Cracking the Code: Mastering Software Engineering Job Interviews
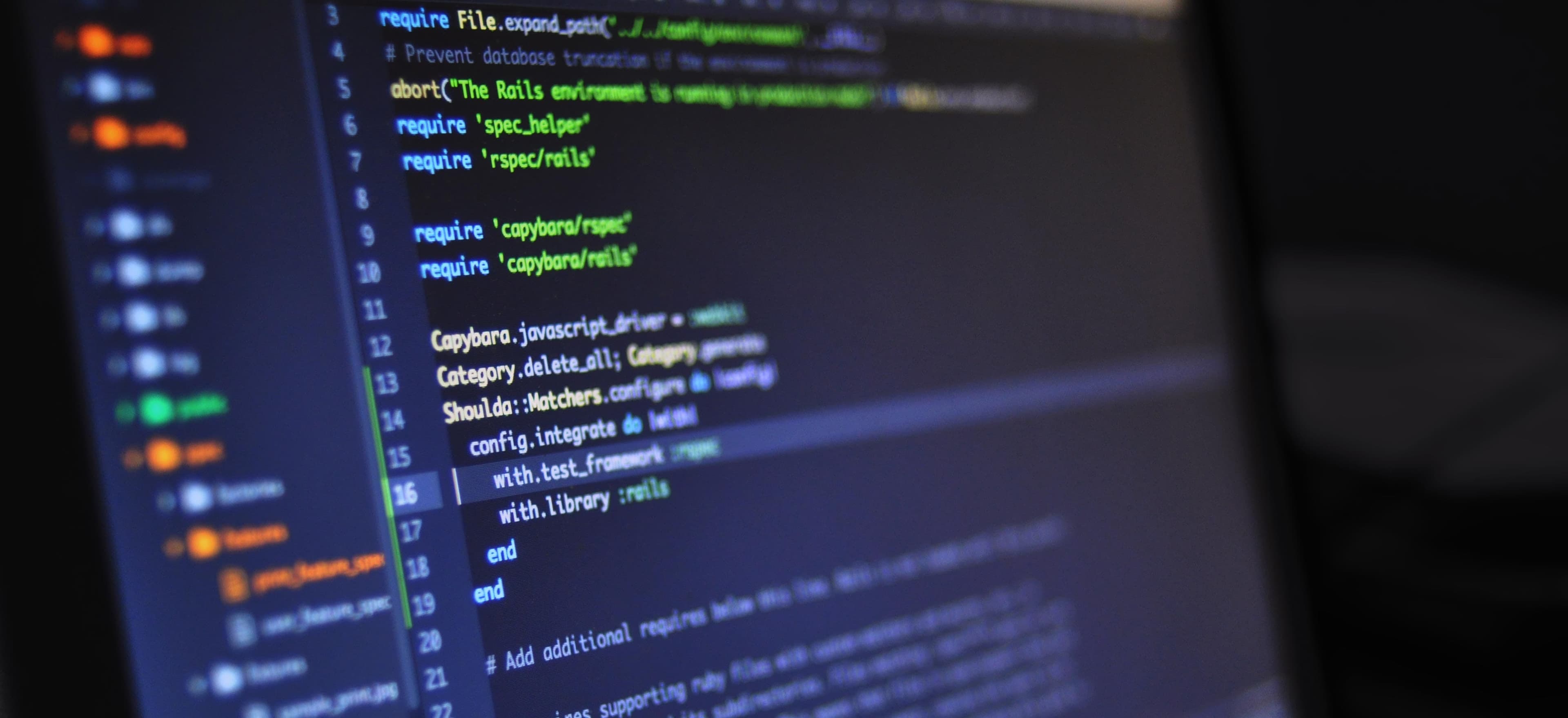
- Published on
Mastering Software Engineering Job Interviews: A Guide to Cracking the Code
In the world of software engineering, technical interviews are a crucial step in landing your dream job. These interviews often include algorithmic problem-solving, data structures, system design, and object-oriented analysis. As a software engineer, mastering Java is essential for excelling in these technical interviews.
In this blog post, we will explore some crucial Java concepts and techniques to help you ace your software engineering job interviews. We will cover essential topics such as Java collections, multithreading, object-oriented programming, and more. We’ll also provide code snippets and explanations to deepen your understanding.
Java Collections Framework
The Java Collections Framework provides a comprehensive set of classes and interfaces that facilitate the handling of collections of objects. Understanding the different collection types and when to use them is vital for solving coding problems efficiently.
Example: Using HashMap to Count Occurrences of Elements in an Array
import java.util.*;
public class ElementCounter {
public static void main(String[] args) {
int[] array = {1, 2, 3, 2, 3, 1, 3, 4, 5, 4};
Map<Integer, Integer> elementCount = new HashMap<>();
for (int num : array) {
elementCount.put(num, elementCount.getOrDefault(num, 0) + 1);
}
System.out.println(elementCount);
}
}
In this example, we demonstrate how to use a HashMap
to efficiently count occurrences of elements in an array. Utilizing the getOrDefault
method helps us avoid redundant if-else checks, resulting in cleaner and more concise code.
Multithreading in Java
Multithreading is a fundamental concept in Java, allowing concurrent execution of multiple threads within a single process. Interviewers often test candidates on multithreading to assess their understanding of synchronization, thread communication, and parallel processing.
Example: Implementing Runnable Interface for Multithreading
public class SimpleRunnableExample {
public static void main(String[] args) {
Runnable task = () -> {
System.out.println("This code is running in a separate thread");
};
Thread thread = new Thread(task);
thread.start();
}
}
Here, we demonstrate the implementation of the Runnable
interface to create a simple multithreaded application. Understanding how to create and manage threads is essential for leveraging the power of multithreading in Java.
Object-Oriented Programming (OOP) Principles
Solid knowledge of OOP principles is imperative for software engineering interviews. Concepts such as inheritance, encapsulation, polymorphism, and abstraction are frequently tested.
Example: Applying Inheritance in Java
class Animal {
void eat() {
System.out.println("The animal is eating");
}
}
class Dog extends Animal {
void bark() {
System.out.println("The dog is barking");
}
}
public class InheritanceExample {
public static void main(String[] args) {
Dog labrador = new Dog();
labrador.eat(); // Inherited from the Animal class
labrador.bark();
}
}
In this example, we showcase inheritance, a key OOP concept. The Dog
class inherits the eat
method from the Animal
class, demonstrating code reusability and hierarchical relationships.
Java Design Patterns
Understanding design patterns is crucial for demonstrating good software design principles during interviews. Design patterns such as Singleton, Factory, and Strategy are frequently discussed and utilized in software engineering.
Example: Singleton Design Pattern
public class Singleton {
private static Singleton instance;
private Singleton() {} // Private constructor to prevent instantiation
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. Implementing this pattern showcases your understanding of creating and managing unique instances in a multithreaded environment.
Key Takeaways
Mastering Java for software engineering job interviews is a continuous learning process. By delving into Java collections, multithreading, OOP principles, and design patterns, you can enhance your problem-solving skills and demonstrate your proficiency during technical interviews. Remember to practice coding problems regularly, understand the rationale behind the code, and stay updated with industry best practices.
Sharpen your Java skills, ace those technical interviews, and land the software engineering job of your dreams! Happy coding!
For further reading on Java interview preparation, consider exploring resources such as LeetCode and GeeksforGeeks. These platforms offer a wide array of coding problems and solutions to help you hone your skills.