Troubleshooting Custom Future Implementation Issues
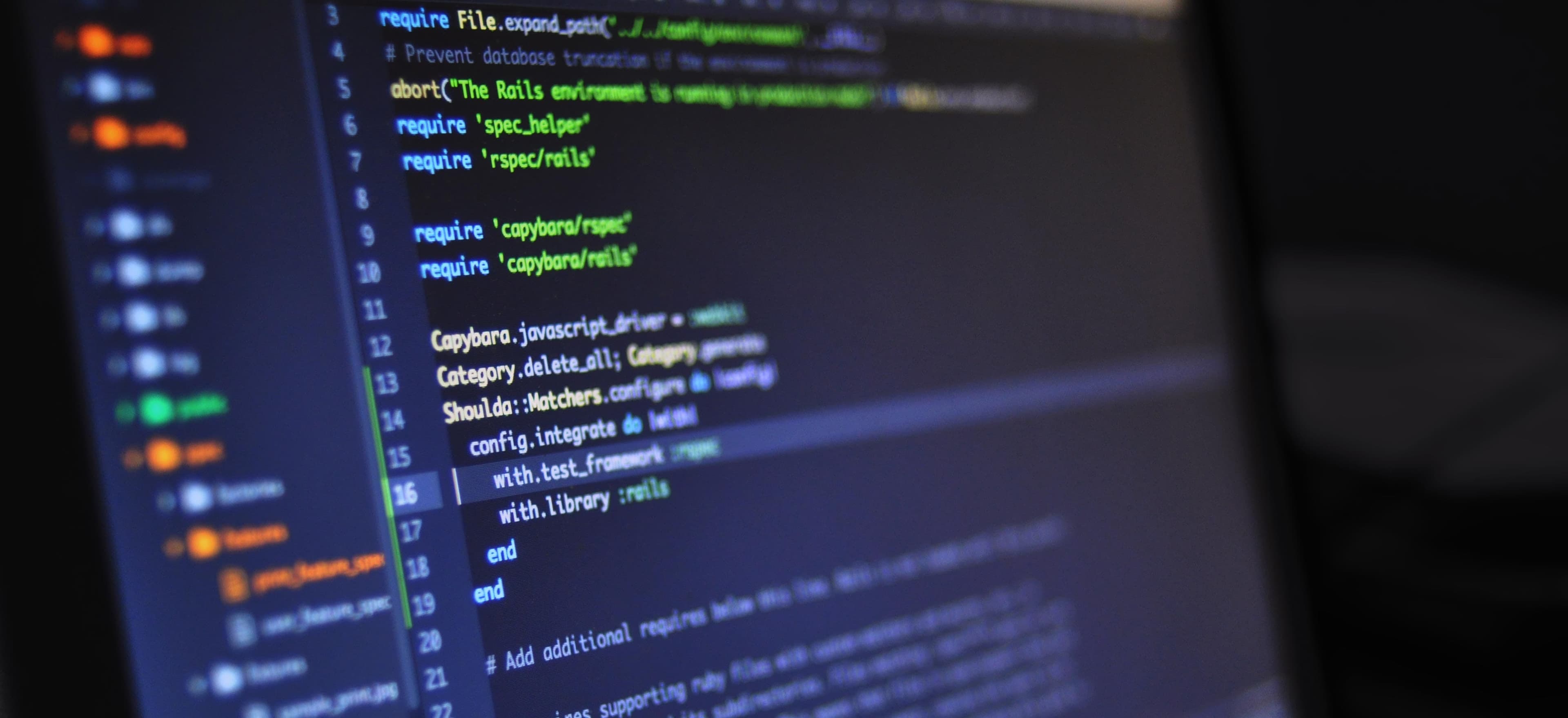
- Published on
Troubleshooting Custom Future Implementation Issues
When working with multithreading and asynchronous operations in Java, using the Future
interface is a common practice. However, implementing custom Future
instances may lead to unexpected issues and errors. In this article, we will explore common problems that developers encounter when working with custom Future
implementations and how to troubleshoot them effectively.
