Avoiding Big O Pitfalls in Java Code Optimization
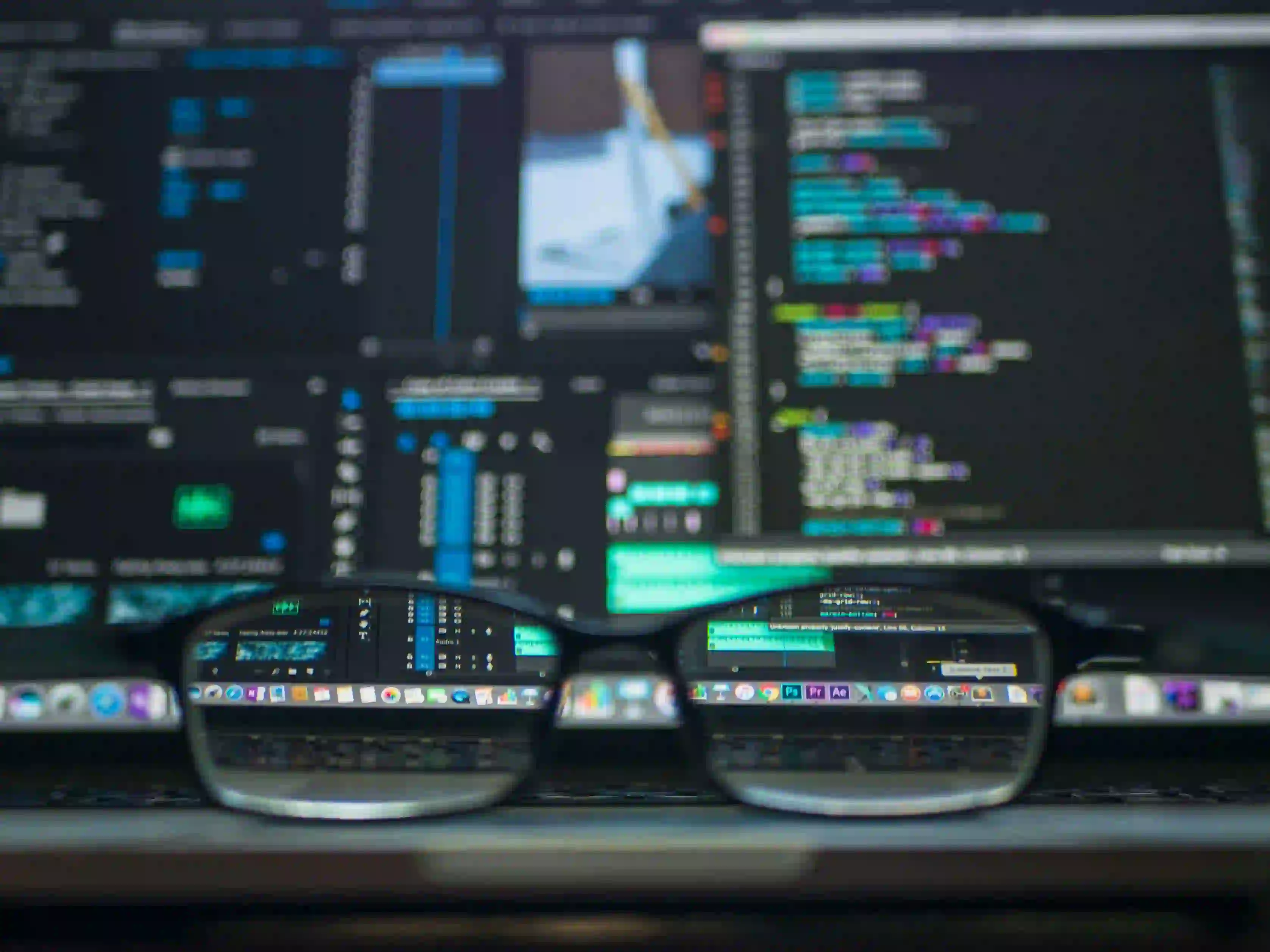
Avoiding Big O Pitfalls in Java Code Optimization
When it comes to writing efficient Java code, understanding algorithmic complexity is crucial. Developers often grapple with Big O notation, a form of mathematical notation that describes the efficiency of algorithms concerning time and space. Mistakes in this area can lead to performance bottlenecks—issues that often arise during code optimization. In this article, we’ll explore how to avoid common Big O pitfalls in Java code, ensuring your applications run efficiently.
