Maximizing Jenkins Pipelines for Efficient CI/CD
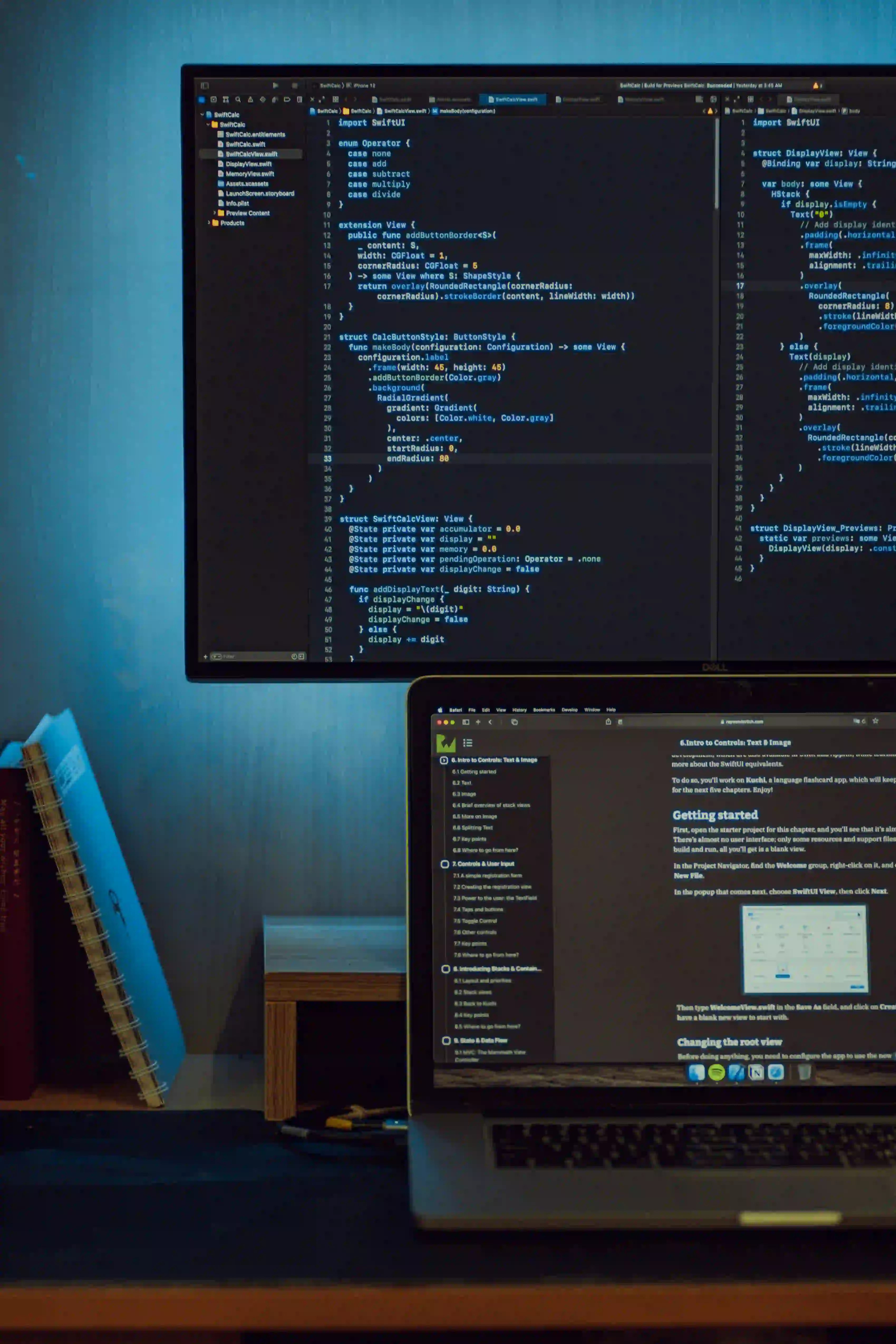
Maximizing Jenkins Pipelines for Efficient CI/CD
Jenkins is a popular open-source automation server that is widely used for continuous integration and continuous delivery (CI/CD) pipelines. Jenkins pipelines allow you to define an entire CI/CD process as code, enabling you to automate the build, test, and deployment of your applications. In this blog post, we will explore how to maximize Jenkins pipelines for efficient CI/CD, covering best practices, optimization techniques, and useful plugins.
