Improving Interactivity in Hypermedia REST APIs
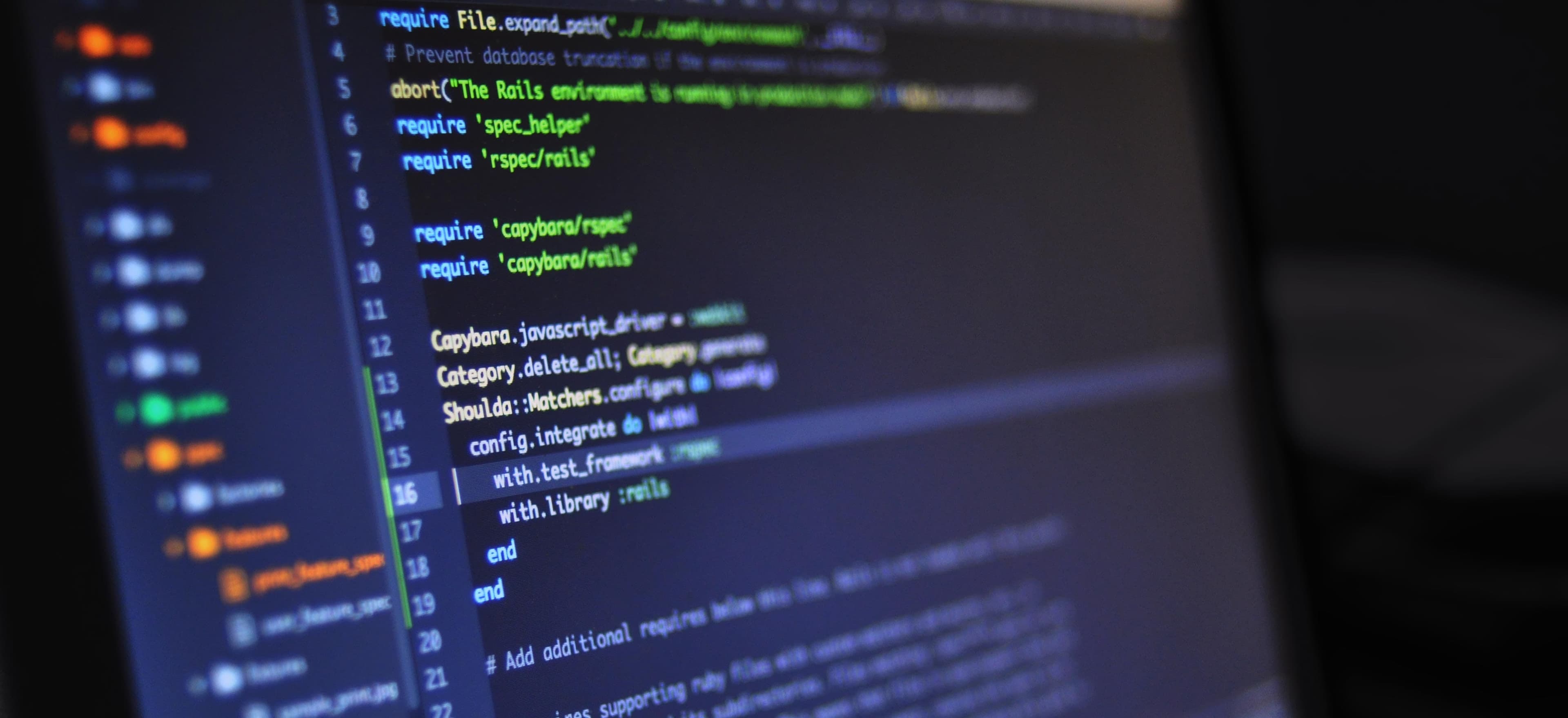
- Published on
Enhancing Interactivity in Hypermedia REST APIs
As developers, we're constantly seeking ways to improve the interactivity of the applications and APIs we create. In the world of REST APIs, hypermedia plays a crucial role in achieving this goal. Hypermedia is the engine of application state (HATEOAS), and it allows clients to navigate through the API dynamically by following links and actions provided by the server.
In this blog post, we'll explore how to enhance the interactivity of Hypermedia REST APIs using Java. We'll look at practical techniques and best practices to make our APIs more intuitive, flexible, and adaptive.
Understanding Hypermedia REST APIs
At its core, a Hypermedia REST API is designed to utilize hypermedia controls to drive the application state. It provides clients with dynamically navigable resources by embedding links and actions in the API responses. This eliminates the need for clients to have prior knowledge of the API's URL structure, and instead, allows them to discover and interact with resources based on the hypermedia controls provided by the server.
