Maximize Code Reusability with Composite Design Pattern
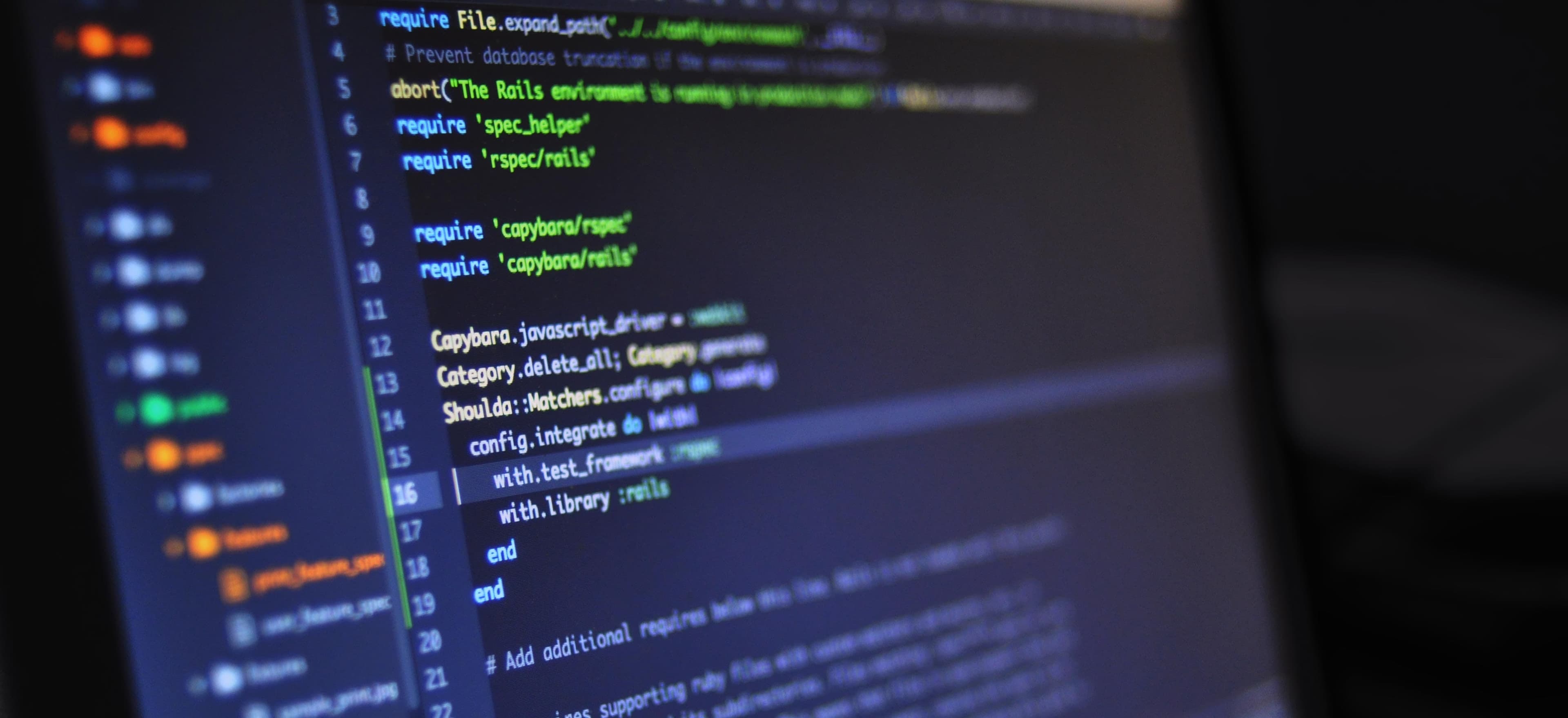
- Published on
Maximizing Code Reusability with Composite Design Pattern
In the world of software development, reusability is a critical aspect that can save time and effort. One of the design patterns that greatly supports code reusability is the Composite Design Pattern. In this blog post, we will explore how this design pattern can help maximize code reusability in Java applications.
