Migrating from Java 6/8 to JPMS with Gradle
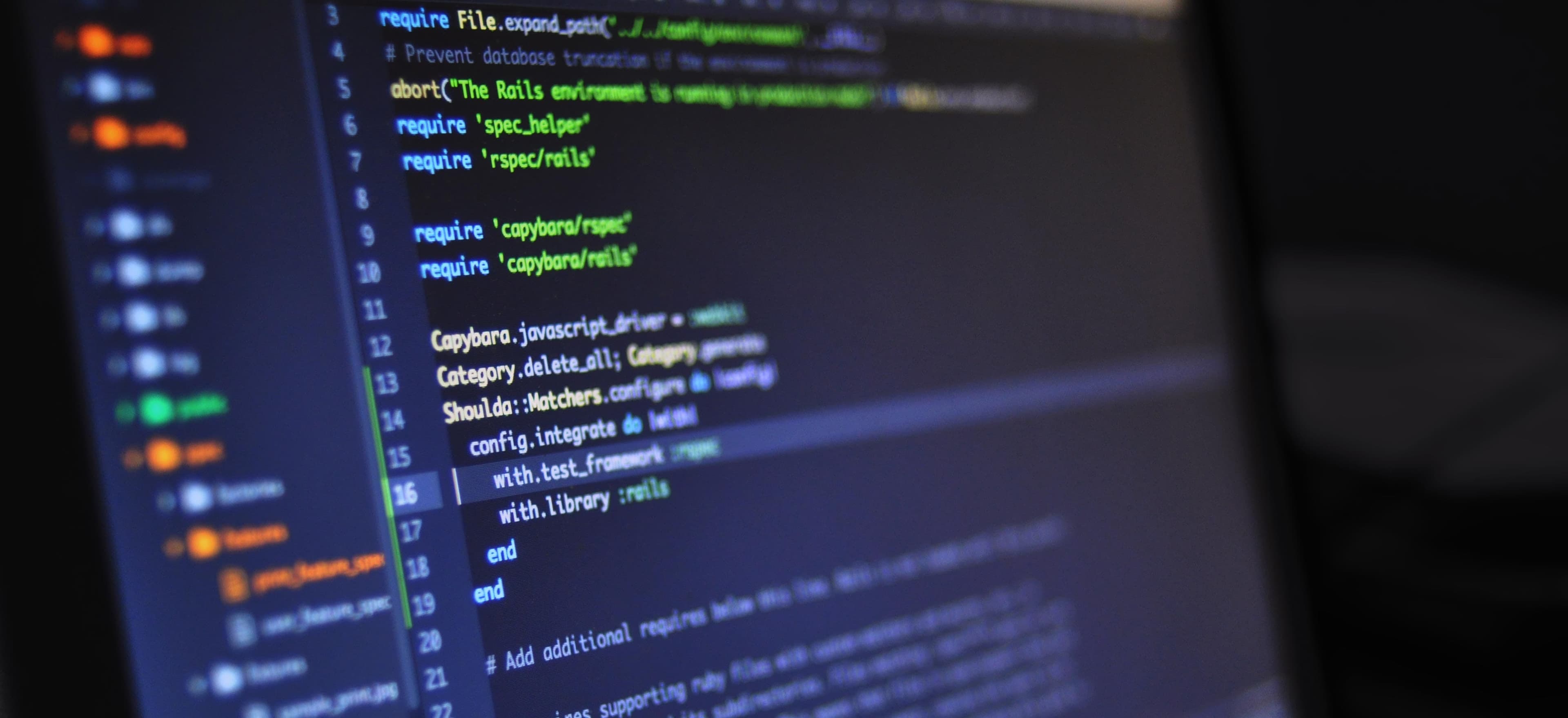
- Published on
Migrating from Java 6/8 to JPMS with Gradle
In this article, we'll explore the process of migrating a Java 6/8 project to Java Platform Module System (JPMS) using Gradle. The Java Platform Module System, introduced in Java 9, provides a modular structure for Java applications, improving maintainability, performance, and security. We'll discuss the steps involved in this migration and provide code examples to illustrate each step.
