Scope Ambiguity: Resolving Injection Qualifier Issues
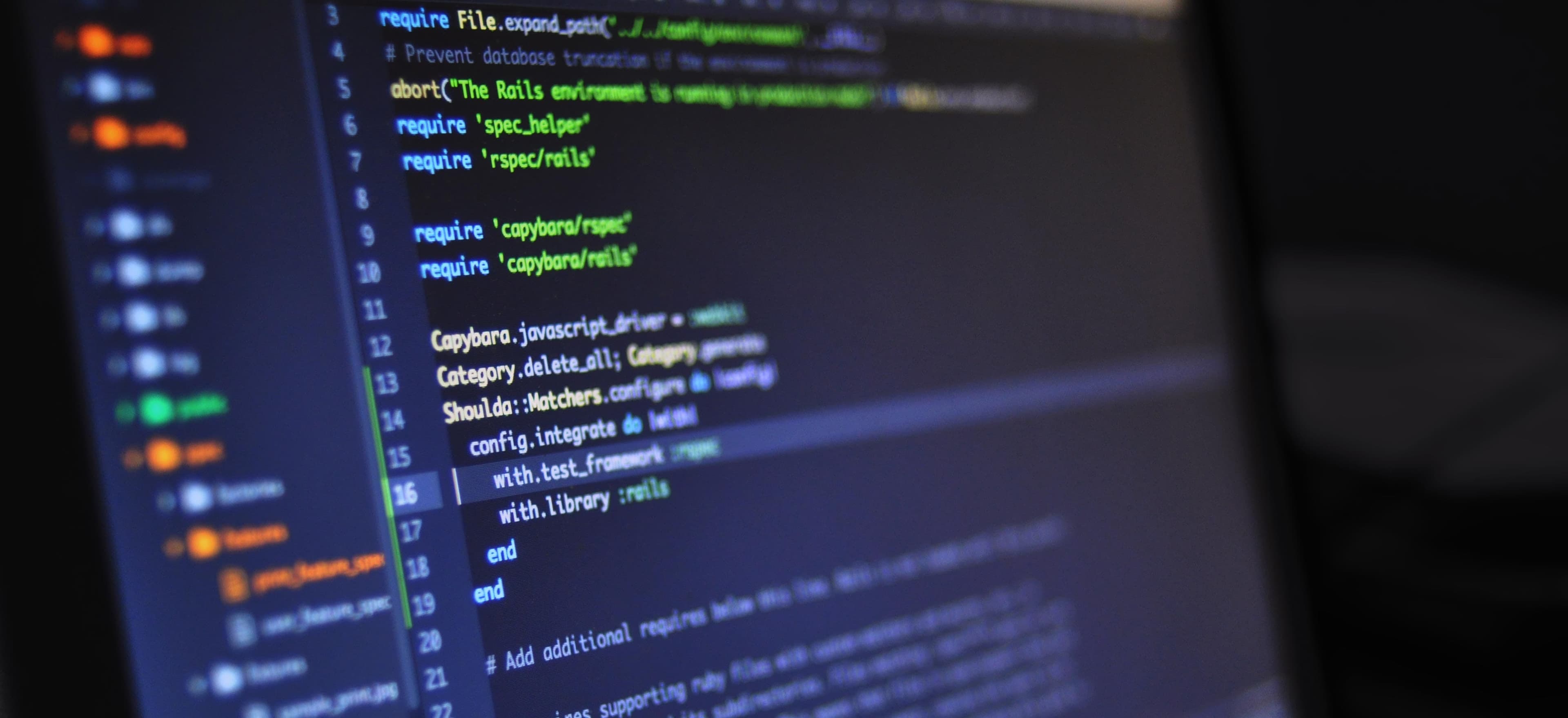
- Published on
Scope Ambiguity: Resolving Injection Qualifier Issues
When working with a large Java codebase, it's common to encounter scenarios where multiple implementations of the same interface need to be used in different parts of the application. This can lead to what is known as "scope ambiguity", where the dependency injection framework is unable to determine which specific implementation to inject. In this blog post, we'll discuss the concept of scope ambiguity in the context of dependency injection, explore its implications, and provide solutions to resolve injection qualifier issues.
