Optimizing Distributed Computing with Open-Source Java Frameworks
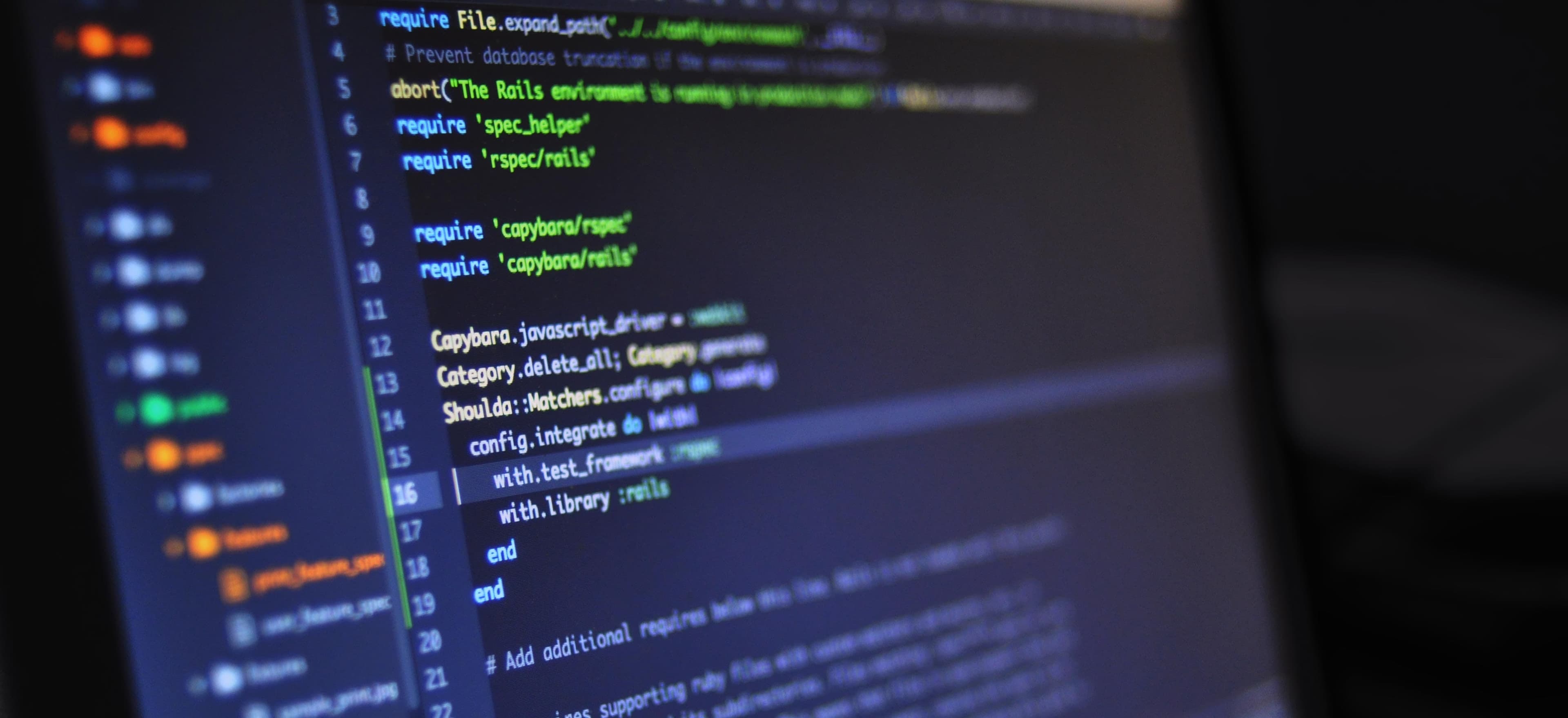
- Published on
Maximizing Distributed Computing Efficiency with Open-Source Java Frameworks
In the realm of distributed computing, the challenge lies in harnessing the power of multiple interconnected machines to perform complex computational tasks. Java, with its robust ecosystem of open-source frameworks, offers a plethora of tools to tackle this challenge effectively. Let's dive into the world of distributed computing with Java and explore how open-source frameworks can optimize this process.
Leveraging the Power of Distributed Computing
Distributed computing involves breaking down a computational task into smaller sub-tasks and distributing them across multiple machines for parallel processing. This approach offers several benefits, including enhanced performance through parallelism, fault tolerance, and the ability to handle large-scale data processing.
