Streamlining Jenkins Job Configuration Management
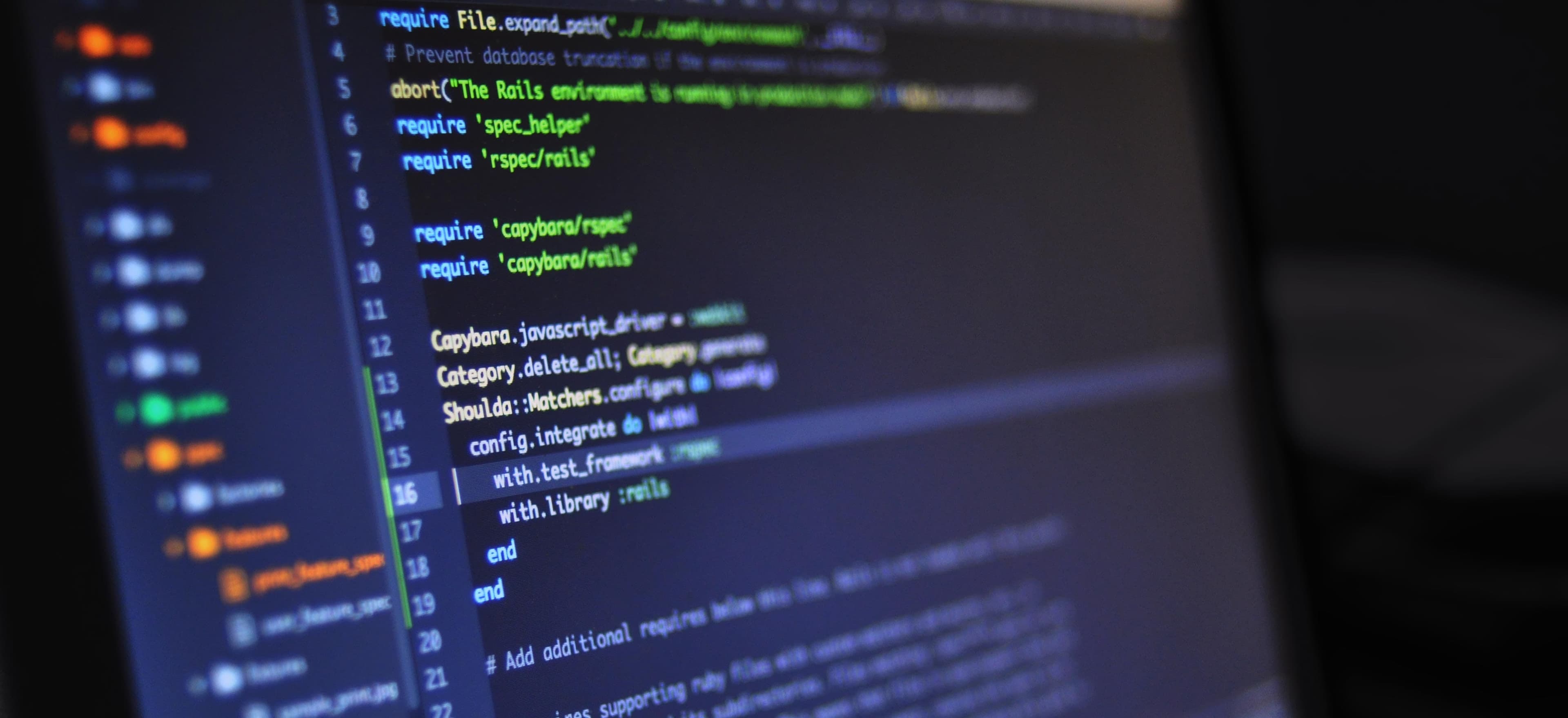
- Published on
Streamlining Jenkins Job Configuration Management
Jenkins is a powerful automation server that allows development teams to automate their build, test, and deployment processes. As projects grow, the number of Jenkins jobs also increases, leading to a complex configuration management challenge. In this blog post, we will explore strategies to streamline Jenkins job configuration management to improve efficiency and maintainability.
Understanding the Challenge
As teams expand, Jenkins configurations become more intricate, with numerous jobs, complex job interdependencies, and a multitude of plugins. Managing these configurations manually not only consumes time but also increases the risk of human errors, resulting in inconsistent job configurations.
Leveraging Jenkins Job DSL
Jenkins Job DSL, a popular Jenkins plugin, provides a programmatic way to define Jenkins jobs. Instead of manually configuring jobs through the Jenkins UI, the Job DSL plugin allows developers to define job configurations in code using the Groovy programming language. This approach offers several benefits:
-
Version Control: Job DSL scripts can be versioned using Git or any other version control system, enabling easier tracking of changes, rollbacks, and collaboration among team members.
-
Reusability: With Job DSL, you can define job templates and reuse them across multiple jobs, ensuring consistency and reducing duplication.
-
Automated Management: Automation of job creation and updates through Job DSL reduces manual effort and enforces consistency in configurations.
Example of Job DSL Script
job('exampleJob') {
scm {
git('https://github.com/example.git')
}
steps {
maven('-e clean test')
}
triggers {
cron('@daily')
}
}
In this example, a Job DSL script defines a Jenkins job named 'exampleJob' that pulls source code from a Git repository, runs a Maven build with the 'clean test' goal, and triggers the job daily. With this script, the job configuration becomes code, facilitating easier management and automation.
Embracing Jenkins Pipeline
Jenkins Pipeline is another essential feature that simplifies job configuration management. It allows you to define your entire build process as code, comprising stages, steps, and post-actions, and can be versioned and stored alongside your application codebase.
Benefits of Jenkins Pipeline
-
Visibility: Pipeline scripts provide a clear and concise view of the entire build process, making it easier to understand and maintain.
-
Scalability: As projects evolve, pipeline scripts can scale to accommodate complex build, test, and deployment workflows.
-
Reusability and Modularity: Pipelines can be modularized, shared, and reused across multiple projects, promoting consistency and reducing redundancy.
Example of Jenkins Declarative Pipeline
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'kubectl apply -f deployment.yaml'
}
}
}
}
In this example, a declarative Jenkins Pipeline script defines three stages: Build, Test, and Deploy. Each stage contains specific steps, such as running Maven commands for build and test stages and deploying the application using Kubernetes manifests. The entire build process is clearly defined in code, allowing for easy management and modification.
Managing Configuration as Code
As we have seen, leveraging Job DSL and Jenkins Pipeline enables us to manage Jenkins job configurations as code. However, to fully optimize the process, it's crucial to treat these configuration scripts like any other software code, adhering to best practices such as code reviews, unit tests, and continuous integration processes.
Best Practices for Managing Configuration as Code
-
Code Reviews: Subjecting job configuration scripts to code reviews ensures quality, consistency, and adherence to standards.
-
Testing: Writing unit tests for configuration scripts validates their correctness and prevents unintended side effects.
-
Continuous Integration: Integrating job configuration scripts into CI/CD pipelines ensures that any changes are automatically validated and applied to Jenkins, reducing the risk of misconfigurations.
-
Modularization: Just like application code, modularization of job DSL scripts and pipeline scripts promotes reusability and maintainability.
Wrapping Up
In conclusion, as your Jenkins instance grows in complexity, managing job configurations manually becomes challenging and error-prone. Adopting practices such as Job DSL and Jenkins Pipeline, coupled with treating configuration scripts as code, streamlines Jenkins job configuration management, improves consistency, and enhances maintainability. By embracing these strategies, development teams can efficiently manage their Jenkins job configurations and focus on delivering high-quality software.