Using Lambdas in Spring MVC 4: Improving Functionality
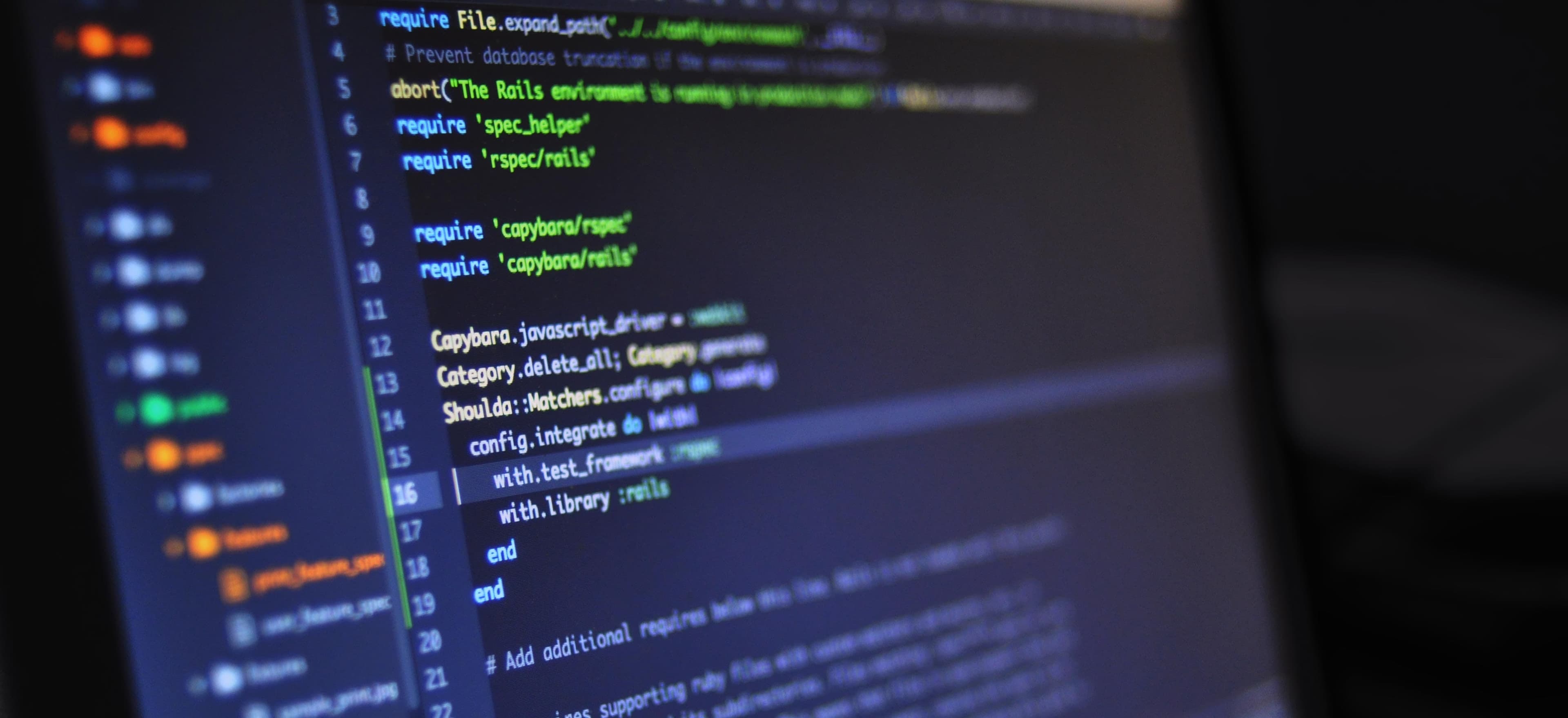
- Published on
Leveraging Lambdas for Enhanced Functionality in Spring MVC 4
When it comes to building robust and scalable web applications, Spring MVC 4 remains a popular choice for Java developers. With its comprehensive features and flexibility, Spring MVC 4 allows for the seamless implementation of various design patterns and programming paradigms. In this article, we will explore how the integration of lambdas, introduced in Java 8, can significantly enhance the functionality and readability of Spring MVC 4 applications.
