Debugging: The Struggle of every Programmer
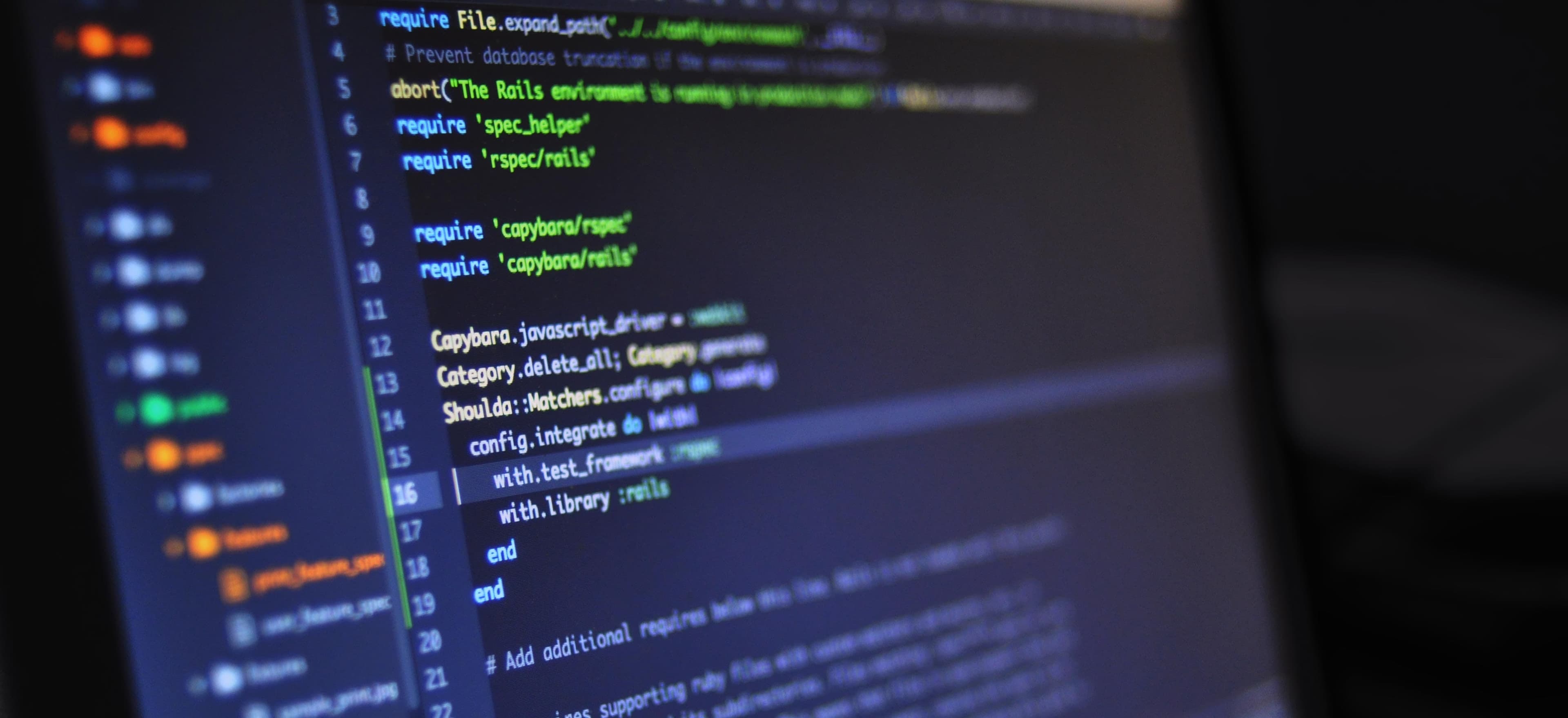
- Published on
Debugging: The Struggle of every Programmer
Debugging is an indispensable part of software development, and it's a skill that every programmer must master. It’s the process of identifying and fixing errors, bugs, and unexpected behavior in the code. Although debugging can be challenging and frustrating at times, it is a crucial skill that separates good programmers from great ones.
In this article, we'll discuss the importance of debugging, some common debugging techniques, and tools that can assist in the debugging process.
The Importance of Debugging
Debugging is essential in the software development lifecycle as it ensures that the code functions as intended. It helps in identifying and resolving issues that might cause the software to crash, produce incorrect results, or behave unexpectedly.
The Cost of Bugs
Bugs can be costly in terms of time, money, and even reputation. They can lead to delays in project delivery, result in user dissatisfaction, and in some cases, cause financial losses. Therefore, effective debugging is vital to ensure high-quality, reliable software.
Common Debugging Techniques
Printing Debug Information
One of the most straightforward ways to debug code is by using print statements. By strategically placing print statements within the code, you can output the values of variables, function calls, and other important information to the console. This can help you understand the flow of the program and identify the point at which things go wrong.
public class DebugExample {
public static void main(String[] args) {
int x = 5;
System.out.println("The value of x is: " + x);
}
}
Using a Debugger
Modern integrated development environments (IDEs) come with powerful debuggers that allow you to step through the code, set breakpoints, inspect variables, and analyze the program's execution flow. This granular level of control and visibility into the code can significantly aid in identifying and rectifying issues.
Code Reviews
Another effective debugging technique is code reviews. Having a fresh pair of eyes look at your code can often uncover issues that you might have overlooked. Code reviews also help in ensuring best practices and maintainable code.
Debugging Tools
IntelliJ IDEA
IntelliJ IDEA is a popular Java IDE that provides extensive debugging capabilities. It offers features like breakpoint debugging, step-through debugging, variable inspection, and more.
Eclipse
Eclipse is another widely used Java IDE that includes a robust debugger. It allows for debugging Java applications, applets, and servlets, and provides powerful features for inspecting variables and controlling program execution.
JDB (Java Debugger)
JDB is a command-line debugger for Java. It can be used for both local and remote debugging and provides a textual interface for debugging Java classes.
Best Practices for Effective Debugging
Reproducing the Issue
Before debugging, it's crucial to be able to reproduce the issue consistently. Understanding the series of steps that lead to the problem is essential for efficient debugging.
Start Small
When faced with a bug, try to isolate the issue by creating a minimal reproduction case. By reducing the problem to its simplest form, it becomes easier to identify and fix the issue.
Version Control
Using version control systems like Git is crucial during debugging. It allows you to track changes, revert to previous versions, and create branches for experimenting with potential fixes.
Utilize Logging
Integrating logging frameworks like Log4j or SLF4J into your codebase can provide valuable insights into the program's behavior. Log messages at different levels (debug, info, error) can help in understanding the flow of the program and pinpointing the issue.
In Conclusion, Here is What Matters
Mastering debugging is essential for every programmer. It not only aids in creating robust, reliable software but also hones problem-solving skills and attention to detail. By employing effective debugging techniques and leveraging the right tools, programmers can streamline the development process and deliver high-quality software. Embracing the challenges of debugging ultimately contributes to becoming a proficient and sought-after developer.
Remember, every bug you squish is a step towards excellence.
So, keep calm and debug on!