Optimizing Geospatial Queries in MongoDB
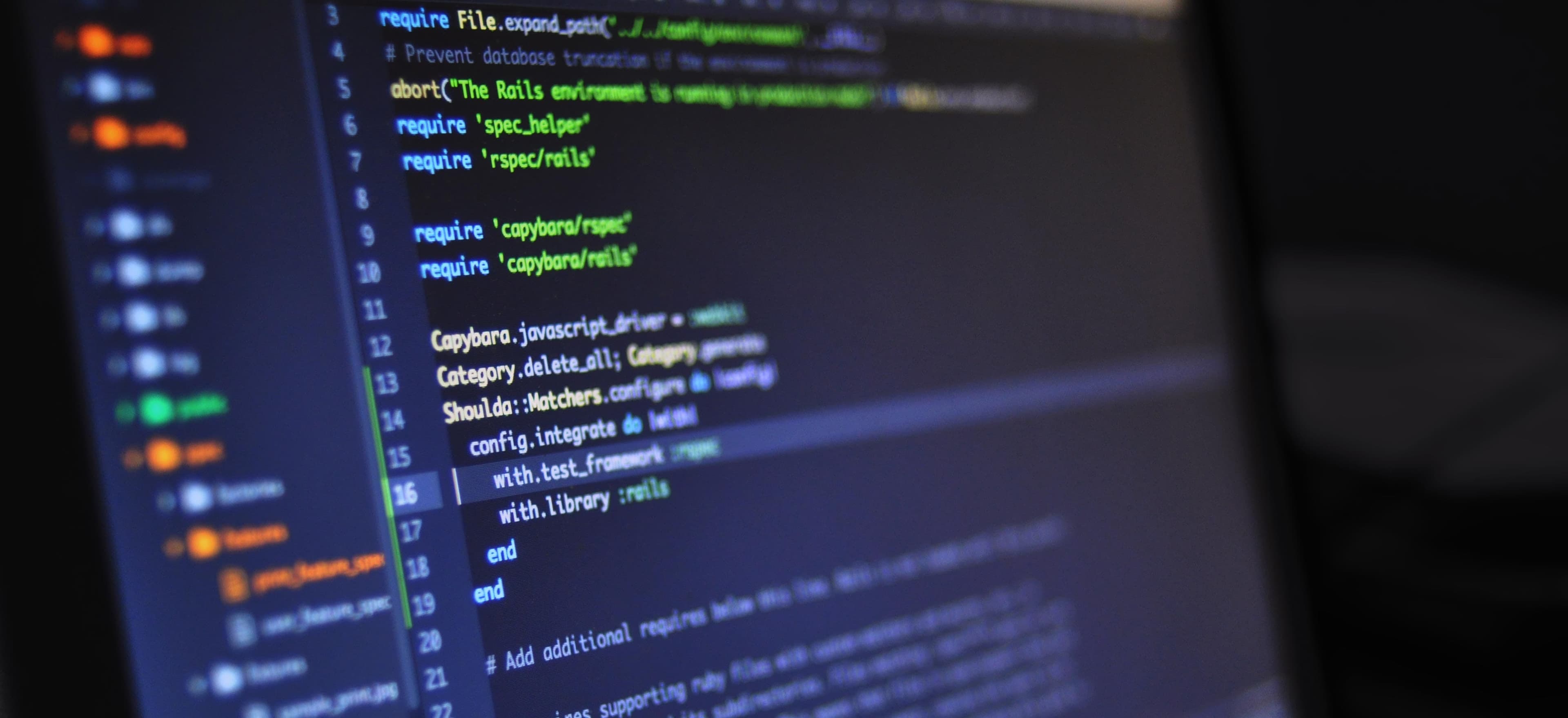
- Published on
Optimizing Geospatial Queries in MongoDB
When working with geospatial data in MongoDB, it's essential to ensure that your queries are optimized for performance. Geospatial queries often involve complex calculations and can be resource-intensive if not handled properly. In this article, we'll explore some best practices for optimizing geospatial queries in MongoDB using Java.
