Optimizing Java Code: Avoiding Big O Pitfalls
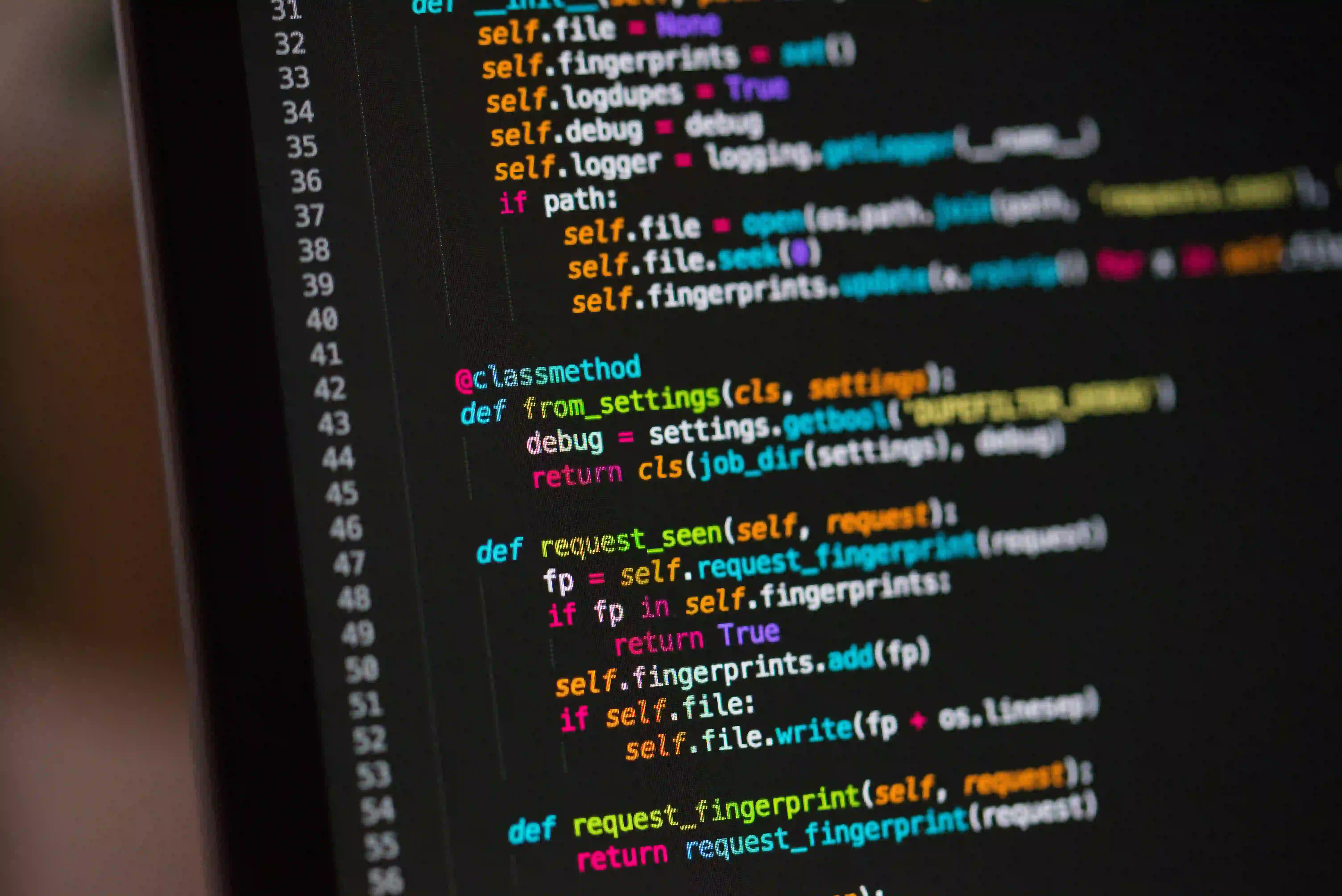
Optimizing Java Code: Avoiding Big O Pitfalls
When it comes to coding in Java or any programming language, efficiency matters. As developers, we often face the challenge of writing code that not only works correctly but also runs efficiently. In this blog post, we will explore how to optimize Java code by avoiding common pitfalls associated with Big O notation, a crucial concept in algorithm analysis.