Why Jenkins Pipelines?
Jenkins pipelines offer several benefits over traditional freestyle projects, including:
-
Code as Configuration: Pipelines are defined using a Jenkinsfile, which is typically stored in the version control system along with the application code. This allows for versioning, code reviews, and provides a centralized view of the entire CI/CD process.
-
Reusability: Pipelines can be written once and used across multiple projects, promoting consistency and reducing duplication of effort.
-
Scalability: Pipelines can scale to handle complex build and deployment workflows, including parallel and sequential stages, manual approvals, and parameterized builds.
Best Practices for Jenkins Pipelines
1. Keep Pipelines DRY (Don't Repeat Yourself)
Avoid duplicating code within pipelines. Extract common functionality into shared libraries or script templates to promote reusability and maintainability. This reduces the overall codebase and ensures consistency across pipelines.
2. Use Declarative Syntax for Simplicity
Jenkins supports two types of syntax for defining pipelines: Declarative and Scripted. While Scripted syntax offers more flexibility, Declarative syntax provides a simpler and more opinionated way to author pipelines. It's recommended to use Declarative syntax for its readability and maintainability.
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'kubectl apply -f deployment.yaml'
}
}
}
}
3. Use Agent Directive Wisely
The agent
directive specifies where the pipeline will execute. Use the appropriate agent to match the specific requirements of your pipeline, such as docker
for containerized builds or label
for running on specific nodes with defined labels.
4. Parallelize Tests and Builds
To speed up the pipeline execution, parallelize time-consuming tasks such as running tests or building artifacts. Jenkins provides the parallel
directive to execute multiple steps concurrently within a stage.
stage('Test') {
steps {
parallel(
"Unit Tests": { sh 'mvn test' },
"Integration Tests": { sh 'mvn verify' }
)
}
}
5. Utilize Agent Docker Images
When working with Docker-based applications, consider using Docker agent images for pipeline execution. This allows for a clean and isolated build environment without the need to install dependencies on the Jenkins host.
pipeline {
agent {
docker {
image 'maven:3.6.3-jdk-11'
}
}
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
}
}
Optimization Techniques
1. Parallelizing Multiple Pipelines
Jenkins supports parallel execution of multiple pipelines using the Multibranch Pipeline feature. This is particularly useful when handling numerous branches within a repository, as each branch can trigger a separate pipeline execution.
2. Using Workspace Cleanup
Clean up the workspace between different stages or builds to free up disk space and avoid potential conflicts from previous builds. Jenkins provides the cleanWs
step to delete the workspace at the end of a pipeline run.
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
post {
always {
cleanWs()
}
}
}
}
}
3. Caching Dependencies
Improve build performance by utilizing dependency caching. Tools like the Pipeline Maven Integration plugin allow you to cache Maven dependencies between builds, reducing the need to download dependencies on every build.
pipeline {
agent any
options {
skipDefaultCheckout(true) // Skip the default checkout
buildDiscarder(logRotator(numToKeepStr: '3')) // Retain only the last 3 builds
}
stages {
stage('Build') {
steps {
withMaven(maven: 'Maven 3.6.3', mavenSettingsConfig: 'my-maven-settings') {
sh 'mvn clean package'
}
}
}
}
}
Useful Plugins for Jenkins Pipelines
1. Blue Ocean
Blue Ocean is a modern, user-friendly interface for Jenkins that provides visualizations of pipeline runs, making it easier to understand and navigate through the CI/CD process.
2. Pipeline Utility Steps
The Pipeline Utility Steps plugin offers a set of common utility functions that can be used within Jenkins pipelines, such as file operations, string manipulations, and environmental variable management.
Closing the Chapter
In conclusion, Jenkins pipelines offer a powerful way to automate and optimize CI/CD workflows. By following best practices, applying optimization techniques, and leveraging useful plugins, you can maximize the efficiency of Jenkins pipelines for your development projects. Remember to keep your pipelines maintainable, scalable, and adaptable to the evolving needs of your software delivery process.
Start optimizing your Jenkins pipelines today for a more efficient CI/CD experience!
Remember, the power of Jenkins lies in its flexibility, so feel free to adapt these practices to your specific needs and requirements.
Happy optimizing!