Optimizing Jenkins Setup for Kubernetes and Docker
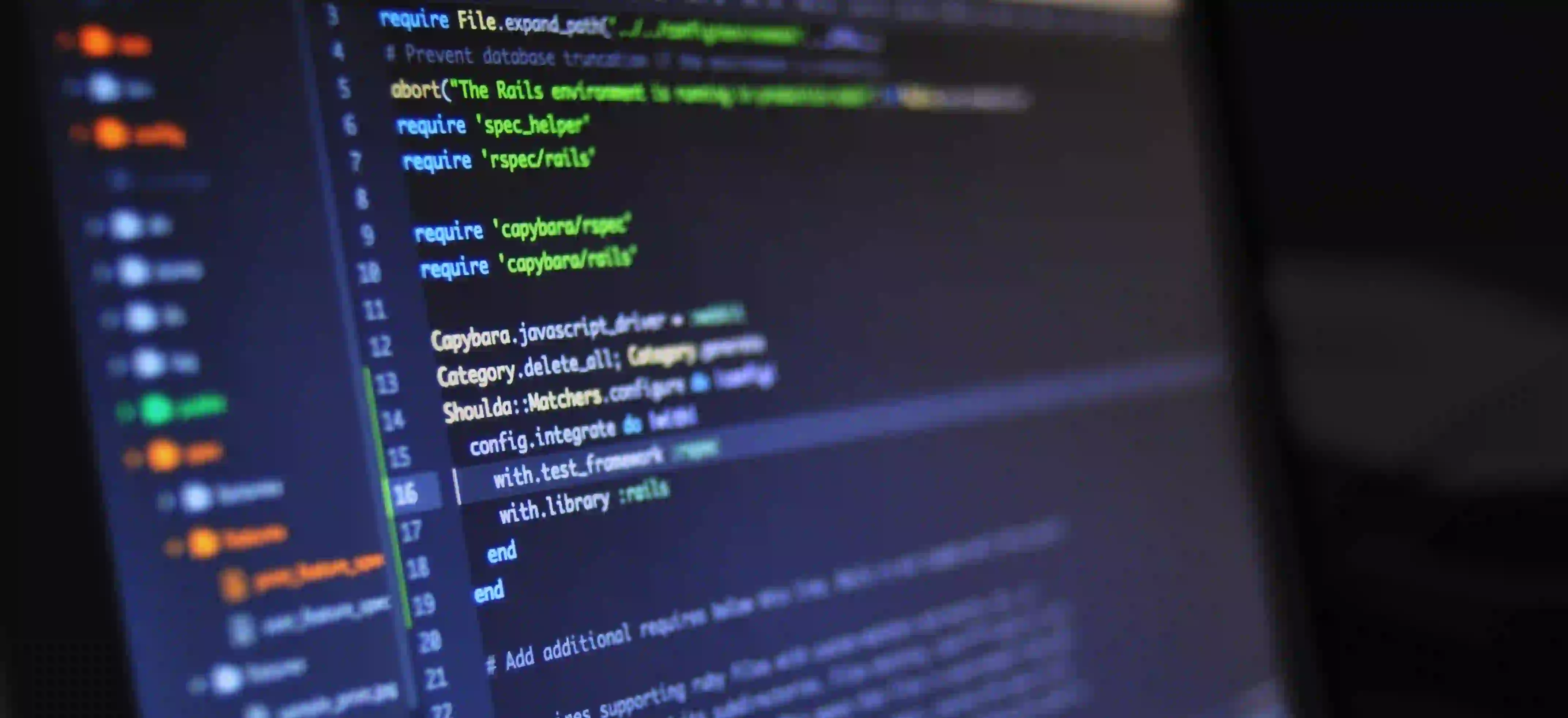
Optimizing Jenkins Setup for Kubernetes and Docker
In the world of continuous integration and continuous deployment (CI/CD), Jenkins remains a popular choice for automating the software delivery process. With the rise of containerization and orchestration technologies like Kubernetes and Docker, optimizing Jenkins setup for these tools has become essential. In this blog post, we'll explore some best practices for optimizing a Jenkins setup for Kubernetes and Docker, ensuring seamless integration and efficient usage of these technologies.