Understanding Lambdas in Java 8
Lambdas, also referred to as anonymous functions, introduce a concise way to represent a block of code as an expression. This powerful feature allows developers to treat functionality as a method argument or code as data. By leveraging lambdas, developers can enhance the flexibility and maintainability of their codebase, resulting in more readable and efficient programs.
Simplifying Event Handlers with Lambdas
One of the key advantages of using lambdas in Spring MVC 4 is the ability to simplify event handling. Traditionally, event handlers in Spring MVC 4 involve the implementation of interfaces or anonymous inner classes. However, lambdas provide a more streamlined approach to defining event handlers, reducing the verbosity of the code and thus improving readability.
Let's consider a scenario where a button click event needs to be handled in a Spring MVC 4 application. Traditionally, the event handling code might look like this:
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// Handle the button click event
}
});
Integrating lambdas into the event handling process can make the code more concise and expressive:
button.addActionListener(e -> {
// Handle the button click event
});
The use of lambdas not only reduces the boilerplate code but also provides a clearer and more direct representation of the event handling logic.
Leveraging Lambdas in Spring MVC 4
Now, let's explore how lambdas can be integrated into Spring MVC 4 to enhance the overall functionality of the framework.
Using Lambdas for Request Mapping
In Spring MVC 4, request mappings are defined using annotations such as @RequestMapping
. By incorporating lambdas, the process of defining request mappings can be made more succinct and expressive.
Consider the following traditional request mapping:
@RequestMapping(value = "/products/{id}", method = RequestMethod.GET)
public ResponseEntity<Product> getProduct(@PathVariable Long id) {
// Retrieve and return the product details
}
Integrating lambdas into request mapping can simplify the code as follows:
@RequestMapping(value = "/products/{id}", method = RequestMethod.GET)
public ResponseEntity<Product> getProduct(@PathVariable Long id) -> {
// Retrieve and return the product details
}
By utilizing lambdas, the request mapping definition becomes more compact, enhancing the readability and maintainability of the code.
Streamlining Validation with Lambdas
Validation is an integral part of web applications, and Spring MVC 4 provides robust support for data validation through annotations such as @Valid
. The integration of lambdas can further streamline the validation process, making the code more concise and expressive.
Traditionally, validation in Spring MVC 4 might involve code similar to the following:
@RequestMapping(value = "/create", method = RequestMethod.POST)
public String createProduct(@Valid @ModelAttribute Product product, BindingResult result, Model model) {
if (result.hasErrors()) {
// Handle validation errors
}
// Process the validated product
}
With lambdas, the validation code can be refined to enhance its readability:
@RequestMapping(value = "/create", method = RequestMethod.POST)
public String createProduct(@Valid @ModelAttribute Product product, BindingResult result, Model model) -> {
if (result.hasErrors()) {
// Handle validation errors
}
// Process the validated product
}
By employing lambdas, the validation logic becomes more succinct, leading to a cleaner and more expressive codebase.
Closing Remarks
Incorporating lambdas into Spring MVC 4 applications can significantly enhance the functionality and readability of the codebase. By leveraging lambdas for event handling, request mapping, and validation, developers can streamline the implementation of various functionalities, resulting in more concise, expressive, and maintainable code.
As you explore the potential of lambdas in Spring MVC 4, it's essential to consider their impact on code readability and the overall design of the application. With careful integration and thoughtful application, lambdas can serve as a powerful tool for enhancing the capabilities of Spring MVC 4 applications.
In summary, the judicious use of lambdas in Spring MVC 4 can lead to more elegant and efficient code, empowering developers to build robust and scalable web applications with ease.
For further information on integrating lambdas in Spring MVC 4, you can refer to the official Spring Framework documentation. Additionally, exploring advanced Java 8 features, including lambdas, can further enrich your understanding of their application in Spring MVC 4.
Begin leveraging the power of lambdas in Spring MVC 4 today, and elevate the functionality of your web applications with streamlined, expressive code.