Dynamically Adding Properties in Neo4j: A How-To Guide
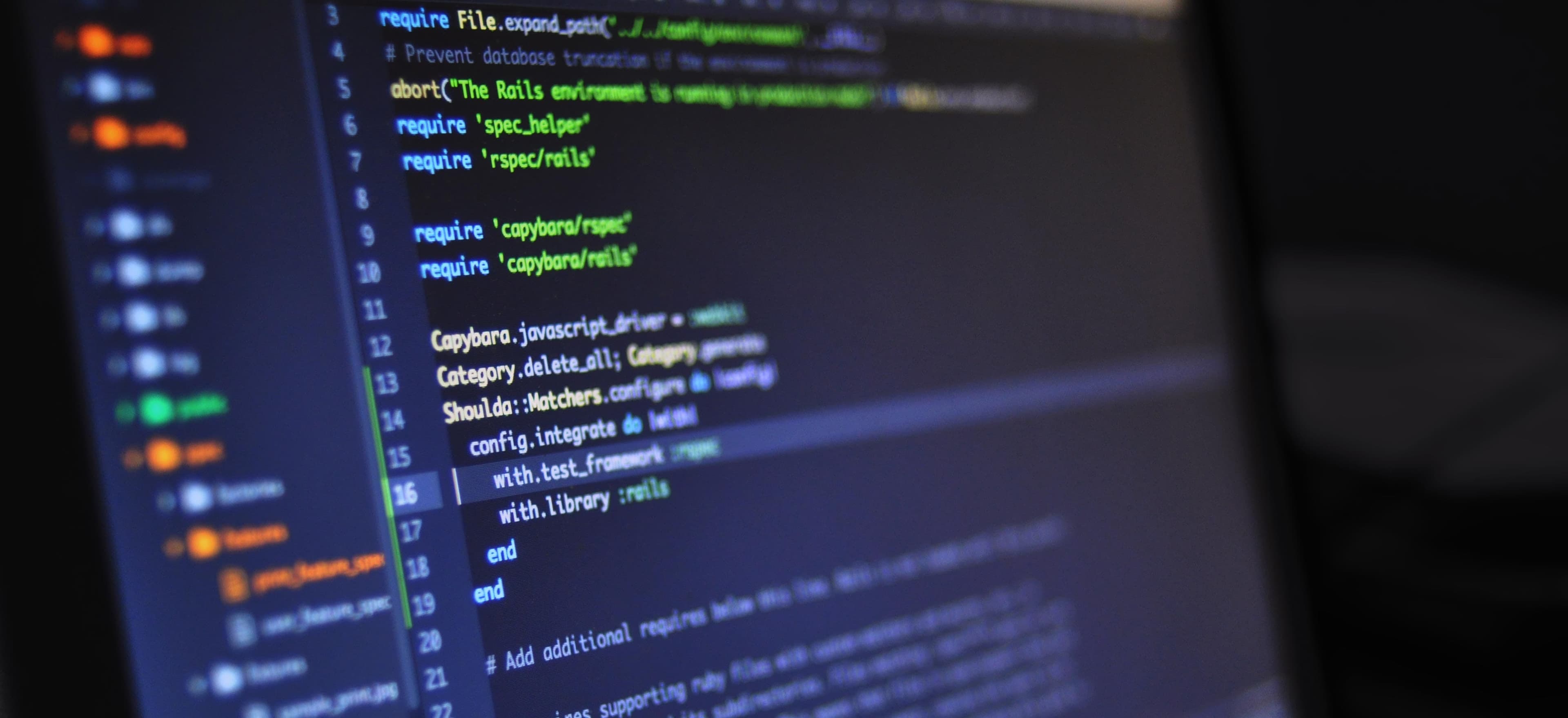
- Published on
Dynamically Adding Properties in Neo4j: A How-To Guide
When working with Neo4j, a popular graph database, it's common to need to dynamically add properties to nodes or relationships. This can be a powerful way to accommodate changing requirements or to handle evolving data structures. In this guide, we'll explore how to dynamically add properties to nodes in Neo4j using Java, and discuss the best practices and considerations.