The Role of Java in Distributed Computing
Java's platform independence, strong networking capabilities, and vast array of libraries make it an ideal choice for distributed computing tasks. Its ability to seamlessly integrate with various open-source frameworks further solidifies its position in this domain.
Open-Source Java Frameworks for Distributed Computing
Apache Hadoop
Apache Hadoop is one of the most widely used open-source frameworks for distributed computing. It facilitates the distributed processing of large data sets across clusters of computers using a simple programming model. Hadoop's core components, HDFS (Hadoop Distributed File System) and MapReduce, form the backbone of its distributed computing capabilities.
// Example of using Hadoop's MapReduce to process data
public class WordCount {
public static void main(String[] args) throws Exception {
// ...
// Configuration and job setup
// ...
Job job = Job.getInstance(conf, "word count");
// ...
// Mapper and Reducer setup
// ...
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
The above snippet showcases a simple Word Count example using Hadoop's MapReduce, highlighting how Hadoop simplifies the process of distributing computational tasks across a cluster of machines.
Apache Spark
Apache Spark is another popular open-source framework that provides lightning-fast cluster computing. Its in-memory computing capabilities and easy-to-use APIs make it well-suited for a variety of distributed computing tasks, including batch processing, real-time analytics, machine learning, and more.
// Example of using Apache Spark for distributed data processing
public class SparkExample {
public static void main(String[] args) {
// ...
// SparkConf and SparkContext setup
// ...
JavaRDD<String> data = sc.textFile("hdfs://...");
// ...
// RDD transformations and actions
// ...
sc.stop();
}
}
In the above code snippet, we see how Apache Spark simplifies distributed data processing with its concise and expressive APIs, allowing for seamless parallelization of tasks across a cluster.
Akka Toolkit
The Akka Toolkit is a powerful toolkit and runtime for building highly concurrent, distributed, and resilient message-driven applications in Java. It provides abstractions for handling concurrency, distribution, and fault tolerance, making it a valuable asset for developing distributed systems.
// Example of using Akka for building a distributed system
public class AkkaExample {
public static void main(String[] args) {
// ...
// ActorSystem setup
// ...
ActorRef actor = system.actorOf(Props.create(MyActor.class), "myActor");
// ...
// Messaging and coordination among actors
// ...
system.terminate();
}
}
The above code snippet demonstrates how Akka simplifies the process of building distributed systems by abstracting the complexities of concurrency and communication, thereby optimizing the development of distributed applications in Java.
Best Practices for Optimizing Distributed Computing
While leveraging open-source frameworks can significantly enhance the efficiency of distributed computing in Java, it's essential to adhere to best practices to maximize performance and scalability.
Data Partitioning
Effective data partitioning is crucial for distributing data-intensive tasks across a cluster. By partitioning data based on key metrics, such as range-based or hash-based partitioning, we can ensure an even distribution of workload across the nodes in the cluster, thereby preventing bottlenecks and optimizing resource utilization.
Fault Tolerance
In distributed computing, failures are inevitable. Implementing fault-tolerant mechanisms, such as replication, checkpointing, and recovery strategies, is vital to ensure the resilience of the system. Open-source frameworks like Apache Hadoop and Apache Spark provide built-in fault tolerance features, which can be harnessed to minimize the impact of failures on computational tasks.
Resource Management
Effective resource management is essential for optimizing the performance of distributed computing tasks. Leveraging tools like Apache YARN for Hadoop or Apache Mesos for Spark enables dynamic resource allocation and management, thereby maximizing the utilization of cluster resources and improving overall task throughput.
Network Optimization
Minimizing data movement across the network is critical for enhancing the efficiency of distributed computing. Techniques such as data locality optimization, compression, and efficient serialization can significantly reduce network overhead, thereby improving the overall performance of distributed tasks.
Closing Remarks
Distributed computing in Java, powered by open-source frameworks, offers a potent solution for handling complex computational tasks at scale. By leveraging the capabilities of frameworks like Apache Hadoop, Apache Spark, and Akka, and adhering to best practices for optimization, developers can unlock the full potential of distributed computing, achieving unparalleled performance and scalability.
In conclusion, the combination of Java and open-source frameworks presents a compelling proposition for organizations seeking to harness the power of distributed computing, paving the way for groundbreaking innovations in big data processing, real-time analytics, and beyond.
Embracing the world of distributed computing in Java, enriched by these open-source frameworks, holds the promise of a future where complex computational challenges are met with unparalleled efficiency and scalability.
To delve deeper into the world of distributed computing and explore the vast landscape of open-source frameworks in Java, consider checking out Oracle's Java documentation and The Apache Software Foundation.