Optimizing Performance with Java Collections
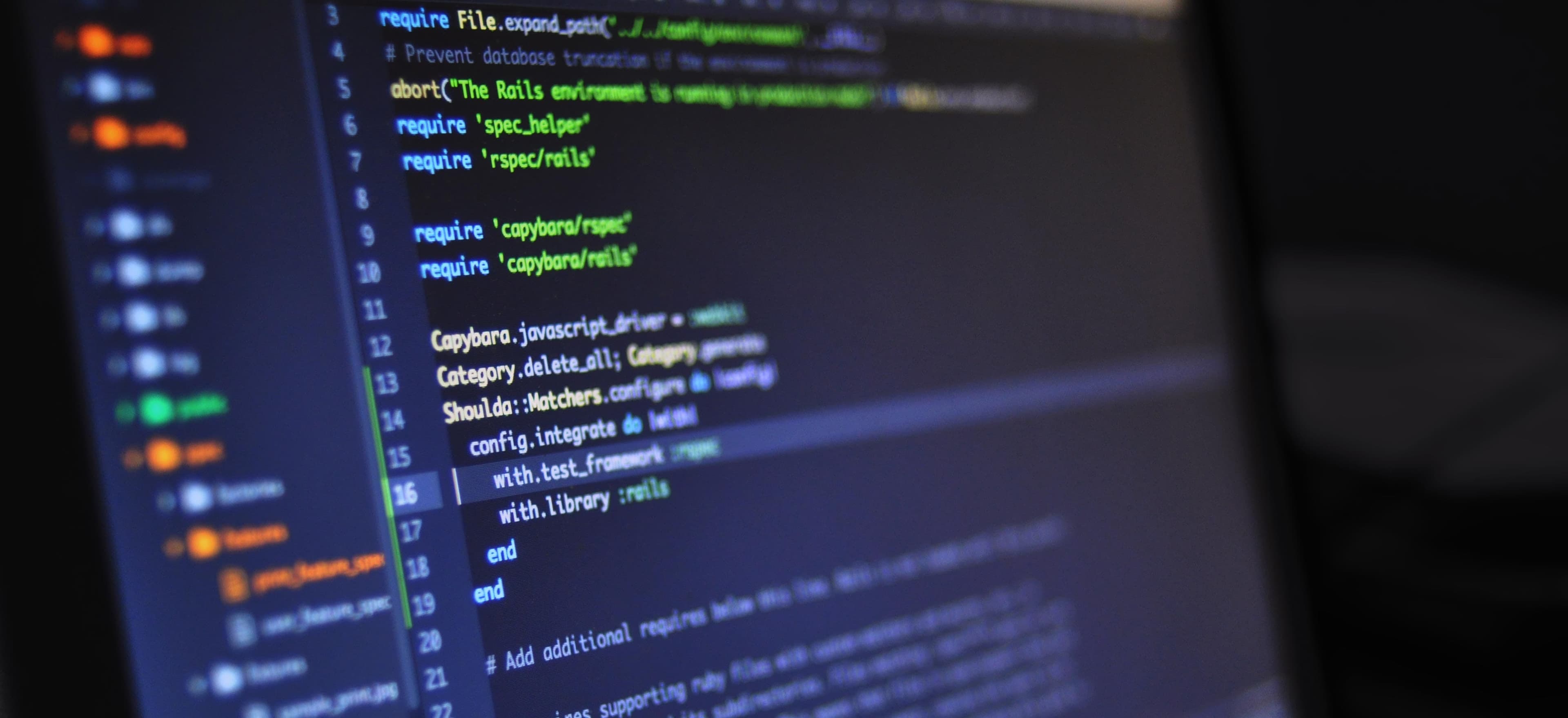
- Published on
Optimizing Performance with Java Collections
When it comes to optimizing the performance of Java applications, efficient data structures are crucial. Java Collections framework provides a rich set of interfaces and classes to store, retrieve, and manipulate collections of objects. In this article, we will explore how to optimize the performance of Java applications using various collection classes and techniques.
Choose the Right Collection
The choice of collection class has a significant impact on the performance of an application. Different collection classes are designed for different use cases. For example:
- Use
ArrayList
when frequent access of elements by index is required. - Use
LinkedList
when frequent insertions and deletions are needed. - Use
HashMap
for fast retrieval of elements based on keys. - Use
HashSet
for unique element storage without duplicates.
Choosing the right collection class ensures that the operations performed on the collection are efficient and optimized for the specific use case.
Use Proper Initial Capacity
When creating a collection, it's important to provide an initial capacity if the number of elements to be stored is known in advance. For example, when creating an ArrayList
, specifying the initial capacity prevents the need for frequent resizing of the underlying array as elements are added.