Why Migrate to JPMS?
Migrating to JPMS offers several benefits:
- Modularity: JPMS allows breaking down a large monolithic codebase into smaller, manageable modules, enabling better organization and reusability.
- Encapsulation: With modules, you can control the visibility of your code, reducing dependencies and potential conflicts.
- Security: JPMS provides a more secure environment by encapsulating internal implementation details.
- Performance: Modules can specify their dependencies, leading to optimized runtime performance.
Preparing the Gradle Project
We begin by preparing the Gradle project for the migration. Ensure you have the latest version of Gradle installed. Update your build.gradle
file to use the Java 9 plugin and specify the Java version to be targeted:
plugins {
id 'java'
}
java {
toolchain {
languageVersion = JavaLanguageVersion.of(9)
}
}
The java
block specifies the language version to be used. This configuration prepares the project to be built with Java 9.
Modularizing the Project
The next step involves modularizing the project. In the source code, create a module-info.java file for each module to define its boundaries and dependencies. Let's consider a simple example where we have a module called com.example.app
:
module com.example.app {
requires java.base;
exports com.example.app;
}
In this module descriptor, we specify that the module com.example.app
requires the java.base
module and exports the package com.example.app
.
Resolving Dependencies
With JPMS, you can no longer rely on the classpath for dependencies. You need to explicitly specify module dependencies and ensure that all required modules are accessible. For external libraries, you can create automatic modules or use existing modular artifacts.
dependencies {
implementation 'org.slf4j:slf4j-api:1.7.30'
implementation 'org.apache.commons:commons-lang3:3.10'
}
In the dependencies
block, we declare dependencies using the implementation
configuration. The specified artifacts will be treated as automatic modules, allowing them to be used in the JPMS environment.
Building and Running the Modularized Project
After modularizing the project and resolving dependencies, you can build and run the modularized application. Use Gradle tasks to compile, package, and execute the modularized project:
gradle clean build
java -p build/libs -m com.example.app/com.example.app.Main
The java
command specifies the module path (-p
) and the main module (-m
) with the qualified name of the module and the main class.
Handling Reflection and Deprecated Features
JPMS introduces restrictions on reflective access and deprecates certain features such as the sun.misc
package. If your codebase relies heavily on reflection or uses deprecated features, you'll need to refactor and adapt your code to comply with JPMS requirements. Consider using the --add-exports
and --add-opens
options to open packages for reflective access when migrating legacy code.
Testing and Migration Verification
It's crucial to thoroughly test the modularized application to ensure that the migration has been successful. This includes testing for correct module resolution, encapsulation, and compatibility with third-party libraries. Verify that the application behaves as expected and that all module boundaries are respected.
To Wrap Things Up
Migrating from Java 6/8 to JPMS with Gradle involves several steps, including preparing the project, modularizing the codebase, resolving dependencies, building the modularized project, addressing reflection and deprecated features, and testing the migration. By migrating to JPMS, you can take advantage of modularity, encapsulation, improved security, and enhanced performance.
Migrating to JPMS may seem daunting, especially for large, legacy codebases, but the long-term benefits outweigh the initial effort. Gradle's support for JPMS simplifies the migration process, making it more manageable.
In conclusion, embracing JPMS with Gradle sets the stage for a more modular, maintainable, and secure Java application. As you embark on this migration journey, leverage tools, documentation, and community resources to ensure a smooth transition to JPMS.
By adhering to these steps, you can seamlessly migrate your Java 6/8 project to JPMS, unlocking the potential of modularity and setting the stage for a more robust and efficient application.
Remember, as you navigate the migration process, stay informed about best practices and keep an eye on the evolving landscape of Java development.
Migrating to JPMS represents a pivotal step forward in embracing the future-ready, modular architecture for your Java applications.
Happy migrating!
Learn more about JPMS and Gradle's support for Java modules.
Happy coding!