Understanding the Composite Design Pattern
The Composite Design Pattern is a structural pattern that allows you to compose objects into tree-like structures to represent part-whole hierarchies. This pattern treats individual objects and compositions of objects uniformly, making it easier to work with complex hierarchies.
Use Case for Composite Design Pattern
Imagine a scenario where you need to work with a hierarchy of elements, such as a file system. Each element in the hierarchy can be a file or a directory, and directories can contain files or other directories. With the Composite Design Pattern, you can treat both individual files and directories the same way, which simplifies the code and promotes reusability.
Implementation of the Composite Design Pattern in Java
Let's dive into a practical example to understand how the Composite Design Pattern can be implemented in Java. We'll create a simple hierarchy of graphic elements, such as shapes and groups of shapes, to demonstrate the application of this design pattern.
Step 1: Defining the Component Interface
public interface Graphic {
void draw();
}
In this step, we define the Graphic
interface, which serves as the component for the hierarchy. The draw
method will be implemented by both individual graphic elements and composite graphic elements.
Step 2: Implementing Leaf Components
public class Ellipse implements Graphic {
@Override
public void draw() {
System.out.println("Drawing Ellipse");
}
}
public class Square implements Graphic {
@Override
public void draw() {
System.out.println("Drawing Square");
}
}
Here, Ellipse
and Square
are the leaf components of the hierarchy. They implement the draw
method to define how they should be drawn.
Step 3: Implementing Composite Components
import java.util.ArrayList;
import java.util.List;
public class Group implements Graphic {
private List<Graphic> graphics = new ArrayList<>();
public void add(Graphic graphic) {
graphics.add(graphic);
}
@Override
public void draw() {
System.out.println("Drawing Group");
for (Graphic graphic : graphics) {
graphic.draw();
}
}
}
The Group
class represents the composite component of the hierarchy. It maintains a collection of Graphic
elements and implements the draw
method to iterate through and draw each element.
Step 4: Utilizing the Composite Hierarchy
public class Main {
public static void main(String[] args) {
Graphic ellipse = new Ellipse();
Graphic square = new Square();
Group group = new Group();
group.add(ellipse);
group.add(square);
group.draw();
}
}
In the main class, we create instances of leaf components (ellipse and square) and a composite component (group). We add the leaf components to the group and call the draw
method on the group to see the entire hierarchy being drawn.
Benefits of Using the Composite Design Pattern for Code Reusability
Now that we have implemented the Composite Design Pattern, let's explore the benefits it offers in terms of code reusability.
Treating Components Uniformly
The Composite Design Pattern allows us to treat individual elements and compositions of elements uniformly. This means that the client code can work with both individual elements and composite elements in a consistent manner, promoting code reusability.
Simplifying Client Code
By using the Composite Design Pattern, the client code becomes simpler and more intuitive. Regardless of whether the client is dealing with a single element or a hierarchy of elements, the same methods and operations can be used, eliminating the need for conditional checks and special cases.
Easy Addition of New Component Types
Adding new types of components to the hierarchy becomes straightforward with the Composite Design Pattern. Whether it's a new leaf component or a new composite component, the existing client code remains unaffected, providing excellent reusability and scalability.
Closing the Chapter
In conclusion, the Composite Design Pattern is a powerful tool for maximizing code reusability in Java applications, especially when dealing with hierarchical data structures. By treating individual elements and compositions uniformly, simplifying client code, and facilitating the addition of new component types, this design pattern promotes a modular and reusable codebase.
When working on projects that involve complex hierarchies of elements, considering the application of the Composite Design Pattern can lead to more maintainable and flexible code, ultimately saving time and effort in the long run.
To delve deeper into how design patterns can enhance code quality, check out this comprehensive guide on Java Design Patterns.
Remember, code reusability is not just a convenience—it's a strategic advantage in software development. Utilize design patterns like the Composite Design Pattern to unlock the full potential of reusability in your Java projects.
Checkout our other articles