Understanding Git Internals with JGit: A Comprehensive Guide
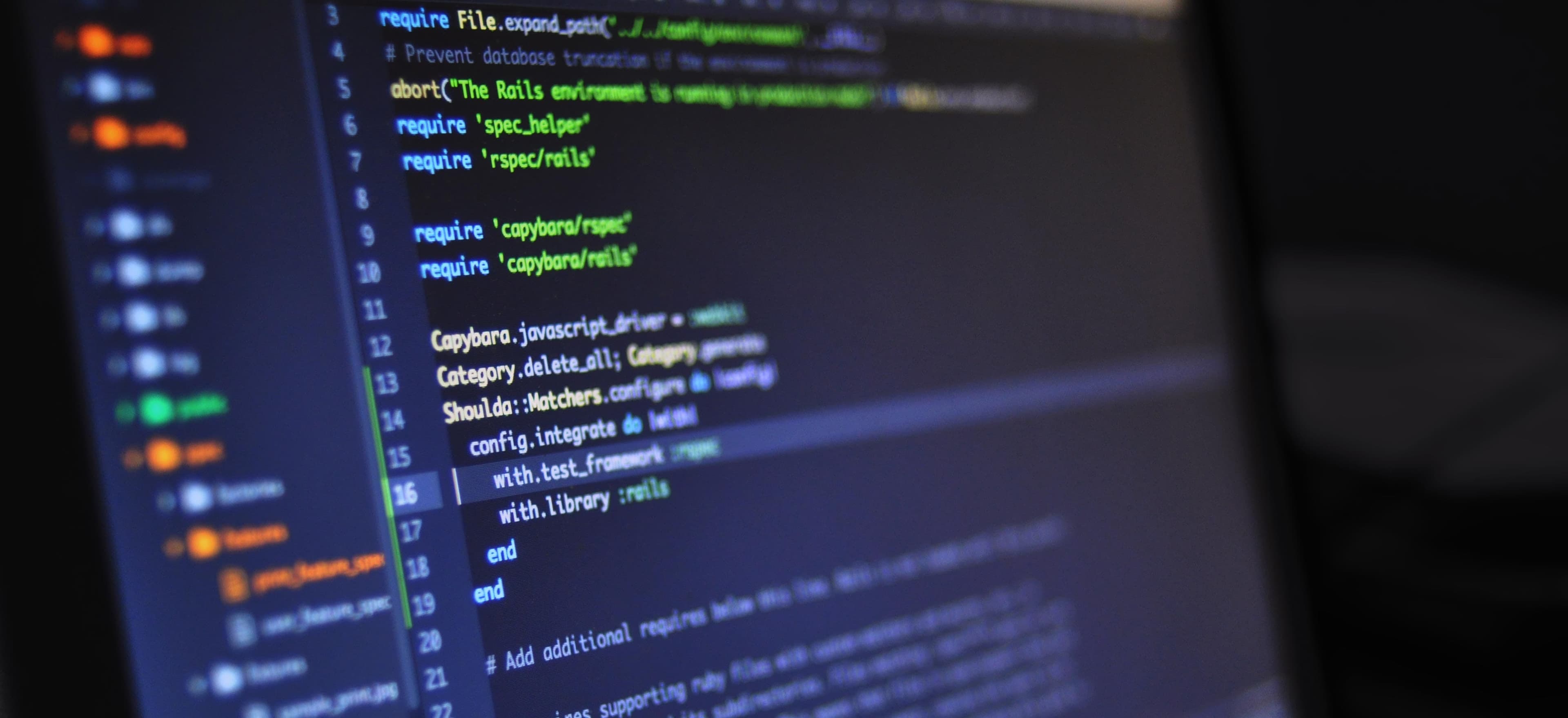
- Published on
Understanding Git Internals with JGit: A Comprehensive Guide
Git is an incredibly powerful and widely used version control system, but have you ever wondered about its internal workings? In this blog post, we will explore the inner workings of Git and how we can leverage the JGit library to interact with Git repositories using Java.
What is JGit?
JGit is an open-source, pure Java implementation of the Git version control system. It provides a high-level API for interacting with Git repositories, allowing Java developers to access and manipulate Git repositories programmatically.