List<String> names = new ArrayList<>(1000); // Initial capacity of 1000
By providing the initial capacity, unnecessary overhead due to resizing can be avoided, leading to improved performance.
Use Enhanced For Loop
When iterating over collections, using the enhanced for loop introduced in Java 5 provides a more concise and readable code. Moreover, it is optimized under the hood and can potentially offer better performance compared to traditional for
loops.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
for (int number : numbers) {
System.out.println(number);
}
The enhanced for loop simplifies the iteration process and can lead to improved performance in certain scenarios.
Be Wary of Autoboxing
Autoboxing, the automatic conversion of primitive types to their corresponding object wrapper classes, can impact performance when working with collections. For example, using ArrayList<Integer>
instead of ArrayList<int>
can lead to unnecessary object creation and memory overhead due to autoboxing.
List<Integer> numList = new ArrayList<>();
for (int i = 0; i < 10; i++) {
numList.add(i); // Autoboxing of 'int' to 'Integer'
}
In scenarios where performance is critical, using primitive types directly can be more efficient than relying on autoboxing.
Use Immutable Collections
Java provides immutable collection classes such as Collections.unmodifiableList
, Collections.unmodifiableSet
, and Collections.unmodifiableMap
to create read-only views of existing collections. Immutable collections offer benefits in terms of thread safety and can be more efficient in certain use cases due to their predictable and unchangeable nature.
List<String> immutableList = Collections.unmodifiableList(Arrays.asList("apple", "banana", "cherry"));
By using immutable collections, unnecessary modifications and synchronization overhead can be avoided, leading to improved performance in multi-threaded environments.
Consider Parallel Streams
In Java 8 and later, the introduction of parallel streams allows for parallel processing of collections, potentially improving performance for computationally intensive operations on large datasets. However, it's important to carefully evaluate whether parallel processing offers actual performance gains, as it introduces overhead related to thread management and synchronization.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
int sum = numbers.parallelStream().mapToInt(Integer::intValue).sum();
System.out.println("Sum: " + sum);
In scenarios where the operations performed on the collection are suitable for parallel processing, parallel streams can lead to performance improvements.
Use Custom Comparators
When working with sorted collections like TreeMap
or TreeSet
, providing custom comparators can enhance performance by allowing fine-grained control over the sorting order. By defining custom comparators, complex sorting logic can be implemented, leading to optimized performance for specific sorting requirements.
Map<String, Integer> studMarks = new TreeMap<>((s1, s2) -> s2.compareTo(s1));
studMarks.put("Alice", 85);
studMarks.put("Bob", 92);
studMarks.put("Charlie", 78);
In scenarios where default sorting order does not suffice, custom comparators enable efficient sorting based on specific criteria.
Use Primitives in Specialized Collections
Java provides specialized collections such as IntArrayList
, LongHashSet
, and DoubleLinkedOpenHashSet
in the org.eclipse.collections
library to work with primitive types directly, avoiding autoboxing overhead and reducing memory footprint.
MutableIntList intList = IntLists.mutable.with(1, 2, 3, 4, 5);
int sum = intList.sum();
System.out.println("Sum: " + sum);
Specialized collections for primitive types can offer improved performance and reduced memory overhead compared to using standard collections with wrapper classes for primitives.
Efficiently Handle Large Data Sets
When dealing with large data sets, it's important to choose the appropriate collection classes and algorithms to ensure optimal performance. Data structures like LinkedHashMap
or LinkedHashSet
can be beneficial in scenarios where predictable iteration order is required, as they maintain the order of insertion.
LinkedHashMap<String, Integer> accessOrderMap = new LinkedHashMap<>(16, 0.75f, true);
accessOrderMap.put("Monday", 1);
accessOrderMap.put("Tuesday", 2);
accessOrderMap.put("Wednesday", 3);
By selecting the right collection classes and data structures, the performance of applications dealing with large data sets can be significantly improved.
Closing the Chapter
Optimizing performance with Java collections involves making informed choices about collection classes, iteration techniques, and handling large data sets efficiently. By leveraging the appropriate collection classes, considering performance implications of autoboxing, utilizing parallel streams when beneficial, and using specialized collections for primitive types, developers can ensure that their Java applications exhibit optimized performance characteristics.
In conclusion, understanding the nuances of Java collections and employing best practices can lead to significant improvements in application performance and efficiency.
References:
- Java Documentation - Collections Framework
- Java Magazine - Choosing the Right Collection
- Baeldung - Java Collections Performance
- Eclipse Collections