Managing Compatibility Issues with Multiple Oracle JDK Versions
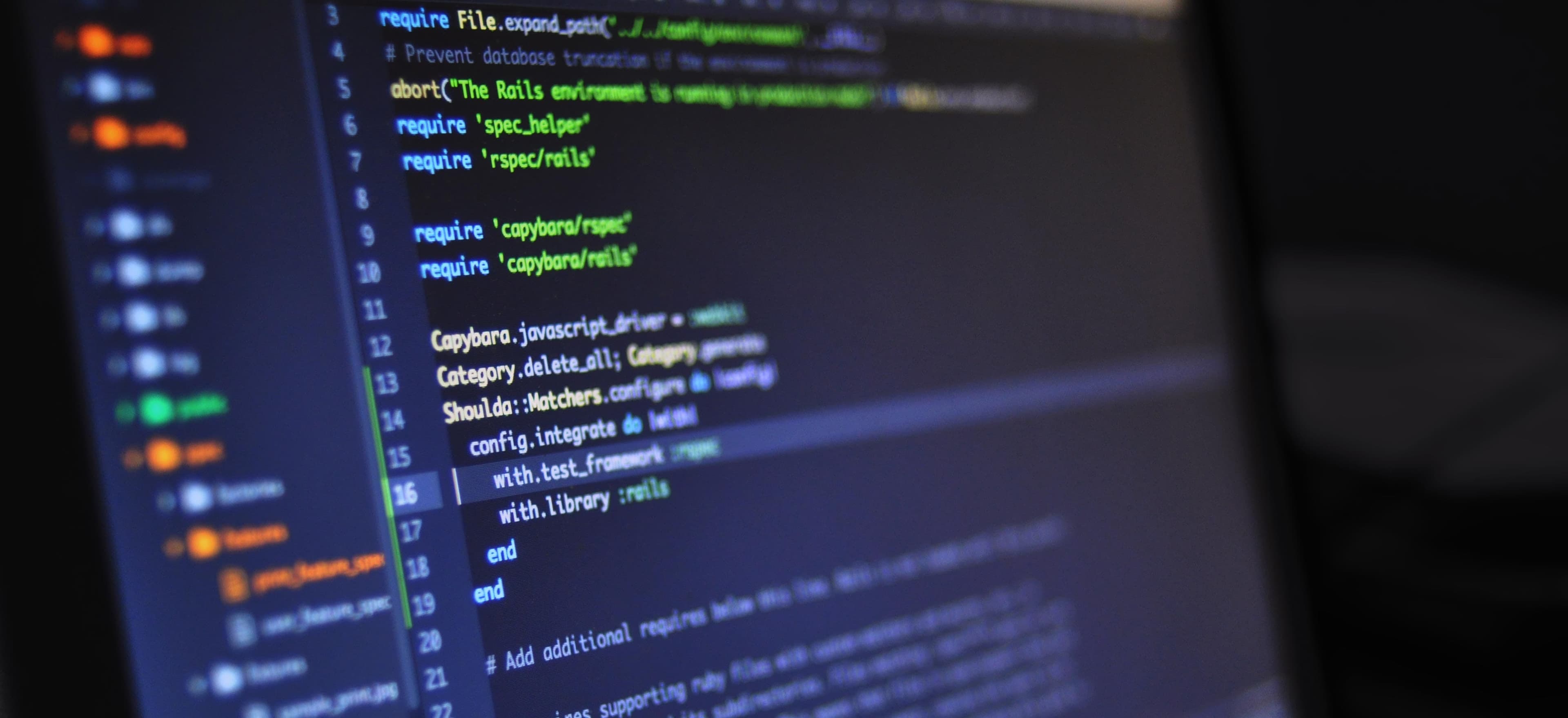
- Published on
Managing Compatibility Issues with Multiple Oracle JDK Versions
When working with Java applications, developers often encounter compatibility issues when using multiple versions of the Oracle JDK. These issues can manifest in various ways, such as runtime errors, unexpected behavior, or performance degradation. In this article, we'll discuss strategies for managing compatibility issues that arise from using multiple Oracle JDK versions.
Understanding Compatibility Issues
Classpath and API Changes
One common source of compatibility issues is the changes in the classpath and API between different JDK versions. New JDK releases often introduce enhancements and deprecations, which can impact the behavior of existing code. For example, a method that was deprecated in an older JDK version may be removed in a newer release, leading to compilation or runtime errors when using the newer JDK.