Understanding Git Objects
At the core of Git are its objects - blobs, trees, commits, and tags. Let's delve into each of these objects and understand their role in the Git ecosystem.
Blobs
A blob is simply a file. When you add a file to a Git repository, Git creates a blob object that represents the content of the file. Each blob is identified by a unique SHA-1 hash of its content.
// Retrieve a blob from a Git repository using JGit
ObjectId blobId = repository.resolve("HEAD:file.txt");
ObjectLoader loader = repository.open(blobId);
byte[] bytes = loader.getBytes();
String content = new String(bytes, StandardCharsets.UTF_8);
Trees
A tree object represents a directory. It contains references to blobs (files) and other trees (subdirectories), along with their corresponding file or directory names.
// Retrieve a tree from a Git repository using JGit
RevTree tree = commit.getTree();
try (TreeWalk treeWalk = new TreeWalk(repository)) {
treeWalk.addTree(tree);
treeWalk.setRecursive(true);
while (treeWalk.next()) {
String path = treeWalk.getPathString();
// Process the files and subdirectories within the tree
}
}
Commits
Commits are snapshots of the repository at a specific point in time. Each commit points to a tree object representing the state of the repository at that particular commit.
// Retrieve commit information from a Git repository using JGit
Iterable<RevCommit> logs = git.log().call();
for (RevCommit rev : logs) {
String commitId = rev.getId().name();
PersonIdent author = rev.getAuthorIdent();
String commitMessage = rev.getFullMessage();
// Process the commit information
}
Tags
Tags provide an easy way to mark a specific commit, typically for release versions. They are often used to provide a stable reference point for certain versions of a project.
// Create a lightweight tag in a Git repository using JGit
git.tag().setName("v1.0.0").setObjectId(commit.getId()).call();
Working with Git References
Git references, such as branches and tags, are pointers to specific commits within a repository. Let's see how we can work with references using JGit.
Branches
Branches in Git are lightweight movable pointers to commits. They allow for the creation of independent lines of development within a repository.
// Create a new branch in a Git repository using JGit
RefUpdate newRef = repository.updateRef("refs/heads/new-branch");
newRef.setNewObjectId(commit.getId());
newRef.update();
Tags
As mentioned earlier, tags are used to mark specific points in history, such as release versions of a project.
// List all tags in a Git repository using JGit
Map<String, Ref> tags = repository.getTags();
for (Map.Entry<String, Ref> entry : tags.entrySet()) {
String tagName = entry.getKey();
ObjectId tagId = entry.getValue().getObjectId();
// Process the tag information
}
Managing Git Operations
With JGit, we can perform various Git operations, such as cloning, committing, and pushing changes to remote repositories.
Cloning a Repository
Cloning a repository is the process of creating a local copy of a remote repository. This is a fundamental operation when working with distributed version control systems like Git.
// Clone a remote repository using JGit
Git.cloneRepository()
.setURI("https://github.com/user/repo.git")
.setDirectory(new File("/path/to/local/repository"))
.call();
Committing Changes
Committing changes in Git creates a new snapshot of the repository, capturing the current state of the working directory.
// Stage and commit changes to a Git repository using JGit
git.add().addFilepattern(".").call();
git.commit().setMessage("Commit message").call();
Pushing Changes
Pushing changes to a remote repository makes the committed changes available to others working on the same project.
// Push changes to a remote repository using JGit
git.push().setRemote("origin").setCredentialsProvider(credentialsProvider).call();
Final Considerations
In this guide, we've explored the fundamental concepts of Git internals and demonstrated how JGit can be used to interact with Git repositories using Java. Understanding these core principles and leveraging JGit's API empowers Java developers to build robust tools and applications that integrate seamlessly with Git.
By gaining insights into the inner workings of Git and learning how to harness the power of JGit, developers can take control of version control processes and streamline their workflows, ultimately contributing to more efficient and collaborative development practices.
Now that you have a solid understanding of Git internals and JGit, why not start experimenting with JGit in your next Java project? The possibilities are endless!
Happy coding with Git and JGit!