Why Dynamic Property Addition is Important
In a real-world scenario, data requirements often change over time. For instance, a new feature might require adding new properties to existing nodes without altering the database schema. Dynamically adding properties allows for flexibility to accommodate such changes without disrupting the existing data or requiring schema modifications. This flexibility is particularly valuable in agile development environments, where adaptability is crucial.
Understanding Neo4j's Dynamic Properties
Neo4j, being a schema-free database, allows properties to be added to nodes and relationships at any time without needing to alter the database schema. This approach is particularly beneficial when dealing with unstructured or semi-structured data. In this guide, we'll focus on adding properties to nodes.
Using Java to Add Properties Dynamically
Let's dive into the practical aspect of dynamically adding properties to nodes in Neo4j using Java.
1. Setting up the Neo4j Java Driver
To interact with Neo4j from a Java application, we need to use the Neo4j Java Driver. Start by adding the driver as a dependency in your Maven pom.xml
file:
<dependency>
<groupId>org.neo4j.driver</groupId>
<artifactId>neo4j-java-driver</artifactId>
<version>4.4.1</version>
</dependency>
2. Connecting to the Neo4j Database
Create a new Java class to handle the connection to the Neo4j database:
import org.neo4j.driver.*;
public class Neo4jConnector {
private Driver driver;
public Neo4jConnector(String uri, String username, String password) {
this.driver = GraphDatabase.driver(uri, AuthTokens.basic(username, password));
}
public void close() {
this.driver.close();
}
public Session getSession() {
return this.driver.session();
}
}
3. Adding Properties to Nodes
Now, let's write a method to add properties to nodes in Neo4j. This method takes the node ID, property name, and property value as parameters:
import org.neo4j.driver.*;
public class NodePropertyManager {
private Neo4jConnector connector;
public NodePropertyManager(Neo4jConnector connector) {
this.connector = connector;
}
public void addPropertyToNode(long nodeId, String propertyName, Object propertyValue) {
try (Session session = connector.getSession()) {
String cypherQuery = "MATCH (n) WHERE id(n) = $nodeId SET n += { " + propertyName + ": $propertyValue }";
session.run(cypherQuery, Values.parameters("nodeId", nodeId, "propertyValue", propertyValue));
}
}
}
In this method, we're using a parameterized Cypher query to match the node by its ID and dynamically add the specified property with the provided value.
Best Practices and Considerations
While dynamically adding properties provides flexibility, it's essential to consider the following best practices and considerations:
1. Use Parameterized Queries
Always use parameterized queries to prevent Cypher injection attacks and ensure safe property value assignment.
2. Handle Property Name Validation
Verify and sanitize the property names to prevent any unintended behavior or conflicts.
3. Performance Implications
Be mindful of the performance implications, especially when dealing with a large number of nodes. Dynamically adding numerous properties can impact query performance and should be evaluated accordingly.
4. Semantic Consistency
Maintain semantic consistency when adding properties. Ensure that new properties align with the overall structure and purpose of the data.
Wrapping Up
In this guide, we've explored the importance of dynamically adding properties in Neo4j and demonstrated how to achieve this using Java. By leveraging the Neo4j Java Driver and Cypher queries, we can seamlessly modify node properties without altering the database schema. However, it's crucial to adhere to best practices and considerations to ensure data integrity and query performance.
Dynamic property addition in Neo4j empowers developers to adapt to evolving requirements and handle dynamic data structures effectively, making it a valuable feature in modern application development.
Now that you have a clear understanding of how to dynamically add properties in Neo4j using Java, you can confidently incorporate this practice into your projects and enhance the flexibility and adaptability of your data model.
To delve deeper into Neo4j's capabilities and best practices, check out the Neo4j official documentation. Additionally, for more advanced usage scenarios and community insights, the Neo4j Community Forums serve as a valuable resource. Happy graph modeling!