Leveraging Hypermedia Controls - The Importance of HATEOAS
One of the key principles of Hypermedia REST APIs is HATEOAS (Hypermedia as the Engine of Application State). HATEOAS enables clients to interact with the API without relying on fixed URLs. By including hypermedia controls such as links and actions in API responses, clients can dynamically navigate through the application state, making the API more flexible and adaptable to changes.
Integrating Hypermedia Support in Java
In Java, there are several frameworks and libraries that facilitate the creation of Hypermedia REST APIs. One popular choice is Spring HATEOAS, which provides a set of abstractions and utilities for creating hypermedia-driven REST APIs.
Let's dive into a practical example to illustrate the integration of hypermedia support using Spring HATEOAS in a Java application.
import org.springframework.hateoas.EntityModel;
import org.springframework.hateoas.server.RepresentationModelAssembler;
import static org.springframework.hateoas.server.mvc.WebMvcLinkBuilder.*;
public class ProductModelAssembler implements RepresentationModelAssembler<Product, EntityModel<Product>> {
@Override
public EntityModel<Product> toModel(Product product) {
return EntityModel.of(product,
linkTo(methodOn(ProductController.class).getProduct(product.getId())).withSelfRel(),
linkTo(methodOn(ProductController.class).getAllProducts()).withRel("products"));
}
}
In the code snippet above, we define a ProductModelAssembler
that implements the RepresentationModelAssembler
interface provided by Spring HATEOAS. This assembler is responsible for converting a Product
entity into a hypermedia representation (EntityModel<Product>
) that includes relevant links.
The toModel
method populates the representation with links such as self
and products
using linkTo
and methodOn
methods from WebMvcLinkBuilder
. These links enable clients to navigate to the specific product and the collection of all products, thereby enhancing the interactivity of the API.
Designing Discoverable APIs with Hypermedia Controls
When designing Hypermedia REST APIs, it's essential to focus on providing discoverable resources with embedded hypermedia controls. This approach empowers clients to traverse the API dynamically without hardcoded URLs, promoting a more adaptable and intuitive API interaction.
Let's consider a scenario where we have an endpoint for retrieving a specific product and want to embed hypermedia controls to enable clients to navigate related resources.
@RestController
@RequestMapping("/products")
public class ProductController {
private final ProductService productService;
private final ProductModelAssembler productModelAssembler;
@Autowired
public ProductController(ProductService productService, ProductModelAssembler productModelAssembler) {
this.productService = productService;
this.productModelAssembler = productModelAssembler;
}
@GetMapping("/{id}")
public EntityModel<Product> getProduct(@PathVariable Long id) {
Product product = productService.getProductById(id);
return productModelAssembler.toModel(product);
}
}
In the ProductController
class, the getProduct
method now returns an EntityModel<Product>
that includes hypermedia controls. By utilizing the ProductModelAssembler
to build the hypermedia representation, the API response becomes enriched with navigable links, allowing clients to explore related resources.
Best Practices for Interactivity in Hypermedia REST APIs
To ensure the effective enhancement of interactivity in Hypermedia REST APIs, here are some best practices to consider:
-
Consistent Use of Hypermedia Controls: Embed hypermedia controls consistently in API responses to guide clients through the application state.
-
Resource Linking: Provide links to related resources to assist clients in navigating the API seamlessly.
-
Avoid Hardcoded URLs: Eliminate hardcoded URLs in API clients by relying on hypermedia controls for resource discovery.
-
Dynamic Navigation: Enable dynamic navigation by offering hypermedia controls that reflect the current application state and available actions.
The Last Word
In conclusion, leveraging hypermedia in REST APIs is paramount for enhancing interactivity and adaptability. By embracing HATEOAS principles and integrating hypermedia support in Java applications, developers can empower clients to dynamically navigate through the API, leading to a more intuitive and flexible API interaction.
When designing Hypermedia REST APIs, the focus should be on providing discoverable resources with embedded hypermedia controls, thereby enabling clients to interact with the API in a more dynamic and adaptive manner.
By implementing best practices and utilizing frameworks such as Spring HATEOAS, developers can elevate the interactivity of their Hypermedia REST APIs, creating a more seamless and enjoyable experience for API consumers.
By following these guidelines and embracing the power of hypermedia, we can build REST APIs that truly excel in interactivity and adaptability.
For more information on building Hypermedia REST APIs with Java, check out the Spring HATEOAS documentation. Additionally, you can explore the concepts of hypermedia and HATEOAS in depth through resources such as the Richardson Maturity Model.