucial to practice regularly. The faster you code in Kotlin, the more comfortable you will become.
Common Pitfalls in Kotlin
There are several pitfalls that Java developers need to be aware of while transitioning to Kotlin.
Default Arguments and Named Parameters
Kotlin supports default arguments and named parameters, which can drastically simplify method signatures.
Kotlin Example with Default Arguments:
fun greet(name: String, greeting: String = "Hello") {
println("$greeting, $name")
}
In Java, you would typically overload methods to achieve similar functionality. Understanding when and how to use these features efficiently can be challenging for new Kotlin developers.
Extension Functions
Kotlin allows you to add new functions to existing classes using extension functions:
Kotlin Example:
fun String.addExclamation(): String {
return this + "!"
}
val excited = "Hello".addExclamation() // "Hello!"
Java developers often need to rethink how they structure their code to accommodate these extensions effectively, deviating from their usual paradigms.
Motivating Transition through Practical Applications
While struggling to learn the ins and outs of Kotlin can be daunting, emphasizing practical applications can ease the transition process. Building simple projects or refactoring existing Java applications into Kotlin can solidify understanding and familiarize developers with the ecosystem.
Example Project: Building a Simple Android App
To illustrate Kotlin in action, let's consider a basic Android application displaying a list of users. Below is a simplified code snippet focused on an Android activity using Kotlin:
class UserActivity : AppCompatActivity() {
private val users = listOf(User("Alice", 25), User("Bob", 30))
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_user)
val userListView: ListView = findViewById(R.id.user_list)
userListView.adapter = ArrayAdapter(this, android.R.layout.simple_list_item_1, users)
}
}
This snippet demonstrates Kotlin’s succinctness when defining a list of users and setting them into a ListView. Notice how the code gets to the point without unnecessary verbosity.
To understand how Kotlin works well in Android, refer to the official Android Developers website.
Wrapping Up: Acclimatization, Practice, and Patience
Transitioning from Java to Kotlin certainly presents challenges. The differences in syntax, null safety mechanisms, functional programming constructs, and ecosystem maturity can initially saturate developers with complexities. However, Kotlin's advantages, such as improved conciseness and modern features, outweigh the hurdles when adequately addressed.
To ease the shift, Java developers should focus on:
- Practical applications: Build small projects in Kotlin.
- Continuous learning: Utilize courses, documentation, and community resources to fill knowledge gaps.
- Emphasizing Kotlin features: Understand and practice unique features to become adept in practical scenarios.
By adopting these strategies, Java developers can navigate their transition to Kotlin more effectively, ultimately unlocking the potential of this modern programming language.
For further reading, check Kotlin Documentation and consider exploring Java-to-Kotlin conversion tools available in popular IDEs like IntelliJ IDEA.
Embrace the journey—Kotlin is waiting for you.
Checkout our other articles