Unifying DevOps Principles and Practices
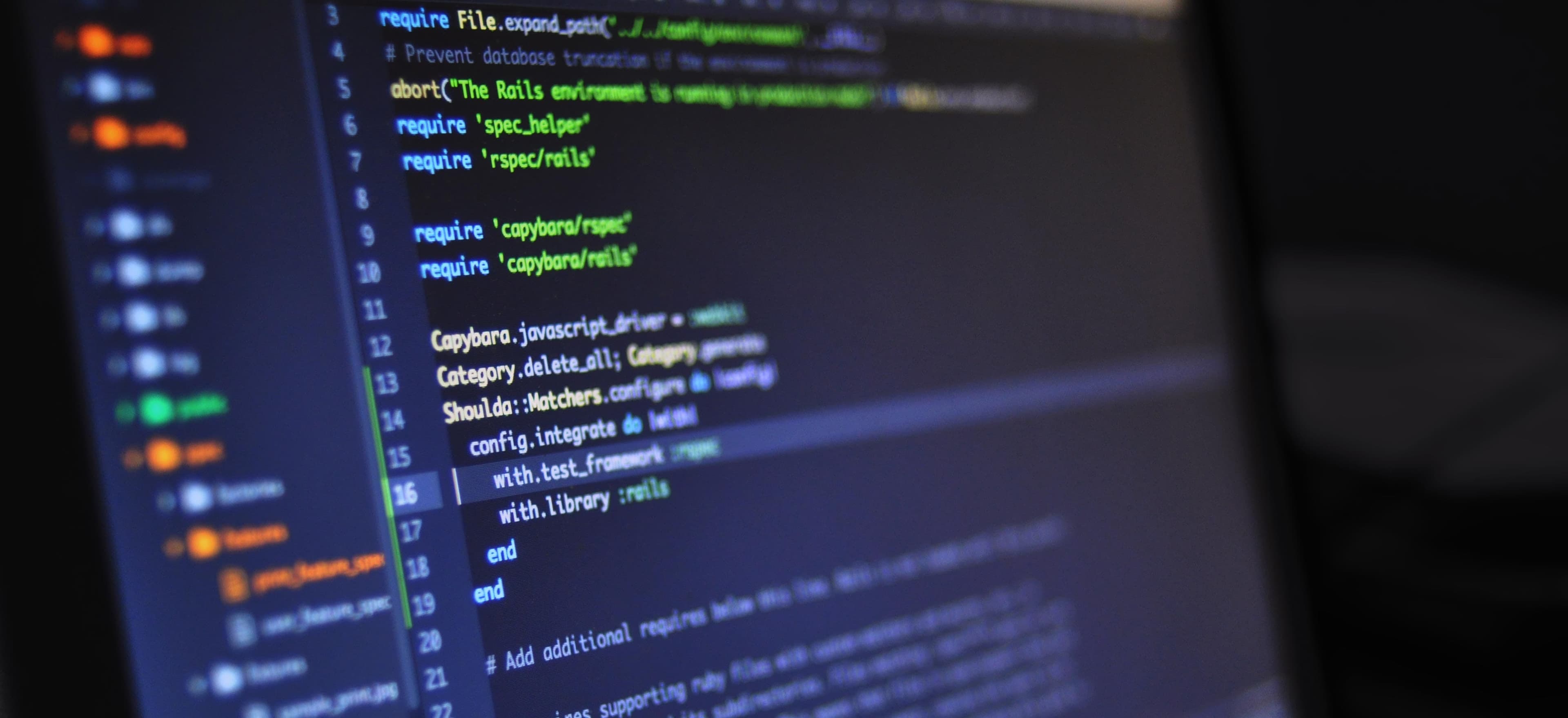
- Published on
Unifying DevOps Principles and Practices
In the contemporary landscape of software development, DevOps has emerged as a critical paradigm shift, breaking down traditional silos between development and operations teams. By fostering collaboration, automation, and continuous integration and delivery, DevOps seeks to streamline the software development lifecycle, enabling greater agility, efficiency, and reliability.
The Role of Java in DevOps
As a versatile and widely used programming language, Java plays a pivotal role in the DevOps ecosystem. Leveraging Java in DevOps practices empowers teams to build robust and scalable applications while embracing automation and continuous improvement.