Understanding Scope Ambiguity
Imagine a scenario where you have an interface PaymentGateway
with multiple implementations such as PayPalPaymentGateway
and CreditCardPaymentGateway
. Now, consider a class PaymentService
which requires a PaymentGateway
implementation to process payments. When you attempt to inject a PaymentGateway
into PaymentService
, the question arises: which implementation should be injected? This is the essence of scope ambiguity in dependency injection.
Scope ambiguity occurs when there are multiple candidate beans that could be injected into a single dependency. Without explicit instructions, the dependency injection framework cannot determine which bean to inject, leading to a potential runtime error.
Implications of Scope Ambiguity
Scope ambiguity can have several implications on the application:
- Runtime Failures: If the framework is unable to determine the correct bean to inject, it may result in runtime failures, leading to unexpected behavior in the application.
- Maintenance Challenges: As the codebase evolves, new implementations of interfaces may be added, exacerbating the scope ambiguity problem. This can make the codebase hard to maintain and extend.
Resolving Injection Qualifier Issues
To address scope ambiguity in dependency injection, we can employ several techniques and annotations provided by popular frameworks like Spring and CDI. Let's explore these solutions in detail.
1. Using Qualifier Annotations
In Java EE and CDI (Contexts and Dependency Injection), the @Qualifier
annotation is used to disambiguate bean instances at the injection point. By creating custom qualifiers, we can explicitly specify which implementation should be injected.
@Qualifier
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.FIELD, ElementType.TYPE, ElementType.METHOD})
public @interface PayPal {}
@Qualifier
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.FIELD, ElementType.TYPE, ElementType.METHOD})
public @interface CreditCard {}
In the above code, we've defined custom qualifiers @PayPal
and @CreditCard
. Now, we can use these qualifiers to disambiguate the injection points in our code.
@Inject
@PayPal
private PaymentGateway paymentGateway;
By using the @PayPal
qualifier, we explicitly instruct the dependency injection framework to inject the PayPalPaymentGateway
implementation. Similarly, we can use @CreditCard
qualifier for the CreditCardPaymentGateway
implementation.
2. Qualifier Metadata
In Spring framework, qualifiers are combined with metadata to disambiguate beans. By using meta-annotations and combining them with the @Qualifier
annotation, we can provide additional context to the dependency injection framework.
@Target({ElementType.FIELD, ElementType.METHOD, ElementType.PARAMETER, ElementType.TYPE, ElementType.ANNOTATION_TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Qualifier
public @interface PayPal {}
@Target({ElementType.FIELD, ElementType.METHOD, ElementType.PARAMETER, ElementType.TYPE, ElementType.ANNOTATION_TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Qualifier
public @interface CreditCard {}
Now, we can use the meta-annotations to disambiguate the injection points, providing clear metadata about the type of implementation being injected.
3. Using Primary Annotation
In the Spring framework, the @Primary
annotation can be used to indicate a "primary" bean when multiple candidates are available for injection. This annotation acts as a hint to the dependency injection framework to prioritize the annotated bean for injection.
@Bean
@Primary
public PaymentGateway payPalPaymentGateway() {
return new PayPalPaymentGateway();
}
In this example, we've marked the PayPalPaymentGateway
bean as primary, ensuring that it will be injected when a PaymentGateway
implementation is required, unless explicitly overridden.
Final Considerations
In conclusion, scope ambiguity in dependency injection can lead to runtime failures and maintenance challenges in a Java application. By using qualifier annotations, metadata, and the @Primary
annotation, we can effectively resolve injection qualifier issues, providing clear instructions to the dependency injection framework about which bean implementation should be injected. It's crucial to understand these techniques to ensure smooth dependency injection in complex Java codebases.
By implementing these solutions, developers can mitigate the impact of scope ambiguity, leading to more robust and maintainable code.
For further reading, you can delve into the official documentation of Spring Framework and CDI to deepen your understanding of resolving injection qualifier issues.