Bytes Loss during Data Conversion
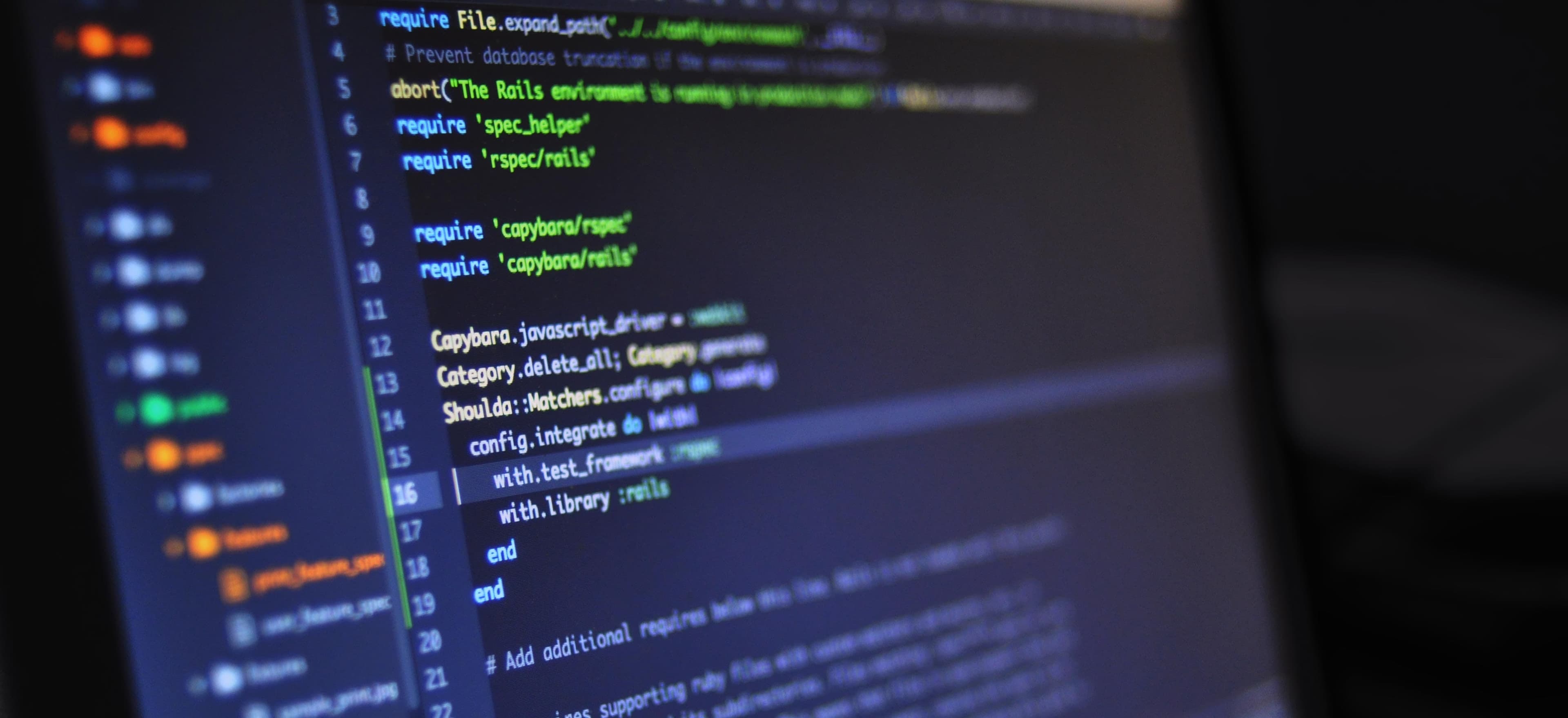
- Published on
Understanding Byte Loss during Data Conversion in Java
In the world of Java programming, data conversion forms a crucial aspect of working with different data types. Whether you're converting integers to strings or bytes to characters, it's essential to understand that loss of data can occur in certain scenarios. This blog post aims to explore the concept of byte loss during data conversion in Java, shedding light on the potential pitfalls and best practices to minimize such loss.
What is Byte Loss?
In Java, data conversion involves converting a value from one data type to another, potentially leading to the loss of precision or information. When converting data to a smaller data type, such as from int
to byte
, byte loss can occur if the value being converted is too large to be represented by the target data type.
Example of Byte Loss in Java
int largeNumber = 300;
byte convertedByte = (byte) largeNumber;
System.out.println(convertedByte); // Output: 44
In this example, the value of largeNumber
exceeds the range that can be represented by a byte (-128 to 127). As a result, byte loss occurs, leading to an unexpected value when largeNumber
is converted to a byte.
Handling Byte Loss with Java's Data Types
To handle byte loss during data conversion, it's important to understand the range and limitations of different data types in Java. For example, the byte
data type has a range of -128 to 127, while the int
data type has a much larger range. When converting from a larger data type to a smaller one, it's crucial to ensure that the value being converted falls within the range of the target data type to avoid byte loss.
Using Casting in Java to Control Data Conversion
In Java, casting is commonly used to explicitly convert a value from one data type to another. When dealing with potential byte loss, casting can be used to control the data conversion process and handle any loss of information.
int largeNumber = 300;
byte convertedByte = (byte) largeNumber;
System.out.println(convertedByte); // Output: 44
In this example, the (byte)
cast is used to explicitly convert the int
value to a byte
, despite the potential byte loss. By using casting, developers can take control of the data conversion process and explicitly handle any loss that may occur.
Best Practices to Avoid Byte Loss
To minimize the risk of byte loss during data conversion, consider the following best practices:
-
Understand Data Type Ranges: Familiarize yourself with the range of each data type in Java to ensure that values being converted fall within the acceptable range to avoid byte loss.
-
Use Conditional Checks: Before performing data conversion, consider using conditional checks to validate whether the value being converted exceeds the range of the target data type.
-
Handle Edge Cases: Pay special attention to edge cases where a value is near the limits of the range of the target data type, as these scenarios are more prone to byte loss.
Wrapping Up
In conclusion, byte loss during data conversion in Java is a concept that developers should be mindful of when working with different data types. By understanding the range of data types, using casting effectively, and following best practices, it's possible to minimize the risk of byte loss and ensure accurate data conversion in Java.
To delve further into the intricacies of data conversion and handling byte loss in Java, consider exploring Oracle's official documentation on data types and conversions. Additionally, experimenting with different scenarios and edge cases can provide valuable insights into how byte loss can be mitigated in practical Java programming.