Working with Path Parameters in Java EE 8 MVC
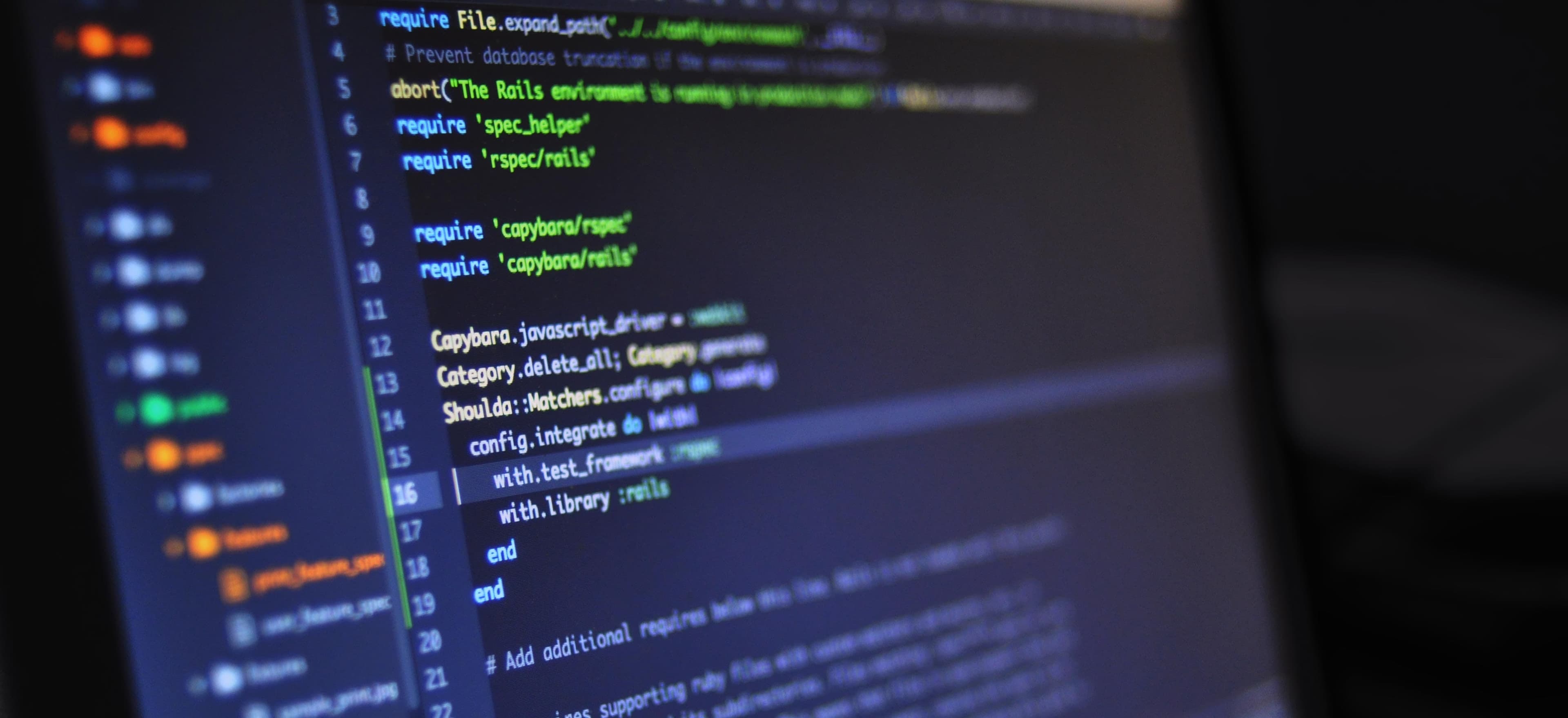
- Published on
Exploring Path Parameters in Java EE 8 MVC
In the world of web development, handling user inputs is a critical aspect. Whether it's retrieving user-specific data or performing specific actions based on user input, effectively managing data passed through URLs is essential. Java EE 8 MVC provides a powerful way to handle this through path parameters.