Before diving in, if you're looking to master Big O notation and its common pitfalls during interviews, I encourage you to check out the article titled "Mastering Big O Notation: Common Pitfalls in Interviews". It provides a comprehensive understanding, which will enrich our discussion here.
Understanding Big O Notation
Big O notation is a mathematical concept used to describe the efficiency of an algorithm. It provides a high-level understanding of the algorithm's performance regarding input size. In simpler terms, Big O gives us an idea of how the time or space complexity of an algorithm grows as the input size increases.
For example, an algorithm with O(1) complexity will execute in constant time regardless of the input size, while O(n) will grow linearly with the input size.
Common Big O Notations
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n^2): Quadratic time
- O(2^n): Exponential time
Understanding these notations will help you analyze your code effectively, ensuring you avoid unnecessary inefficiencies.
Avoiding Big O Pitfalls
While developers may focus on the correctness of their algorithms, they often overlook optimizations that can greatly enhance performance. Here’s how to avoid common pitfalls:
1. Inefficient Data Structures
Using the appropriate data structure is key to improving performance. A common mistake is to choose the wrong data structure for the task at hand, leading to inefficient algorithms with high time complexities.
Example: Array vs. HashMap
Imagine we need to check for duplicates in an array. A naive approach would use a nested loop, yielding O(n^2) complexity.
public boolean hasDuplicate(int[] array) {
for (int i = 0; i < array.length; i++) {
for (int j = i + 1; j < array.length; j++) {
if (array[i] == array[j]) {
return true; // Found a duplicate
}
}
}
return false; // No duplicates
}
The above code repeatedly checks elements against each other. However, we can drastically improve performance to O(n) by using a HashSet
.
import java.util.HashSet;
public boolean hasDuplicate(int[] array) {
HashSet<Integer> seen = new HashSet<>();
for (int num : array) {
if (seen.contains(num)) {
return true; // Found a duplicate
}
seen.add(num); // Add number to HashSet
}
return false; // No duplicates
}
Why This Works: The HashSet
allows for O(1) average-time complexity for checks and insertions, leading to an overall O(n) time complexity for duplicates checks.
2. Nested Loops
While nested loops can sometimes be necessary, their use can lead to inefficiencies, especially with larger datasets. Instead, consider how you can flatten loops or utilize more efficient searching and sorting algorithms.
Example: Flattening Loops
Let’s say we originally had:
public void printCombinations(int[] arr) {
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr.length; j++) {
System.out.println(arr[i] + " " + arr[j]);
}
}
}
The above code has O(n^2) complexity because of the nested loops. You can optimize scenarios like this by using other algorithms or breaking down the processing differently.
3. Unnecessary Calculations
Redundant calculations within loops are a surefire way to increase time complexity unnecessarily. Cache results when possible and assess whether some computations can be moved outside of loops.
Example: Avoiding Redundant Computations
If we need to calculate the sum of squares multiple times within a loop:
public int calculateSumOfSquares(int n) {
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += i * i; // Calculates square each time
}
return sum;
}
Instead, we can pre-compute or utilize a formula where applicable:
public int calculateSumOfSquares(int n) {
return (n * (n + 1) * (2 * n + 1)) / 6; // Use formula for sum of squares
}
Why This Matters: By eliminating the need for a loop entirely, we reduce the time complexity to O(1).
4. Using Streams Efficiently
Java 8 introduced streams that facilitate functional programming. While streams can lead to cleaner code, improper usage can lead to performance pitfalls if not understood clearly—like creating excessive intermediate collections.
Example of Inefficient Stream Use
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> squares = numbers.stream().map(n -> n * n).collect(Collectors.toList());
This can lead to O(n) memory overhead due to intermediate lists. Consider operations that reduce the need for interim storage if your dataset is large.
A Final Look
Optimizing Java code is an essential skill, and it begins with understanding the principles of Big O notation. By avoiding common pitfalls—like utilizing inefficient data structures, nesting loops unnecessarily, executing redundant calculations, and misusing streams—you can write code that performs well for larger datasets.
By implementing the strategies discussed in this article, you will greatly enhance your coding efficiency, making you a more reliable developer. To deepen your understanding of algorithm efficiency and avoid interview pitfalls, revisit the article "Mastering Big O Notation: Common Pitfalls in Interviews".
Remember, code optimization is an ongoing journey. Always look for ways to improve your algorithms while maintaining simplicity and usability. Happy coding!