Let’s delve into some key considerations for integrating Java into DevOps and explore how it can be an asset in achieving seamless, efficient, and reliable software delivery.
1. Continuous Integration with Java
In the realm of DevOps, continuous integration is the cornerstone of streamlining development and delivery processes. Java, with its rich ecosystem of build automation tools like Maven and Gradle, plays a crucial role in enabling seamless continuous integration workflows.
Example of a Maven pom.xml
file for Continuous Integration
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-java-app</artifactId>
<version>1.0.0</version>
<properties>
<!-- Define Java version -->
<java.version>11</java.version>
</properties>
<dependencies>
<!-- Define project dependencies -->
</dependencies>
<build>
<plugins>
<!-- Configure Maven plugins for build automation -->
</plugins>
</build>
</project>
In this example, the pom.xml
file serves as a declarative configuration for a Java project, defining its dependencies, build plugins, and other essential details. By embracing such configuration as code, Java projects can seamlessly integrate into CI/CD pipelines, fostering a culture of frequent, automated testing and integration.
2. Infrastructure as Code with Java
DevOps emphasizes treating infrastructure as code, enabling the automated provisioning and management of infrastructure resources. Java, with its strong object-oriented programming capabilities, can be employed to build and manage infrastructure through tools like Terraform and AWS Cloud Development Kit (CDK), promoting consistency and repeatability.
Example of AWS CDK Infrastructure as Code in Java
import software.amazon.awscdk.core.Stack;
import software.amazon.awscdk.core.Construct;
import software.amazon.awscdk.services.s3.Bucket;
public class MyInfrastructureStack extends Stack {
public MyInfrastructureStack(final Construct scope, final String id) {
super(scope, id);
// Create an S3 bucket
Bucket myBucket = Bucket.Builder.create(this, "MyBucket")
.bucketName("my-unique-bucket-name")
.build();
}
}
In this example, Java is used with AWS CDK to define an S3 bucket as a part of cloud infrastructure. By leveraging Java’s expressive syntax and type safety, infrastructure can be defined, provisioned, and managed with ease, adhering to the principles of infrastructure as code.
3. Monitoring and Logging in Java Applications
Effective monitoring and logging are indispensable in the DevOps landscape, providing visibility and insights into application behavior and performance. Java’s extensive support for logging frameworks like Log4j and SLF4J, coupled with monitoring tools such as Prometheus and Grafana, facilitates robust observability in Java applications.
Example of Logging in a Java Application
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyClass {
private static final Logger LOGGER = LoggerFactory.getLogger(MyClass.class);
public void myMethod() {
LOGGER.debug("This is a debug message");
LOGGER.info("This is an info message");
LOGGER.error("This is an error message");
}
}
By leveraging the above logging example in a Java class, developers can ensure that the application’s behavior and errors are well-captured, contributing to proactive troubleshooting and performance optimization. Furthermore, integrating Java applications with monitoring tools empowers DevOps teams to gain actionable insights into their applications’ health and performance.
A Final Look
In essence, Java serves as a potent enabler in realizing DevOps principles and practices, contributing to the seamless integration, automation, and observability of software development and delivery pipelines. By embracing Java’s prowess in continuous integration, infrastructure as code, and monitoring/logging, DevOps teams can fortify their endeavors towards achieving agility, reliability, and scalability in their software delivery lifecycle.
With its rich ecosystem of libraries, frameworks, and tools, Java remains a formidable force in the realm of DevOps, upholding its status as a language of choice for building and orchestrating modern, resilient, and efficient software systems.
By unifying DevOps principles and practices with Java at the helm, organizations can forge a path towards sustainable innovation and operational excellence in the ever-evolving landscape of software engineering.
We hope this article provides meaningful insights and inspiration for integrating Java into your DevOps endeavors. Happy coding!
Disclaimer: The code examples provided in this article serve illustrative purposes and may require adaptation to specific project requirements and best practices.
References:
- Maven - https://maven.apache.org/
- Gradle - https://gradle.org/
- Terraform - https://www.terraform.io/
- AWS CDK - https://aws.amazon.com/cdk/
- Log4j - https://logging.apache.org/log4j/2.x/
- SLF4J - http://www.slf4j.org/
- Prometheus - https://prometheus.io/
- Grafana - https://grafana.com/