Use Indexes for Geospatial Data
Just like with regular data, indexing plays a crucial role in optimizing geospatial queries. MongoDB supports geospatial indexes, which use geohash-based grid coordinates to optimize the storage and retrieval of geospatial data.
Here's an example of creating a geospatial index on a location
field in a MongoDB collection using the Java driver:
collection.createIndex(Indexes.geo2dsphere("location"));
In this example, we're creating a 2dsphere geospatial index on the location
field of the collection using the geo2dsphere
helper from the MongoDB Java driver.
By using geospatial indexes, MongoDB can efficiently execute geospatial queries and perform proximity-based searches.
Utilize Geospatial Query Operators
MongoDB provides a variety of geospatial query operators that allow you to perform different types of geospatial queries. These operators include $geoWithin
, $geoIntersects
, $near
, and $nearSphere
, among others.
Let's take a look at an example of using the $geoWithin
operator to find documents within a specified polygon:
Bson filter = Filters.geoWithinPolygon("location", polygon);
FindIterable<Document> documents = collection.find(filter);
In this example, we're using the geoWithinPolygon
method from the MongoDB Java driver to construct a query filter that finds documents within the specified polygon.
By leveraging these geospatial query operators, you can precisely tailor your geospatial queries to retrieve the exact data you need, which can significantly improve query performance.
Leverage Aggregation Framework for Geospatial Analysis
The MongoDB Aggregation Framework provides powerful tools for performing advanced analytics and data processing, including geospatial analysis. By using the Aggregation Framework, you can manipulate and process geospatial data in sophisticated ways, which can be beneficial for optimizing complex geospatial queries.
Here's an example of using the Aggregation Framework to perform a geospatial aggregation:
List<Bson> pipeline = Arrays.asList(
Aggregates.nearSphere("location", new Point(new BsonDouble(-73.9667), new BsonDouble(40.78)), 1000, 0)
);
AggregateIterable<Document> results = collection.aggregate(pipeline);
In this example, we're using the nearSphere
aggregation stage to find documents near a specific geographical point within a specified distance.
By incorporating the Aggregation Framework into your geospatial query optimization strategy, you can take advantage of its flexibility and performance optimizations for complex geospatial data processing.
Efficiently Retrieve and Analyze Geospatial Data
In geospatial applications, it's crucial to retrieve and analyze geospatial data efficiently. This involves fetching only the necessary data and performing the required calculations as optimally as possible.
When retrieving geospatial data from MongoDB, consider using projection to limit the fields returned in the query results. By retrieving only the essential fields, you can reduce the amount of data transferred over the network and improve query performance.
Here's an example of how to apply projection to a geospatial query in MongoDB using the Java driver:
Bson projection = Projections.include("name", "location");
FindIterable<Document> documents = collection.find().projection(projection);
In this example, we're using the include
method from the MongoDB Java driver to specify the fields "name"
and "location"
for inclusion in the query results.
Additionally, when performing calculations or analysis on geospatial data, consider leveraging MongoDB's geospatial functions and operators to offload computational tasks to the database server. This can reduce the overhead on the application side and improve overall query performance.
Key Takeaways
Optimizing geospatial queries in MongoDB using Java involves leveraging geospatial indexes, utilizing geospatial query operators, harnessing the power of the Aggregation Framework, and efficiently retrieving and analyzing geospatial data. By following these best practices and incorporating them into your Java-based MongoDB applications, you can ensure that your geospatial queries are optimized for performance and deliver the best possible user experience.
In conclusion, by implementing these strategies, you can ensure that your Java-based MongoDB applications perform optimally when dealing with geospatial data.
For further information on geospatial indexing in MongoDB, you can refer to the official MongoDB documentation on Geospatial Indexes.
Remember, ensuring that your geospatial queries are optimized not only improves performance but also provides a more seamless experience for your application users.