Understanding Path Parameters
Path parameters are dynamic values within the URL path of a web request. They allow the endpoint to handle variable data, enabling more flexible and efficient routing. In the context of Java EE 8 MVC, path parameters can be utilized to capture variables from the URL and use them within the application's logic.
Setting Up a Java EE 8 MVC Project
To begin working with path parameters in Java EE 8, it's essential to have a project set up. Assuming a basic understanding of Java EE 8 and a compatible IDE such as IntelliJ IDEA or Eclipse, let's start by creating a new Java EE 8 MVC project or using an existing one.
Maven Dependency
Make sure to include the Java EE 8 dependencies in the pom.xml
file:
<dependencies>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>8.0.1</version>
<scope>provided</scope>
</dependency>
</dependencies>
Controller Class
Create a controller class to handle the incoming requests and process the path parameters:
import javax.mvc.Controller;
import javax.mvc.Models;
import javax.mvc.View;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.inject.Inject;
@Controller
@Path("/users")
public class UserController {
@Inject
private Models models;
@GET
@Path("/{id}")
@View("user-details.jsp")
public void getUserDetails(@PathParam("id") String userId) {
// Logic to retrieve user details based on the ID
models.put("userId", userId);
}
}
In this example, the getUserDetails
method uses the @PathParam
annotation to capture the id
from the URL, which is then used to retrieve the user details.
JSP View
Create a JSP view file to render the user details:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>User Details</title>
</head>
<body>
<h1>User Details</h1>
<p>User ID: ${userId}</p>
<!-- Other user details output here -->
</body>
</html>
Accessing Path Parameters
Now that the project is set up, let's delve into how path parameters can be accessed and utilized within the Java EE 8 MVC application.
Path Parameter Capture
In the UserController
class, the @PathParam("id")
annotation captures the id
from the URL as a method parameter. This allows for seamless integration of path parameters into the application logic.
Dynamic Routing
By incorporating path parameters, the application gains the capability to dynamically route requests based on the values present in the URL. This flexibility enables a more robust and adaptable approach to handling user input.
Cleaner URL Structures
Utilizing path parameters results in cleaner and more descriptive URLs. Instead of query parameters or cumbersome URL structures, path parameters offer a more elegant and user-friendly approach to passing data within the URL.
Best Practices and Considerations
While working with path parameters, it's important to keep certain best practices in mind to ensure optimal implementation and maintainability.
Validation and Error Handling
Always validate and handle path parameters appropriately. Input validation is crucial to prevent potential security vulnerabilities and ensure the application's robustness.
Path Parameter Mapping
Consider the mapping of path parameters to domain entities or database records. Designing a clear and consistent mapping strategy can streamline the process of retrieving and processing data.
Parameterized Queries
When utilizing path parameters for database interactions, parameterized queries should be used to safeguard against SQL injection attacks and maintain data integrity.
Conclusion
In conclusion, path parameters in Java EE 8 MVC provide a powerful mechanism for handling dynamic data within web applications. By embracing path parameters, developers can create more flexible, efficient, and user-friendly web experiences. It's crucial to understand the nuances of working with path parameters and adhere to best practices to ensure the security and reliability of the application.
Java EE 8 MVC empowers developers to harness the potential of path parameters in an intuitive and effective manner, paving the way for innovative and dynamic web applications. Incorporating path parameters into the development workflow can significantly enhance the overall user experience and streamline the handling of user input.
By mastering path parameters in Java EE 8 MVC, developers can elevate their web development skills and deliver exceptional, data-driven applications that efficiently capture and process user-specific data. Whether it's retrieving personalized content or executing tailored actions, path parameters play a pivotal role in shaping modern web applications. Embrace the power of path parameters and unlock new dimensions of web development with Java EE 8 MVC.
Remember, path parameters are not only about capturing values from URLs- they're about enabling dynamic, user-driven interactions within web applications. Mastering their usage is a crucial skill for any modern web developer using Java EE 8 MVC.