Understanding Custom Future Implementations
Custom Future
implementations can be useful in scenarios where the standard Future
interface does not meet specific requirements. By creating a custom Future
, developers have more control over the asynchronous computation, allowing for additional functionalities and error handling.
Common Issues with Custom Future Implementations
1. Inconsistent State Management
One of the most common issues with custom Future
implementations is inconsistent state management. This can lead to unpredictable behavior and errors when attempting to retrieve the result or handle exceptions.
2. Incorrect Threading and Synchronization
Managing threads and synchronization within a custom Future
implementation is crucial. Failing to handle these aspects properly can result in race conditions, deadlocks, or thread starvation.
3. Improper Exception Handling
Effective exception handling is essential in custom Future
implementations. Mishandling exceptions can lead to silent failures or incorrect results, making it challenging to diagnose and fix issues.
Troubleshooting Custom Future Implementation Issues
Now that we have identified the common issues, let's delve into troubleshooting strategies to address them effectively.
1. Consistent State Management
Maintaining a consistent state in a custom Future
implementation is critical for its functionality. Ensure that state transitions, such as from running
to completed
or exceptional
, are handled correctly.
public class CustomFuture<T> implements Future<T> {
private volatile boolean completed;
private T result;
private Exception exception;
public boolean isDone() {
return completed;
}
public T get() throws InterruptedException, ExecutionException {
// Add proper state checks and handle state transitions
if (completed) {
if (exception != null) {
throw new ExecutionException(exception);
}
return result;
} else {
// Handle incomplete state
}
}
// Additional methods and state handling
}
In the above code snippet, the volatile
keyword ensures that the completed
flag is consistently updated across threads. By properly managing state transitions and access to shared variables, the custom Future
can maintain a consistent state.
2. Threading and Synchronization
When dealing with custom Future
implementations, it's crucial to consider threading and synchronization to avoid concurrent modification and race conditions.
public class CustomFuture<T> implements Future<T> {
private final Object lock = new Object();
public T get() throws InterruptedException, ExecutionException {
synchronized (lock) {
while (!completed) {
lock.wait();
}
// Return result or throw exception
}
}
public void setResult(T result) {
synchronized (lock) {
this.result = result;
this.completed = true;
lock.notifyAll();
}
}
// Additional methods and synchronization
}
In the above code, a dedicated lock object lock
is used to synchronize access to shared variables and coordinate state changes. By using proper synchronization, potential threading issues can be mitigated.
3. Exception Handling
Proper exception handling within custom Future
implementations is crucial for effectively communicating errors and failures.
public class CustomFuture<T> implements Future<T> {
// ...
public T get() throws InterruptedException, ExecutionException {
if (completed) {
if (exception != null) {
throw new ExecutionException(exception);
}
return result;
} else {
throw new InterruptedException("Operation was interrupted");
}
}
public void setException(Exception exception) {
synchronized (lock) {
this.exception = exception;
this.completed = true;
lock.notifyAll();
}
}
// Additional exception handling
}
In the provided code snippet, setting and propagating exceptions through the setException
method ensures that exceptional conditions are properly communicated to the caller.
Key Takeaways
Implementing custom Future
instances in Java can offer flexibility and control over asynchronous computations. However, it's essential to troubleshoot and address common issues such as inconsistent state management, threading and synchronization, and exception handling to ensure the reliability and robustness of custom Future
implementations.
By applying proper state management, threading and synchronization techniques, and effective exception handling, developers can create and maintain custom Future
implementations that function predictably and reliably.
Remember that troubleshooting custom Future
implementation issues requires attention to detail and thorough testing to ensure the correctness and robustness of the asynchronous operations.
For further understanding, you can refer to the official Java documentation on Future and Concurrency in Java.
Happy coding!