Mastering Data Joins in MapReduce: Common Pitfalls Unveiled
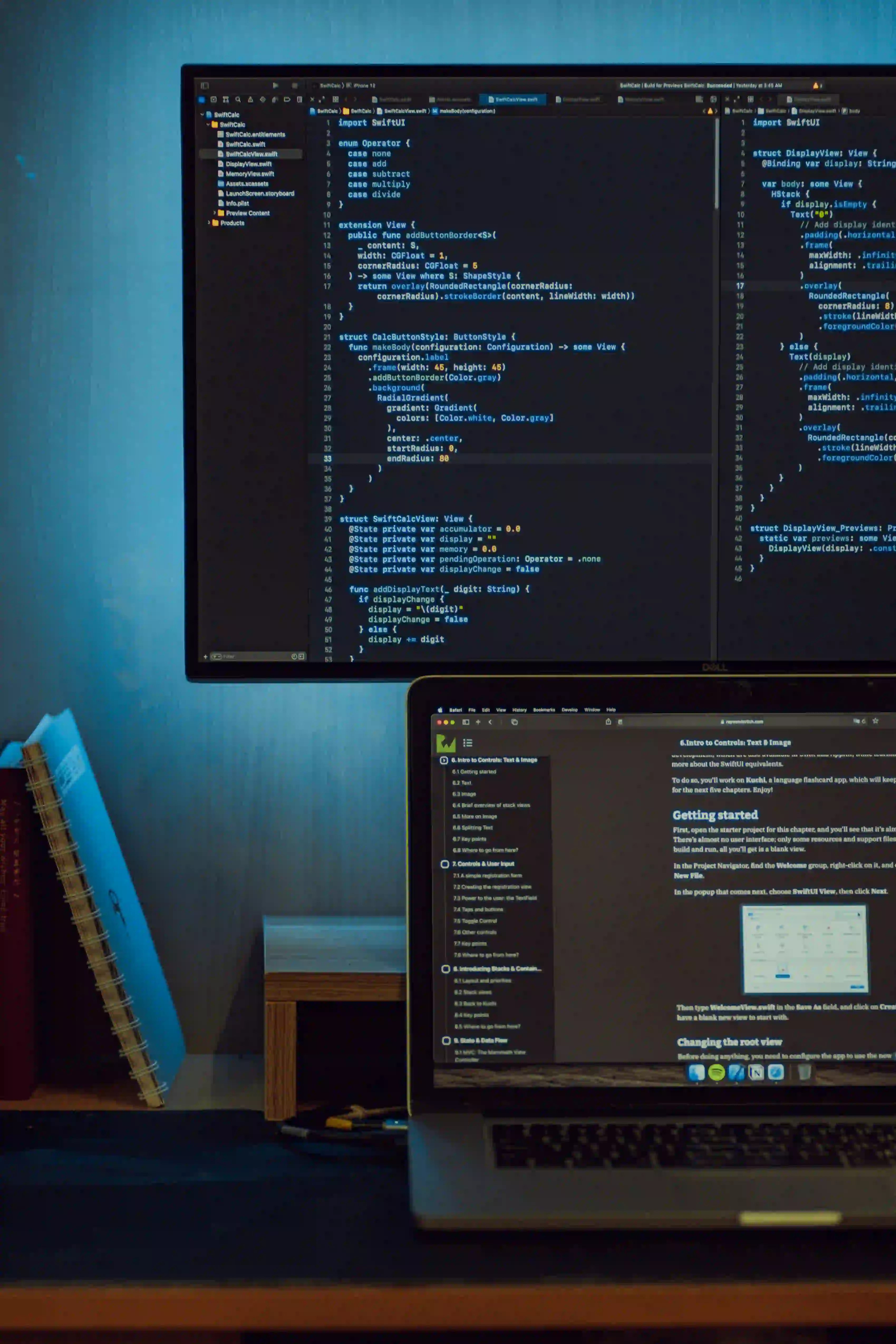
Mastering Data Joins in MapReduce: Common Pitfalls Unveiled
In the world of big data, managing and processing large datasets efficiently is paramount. Among the various technologies that enable this, MapReduce stands out for its scalability and speed. However, as powerful as MapReduce is, it presents unique challenges, particularly when it comes to data joins. In this blog post, we'll delve into common pitfalls associated with data joins in MapReduce and how to master them for more effective data analytics.
Understanding Data Joins in MapReduce
Before we dive into the common pitfalls, let’s take a moment to understand what data joins mean in the context of MapReduce.