Understanding the Basics
Before delving into optimization techniques, let's ensure a clear understanding of the basic concepts and interactions between Jenkins, Kubernetes, and Docker.
Jenkins
Jenkins is an open-source automation server that enables the automation of building, testing, and deploying software. It accomplishes this through a robust ecosystem of plugins, making it highly customizable and extensible.
Kubernetes
Kubernetes is a popular container orchestration platform that automates the deployment, scaling, and management of containerized applications. It allows for seamless management of containerized workloads and services.
Docker
Docker is a leading containerization platform that enables developers to encapsulate applications and dependencies into containers for easy deployment and portability across environments.
Optimizing Jenkins for Kubernetes and Docker
Now, let's explore some best practices for optimizing Jenkins setup for Kubernetes and Docker.
1. Utilize Jenkins Kubernetes Plugin
The Jenkins Kubernetes plugin allows Jenkins agents to be dynamically provisioned on a Kubernetes cluster. This enables efficient utilization of resources by creating Jenkins build agents as Kubernetes pods.
Example usage of Jenkins Kubernetes Plugin:
agent {
kubernetes {
label 'my-custom-label'
defaultContainer 'jnlp'
yaml """
apiVersion: v1
kind: Pod
metadata:
labels:
some-label: some-label-value
"""
}
}
In the above example, a Jenkins agent is provisioned as a Kubernetes pod based on a custom label, demonstrating the dynamic provisioning capability enabled by the plugin.
2. Containerize Jenkins Build Environment
By containerizing the Jenkins build environment using Docker, you can achieve consistency across different build agents and environments. This approach ensures that the build environment is isolated and reproducible, leading to more reliable builds.
Example Dockerfile for Jenkins build environment:
FROM jenkins/jnlp-slave
# Install necessary tools and dependencies
RUN apt-get update && apt-get install -y <your-dependencies>
The above Dockerfile showcases the process of creating a custom Jenkins build agent image with specific tools and dependencies installed, thus encapsulating the build environment within a container.
3. Implement Jenkins Pipeline for Containerized Builds
Jenkins Pipeline, also known as Jenkinsfile, allows you to define the entire build process as code. When optimizing for Docker, Jenkins Pipeline can be leveraged to define and execute containerized builds, making the build process more transparent and version-controlled.
Example Jenkins Pipeline for containerized builds:
pipeline {
agent {
docker { image 'your-custom-build-image' }
}
stages {
stage('Build') {
steps {
sh 'your-build-command'
}
}
// Additional stages for testing, deployment, etc.
}
}
In the above Jenkins Pipeline example, the entire build process is encapsulated within a Docker container, ensuring consistency and reproducibility across build agents and environments.
4. Enable Docker within Jenkins Agents
To support Docker commands within Jenkins build agents, you can configure Jenkins to communicate with the Docker daemon. This enables build pipelines to perform Docker-related operations, such as building and pushing Docker images.
Example Jenkins agent configuration for Docker support:
agent {
docker {
image 'docker'
args '-v /var/run/docker.sock:/var/run/docker.sock'
}
}
By enabling Docker within Jenkins agents, you provide the capability to interact with Docker directly from the build pipeline, facilitating Docker image builds and other Docker-related tasks.
5. Use Kubernetes for Jenkins Master High Availability
To ensure high availability of the Jenkins master, Kubernetes can be leveraged to deploy Jenkins in a highly available configuration. This involves using Kubernetes features such as StatefulSets or Helm charts for managing Jenkins master components.
Example StatefulSet for Jenkins master deployment:
apiVersion: apps/v1
kind: StatefulSet
metadata:
name: jenkins-master
labels:
app: jenkins
spec:
serviceName: jenkins
replicas: 3
// Other StatefulSet configurations
In the above example, a StatefulSet is used to deploy Jenkins master with multiple replicas, ensuring fault tolerance and high availability.
Key Takeaways
Optimizing Jenkins setup for Kubernetes and Docker is crucial for modern CI/CD practices. By leveraging the capabilities of Kubernetes and Docker, Jenkins can be empowered to efficiently build, test, and deploy containerized applications. From dynamic agent provisioning to containerized build environments, these optimization techniques pave the way for a seamless integration between Jenkins, Kubernetes, and Docker, ultimately enhancing the automation and reliability of software delivery pipelines.