Runtime Environment Variances
Another factor contributing to compatibility issues is the variance in the runtime environment between different JDK versions. Changes in the JVM implementation, garbage collection algorithms, and runtime optimizations can affect the performance and stability of Java applications. Consequently, an application that runs smoothly on one JDK version may encounter unexpected issues on another version due to differences in the runtime environment.
Native Library and Platform Dependencies
Java applications often rely on native libraries and platform-specific features, which can introduce compatibility challenges when transitioning between JDK versions. Changes in the native method interface, platform-specific behavior, and system dependencies can result in runtime failures or behavioral discrepancies across different JDK versions.
Strategies for Managing Compatibility Issues
Version-Specific Testing
One effective strategy for managing compatibility issues is to perform version-specific testing across different Oracle JDK versions. By running comprehensive test suites on each target JDK version, developers can identify and address compatibility issues early in the development lifecycle. Automated testing tools and continuous integration pipelines can streamline the process of testing compatibility across multiple JDK versions.
Dependency Management
Careful management of dependencies, including third-party libraries and internal modules, is crucial for mitigating compatibility issues across JDK versions. Dependency management tools such as Maven and Gradle offer features for specifying compatible JDK versions, managing transitive dependencies, and resolving version conflicts. By maintaining strict control over dependencies, developers can minimize the risk of compatibility issues stemming from conflicting library versions.
Use of Compatibility Flags and APIs
Oracle JDK releases often provide compatibility flags and APIs to facilitate the migration of applications from older versions to newer releases. By leveraging these compatibility features, developers can ensure that their applications behave consistently across different JDK versions. For instance, the -source
and -target
flags in the Java compiler can be used to specify the source and target JDK versions, enabling developers to maintain backward compatibility while taking advantage of newer language features.
Runtime Environment Configuration
Adjusting the runtime environment configuration, such as JVM parameters and runtime flags, can help address compatibility issues stemming from differences in the underlying runtime environment. For example, tuning the garbage collection settings or adjusting thread pool configurations can improve the performance and stability of an application when running on different JDK versions. Additionally, monitoring tools and profilers can be employed to identify runtime discrepancies and optimize the application's behavior for specific JDK versions.
Exemplary Code Snippets
Using Maven to Specify JDK Version Compatibility
When using Maven as a build tool, developers can specify the target JDK version for their project to ensure compatibility. The following excerpt from a Maven pom.xml
file demonstrates how to define the JDK version for the project:
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
By setting the maven.compiler.source
and maven.compiler.target
properties to the desired JDK version, developers can ensure that the project is compiled and built with compatibility for the specified JDK.
Leveraging Compatibility APIs
In Java, certain APIs are designated as "compatibility APIs," providing a bridge between older and newer JDK versions. For example, the sun.misc.Unsafe
class, though not part of the public API, can be used for compatibility and performance optimizations. The following code snippet demonstrates how to use the sun.misc.Unsafe
class for off-heap memory management:
import sun.misc.Unsafe;
public class OffHeapMemoryAllocator {
private static final Unsafe unsafe = Unsafe.getUnsafe();
// ... rest of the implementation
}
While using compatibility APIs can facilitate interoperability between JDK versions, it's crucial to exercise caution and understanding of the associated risks, as these APIs may not undergo the same level of testing and validation as public APIs.
Final Considerations
Managing compatibility issues with multiple Oracle JDK versions is a critical aspect of Java application development. By understanding the sources of compatibility challenges and employing effective strategies such as version-specific testing, dependency management, and utilization of compatibility features, developers can ensure smooth migration and consistent behavior across different JDK versions. Additionally, staying abreast of Oracle's guidance on compatibility and migration best practices can help mitigate potential issues when transitioning between JDK releases.
In conclusion, proactive management of compatibility issues is essential to maintain the performance, stability, and portability of Java applications in an environment where multiple JDK versions coexist.
By implementing the discussed strategies and leveraging compatibility features, developers can navigate the challenges posed by multiple Oracle JDK versions and ensure a seamless experience for their Java applications.
Interested in more detailed insights? Check out our article on Java Development Best Practices for additional tips and techniques to enhance your Java development workflow.