A join is a relational operation that combines rows from two or more tables based on a related column. For example, if you have two datasets—a customer dataset and an orders dataset—a join operation can help you combine this information to analyze customer buying behavior.
MapReduce Structure:
- Map Phase: Processes input data, organizing it into key-value pairs.
- Shuffle and Sort: The framework redistributes data based on keys.
- Reduce Phase: Processes the grouped data.
Here's a simple illustration of how a join would look in a MapReduce job.
Example Code: Simple Join
import org.apache.hadoop.io.*;
import org.apache.hadoop.mapreduce.*;
public class JoinExample {
public static class JoinMapper extends Mapper<LongWritable, Text, Text, Text> {
@Override
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] fields = value.toString().split(",");
if (fields.length == 2) {
// Emit customer data
context.write(new Text(fields[0]), new Text("CUSTOMER," + fields[1]));
} else {
// Emit order data
context.write(new Text(fields[0]), new Text("ORDER," + fields[1]));
}
}
}
public static class JoinReducer extends Reducer<Text, Text, Text, Text> {
@Override
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
for (Text val : values) {
context.write(key, val);
}
}
}
}
Why This Code Works
- Versatile Mapper: The mapper differentiates between customer data and order data. This versatility allows us to handle multiple data types efficiently.
- Clear Key-Value Emission: Clearly defining the emitted value types helps the reducer handle them appropriately without confusion.
- Simple Reduce Logic: The reducer’s role is streamlined, outputting all matched records, which simplifies the flow of data processing.
Common Pitfalls in Data Joins
Even expert developers can stumble upon various pitfalls. Let’s explore some of the most common issues along with solutions.
1. Data Skewness
Issue: Data skew occurs when a disproportionate amount of data is associated with a single key. For instance, when many orders belong to a few popular customers, the reducers assigned to those keys can become overwhelmed.
Resolution: Implement a strategy for handling skew, such as salting keys. This involves adding a random number to keys to distribute the load more evenly across reducers.
Example of Salting:
String saltedKey = fields[0] + "_" + new Random().nextInt(10);
context.write(new Text(saltedKey), new Text("CUSTOMER," + fields[1]));
2. Improper Data Handling in the Map Phase
Issue: Incorrect parsing or handling of data can lead to inaccurate joins. Failing to recognize the structure of data can lead to a loss of crucial information.
Resolution: Always validate your data at the map phase. Use checks to ensure that the expected data format adheres to your predefined rules. If any discrepancies occur, log them for later review.
Example of Validation Check:
if (fields.length < 2) {
context.getCounter("JoinJob", "Malformed Records").increment(1);
return; // Skip processing
}
3. Overloading Reducer Logic
Issue: The reducer can quickly become a bottleneck if it is tasked with complex logic. This can lead to performance issues and increased processing time.
Resolution: Simplify the reduce function to focus only on the essential operations. You can perform the detailed processing in a subsequent MapReduce job, thus adhering to the "single responsibility principle".
4. Not Utilizing Combiner Functions
Issue: MapReduce jobs often overlook the potential of a combiner function, which can aggregate results before the shuffle phase.
Resolution: Use a combiner to reduce data volume transmitted over the network. This decreases the amount of data processed in the reduce phase.
Example of Combining:
public static class JoinCombiner extends Reducer<Text, Text, Text, Text> {
@Override
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
// Logic to combine records
}
}
Best Practices for Effective Data Joins
To avoid these common pitfalls, here are some best practices you should follow:
-
Plan Your Data Schema: Understand your data and how it relates. Proper schema design can mitigate issues from the outset.
-
Use Distributed Data Structures: For datasets that don't fit into memory, use Hadoop's distributed data structures like
HFile
orSequenceFile
. -
Perform Local Tests: Before pushing a job to a production cluster, run local tests with smaller data samples. This helps catch issues early.
-
Monitor and Optimize: Utilize monitoring tools such as Apache Ambari or Cloudera Manager to keep an eye on job performance. Identify long-running jobs and analyze their bottlenecks.
-
Documentation is Crucial: Keep documentation for your datasets and join logic. It aids in troubleshooting and helps onboard new team members.
Lessons Learned
Data joins can be complex, but with appropriate strategies to avoid common pitfalls, you can leverage MapReduce effectively for your data analytics needs. As with any technology, mastering the intricacies comes with practice, planning, and a proactive approach to managing your data.
For further reading, you may want to explore Apache Hadoop's official documentation or Data Joins with Hadoop to deepen your understanding and skills.
As you embark on your big data journey, remember: anticipating challenges and strategically planning will lead to more efficient and effective data processing. Happy coding!