Overcoming Flakiness in Continuous Test Automation
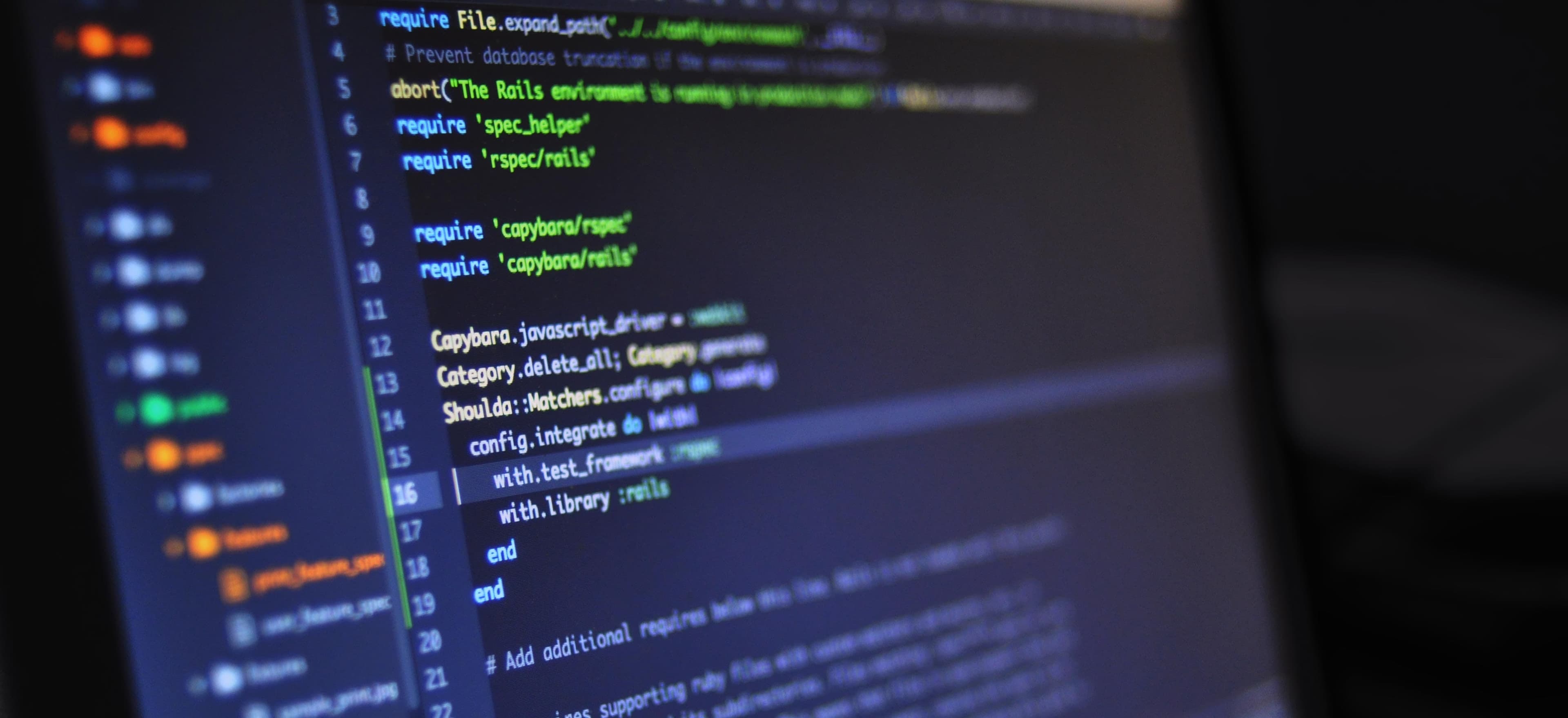
- Published on
Overcoming Flakiness in Continuous Test Automation
Continuous test automation is a crucial part of the software development process, allowing teams to catch bugs early and ensure high-quality code. However, one of the biggest challenges in continuous test automation is dealing with flaky tests. Flaky tests are tests that produce both passing and failing results without any code changes. They are unreliable, erode trust in the test suite, and waste valuable time.
In this post, we will discuss the concept of test flakiness, its impact on continuous test automation, and effective strategies to overcome flakiness in Java-based test automation.
Understanding Test Flakiness
Test flakiness can be caused by various factors such as external dependencies, shared resources, non-deterministic behavior, timing issues, and environmental differences. In Java test automation, flakiness can manifest in different ways, including intermittent failures, race conditions, and non-repeatable failures.
@Test
public void flakyTestExample() {
// Test code with potential for flakiness
}
The above example shows a simple JUnit test method that has the potential to exhibit flakiness due to its reliance on external factors or timing-related issues.
Impact of Flaky Tests in Continuous Test Automation
Flaky tests can significantly impact the effectiveness of continuous test automation. They can lead to the following consequences:
-
Reduced Trust: Flaky tests erode trust in the test suite, leading developers to ignore or bypass failing tests, which defeats the purpose of automated testing.
-
False Alarms: Flaky tests generate false alarms, causing unnecessary interruptions and delays in the development process.
-
Uncertain Test Results: Continuous integration pipelines can become unstable due to flaky tests, making it challenging to determine the true status of the codebase.
Strategies to Overcome Flakiness in Java Test Automation
1. Manage External Dependencies
When writing Java-based automated tests, it's crucial to manage external dependencies effectively. External services, databases, or APIs can introduce flakiness into tests. Consider using mock servers, stubs, or service virtualization to decouple tests from these dependencies.
2. Use Explicit Waits
In Selenium-based UI tests, using explicit waits can mitigate timing-related flakiness. Instead of relying on implicit waits or fixed sleep durations, explicit waits allow tests to wait for specific conditions to be met before proceeding, reducing the probability of timing issues.
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("myElement")));
The above Selenium WebDriver code demonstrates the use of explicit wait to ensure the visibility of an element before interacting with it.
3. Leverage Retry Mechanisms
Implementing retry mechanisms for flaky tests can improve their reliability. In Java, libraries like JUnit and TestNG provide options for retrying failed tests with customizable retry counts and conditions.
@Test(retryAnalyzer = RetryAnalyzer.class)
public void flakyTestWithRetryMechanism() {
// Test code with retry mechanism
}
The annotated test method above utilizes a custom retry analyzer to retry the test in case of failure.
4. Isolate Test Cases
Isolating test cases by minimizing shared resources and ensuring independence can reduce interference between tests, thereby decreasing the likelihood of flakiness.
5. Analyze Test Logs and Artifacts
Regularly analyzing test logs and artifacts can provide insights into the root causes of flakiness. Tools like Log4j and SLF4J in Java can be utilized to enhance logging and facilitate comprehensive analysis.
Bringing It All Together
Continuous test automation is a cornerstone of modern software development, but flaky tests pose a significant challenge to its effectiveness. By understanding the factors contributing to flakiness and implementing robust strategies tailored to Java-based test automation, teams can overcome flakiness and build a trustworthy test suite that accelerates the delivery of high-quality software.
Dealing with flakiness requires a combination of technical solutions, best practices, and a proactive mindset towards test maintenance. By addressing flakiness head-on, teams can ensure that their continuous test automation efforts yield reliable results and contribute to the overall success of the development process.
For more insights on Java test automation best practices, explore Java Testing Best Practices.
Remember, continuous improvement is key to mastering test automation in Java, and overcoming flakiness is a critical step towards achieving that mastery.