For those interested in a deeper dive into Big O notation, I recommend checking out the article titled "Mastering Big O Notation: Common Pitfalls in Interviews" for further insights.
Understanding Big O Notation
Big O notation is a way to express the time complexity of an algorithm, providing a high-level understanding of how the performance will change as the size of the input data grows. The most fundamental classes of Big O include:
- O(1) : Constant time
- O(log n) : Logarithmic time
- O(n) : Linear time
- O(n log n) : Linearithmic time
- O(n^2) : Quadratic time
Understanding these classes will help you make informed decisions when choosing algorithms or data structures for your application.
Common Big O Pitfalls
1. Ignoring Worst-Case Scenarios
One common mistake is failing to consider the worst-case time complexity. Many algorithms exhibit different performance metrics depending on the input. For example, while a binary search has an average case of O(log n), its worst-case scenario can still be O(n) if the input is not properly set up.
public int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid; // Target found
} else if (arr[mid] < target) {
left = mid + 1; // Search on right side
} else {
right = mid - 1; // Search on left side
}
}
return -1; // Target not found
}
In this example, the binary search algorithm generally operates in O(log n) time. However, if the array is not sorted, you'll revert to O(n) when checking each element linearly. Always account for the worst case when optimizing your code.
2. Misunderstanding Nested Loops
Another frequent hurdle is incorrectly assessing the time complexity of nested loops. Developers often assume if the outer loop runs O(n) and the inner loop runs O(n), the overall complexity is linear. In reality, it’s O(n²).
public void nestedLoops(int[] arr) {
for (int i = 0; i < arr.length; i++) { // O(n)
for (int j = 0; j < arr.length; j++) { // O(n)
System.out.println(arr[i] + arr[j]); // O(1)
}
}
}
The above example will indeed run in O(n²) time because the inner loop executes fully for each iteration of the outer loop.
3. Failing to Optimize Data Structures
Choosing the right data structure can drastically affect your algorithm's Big O performance. For instance, implementing a lookup feature with an ArrayList results in O(n) time complexity, whereas using a HashMap allows for O(1).
import java.util.HashMap;
public class Example {
private HashMap<String, Integer> map = new HashMap<>();
public void add(String key, Integer value) {
map.put(key, value); // O(1)
}
public Integer get(String key) {
return map.get(key); // O(1)
}
}
By utilizing a HashMap, both addition and retrieval of elements run in constant time, significantly improving performance in cases of frequent lookups or insertions.
4. Recursive Depth and Performance
Recursion can also introduce complexities in Big O notation. A simple recursive function might not show its true complexity without evaluating how many times it calls itself.
public int factorial(int n) {
if (n == 0) {
return 1;
}
return n * factorial(n - 1);
}
The above function has a time complexity of O(n) because it calls itself n times. However, the space complexity is O(n) as well due to the call stack. Understanding both time and space complexity is vital for optimization.
5. Forgetting to Analyze All Operations
Developers may focus on dominant operations while neglecting other elements influencing performance, such as I/O operations. Always take into account every operation's Big O when calculating the overall complexity.
public void sortAndPrint(int[] arr) {
Arrays.sort(arr); // O(n log n)
for (int num : arr) { // O(n)
System.out.println(num); // O(1)
}
}
The Arrays.sort()
method runs in O(n log n), and the subsequent loop runs in O(n). Therefore, the overall time complexity for this operation is O(n log n), not O(n).
Strategies for Avoiding Pitfalls
Use Profiling Tools
Profiling allows you to identify portions of your code that consume a significant amount of time or resources. Tools like VisualVM can help you measure the performance impact of your code.
Keep Learning
Stay updated about algorithm improvements and explore various data structures beyond arrays and lists. Books, tutorials, and online courses focused on algorithms are valuable resources.
Collaborate and Code Review
Sharing your code with peers or seeking feedback can help identify oversights in performance. Collaboration often brings fresh perspectives on potential optimization opportunities.
Write Modular Code
By breaking down larger algorithms into smaller methods or functions, you can more easily analyze and optimize performance independently. Remember that clean code is not just about aesthetics; it's also about performance.
In Conclusion, Here is What Matters
Optimizing Java code requires a keen understanding of Big O notation to avoid common pitfalls. From recognizing worst-case scenarios to selecting the appropriate data structures, every detail matters. By being mindful of these principles, you can dramatically enhance your application's performance.
For an even deeper exploration into Big O notation and common pitfalls, be sure to check out "Mastering Big O Notation: Common Pitfalls in Interviews". Happy coding!