Challenges with Strong Encapsulation of JDK Internals
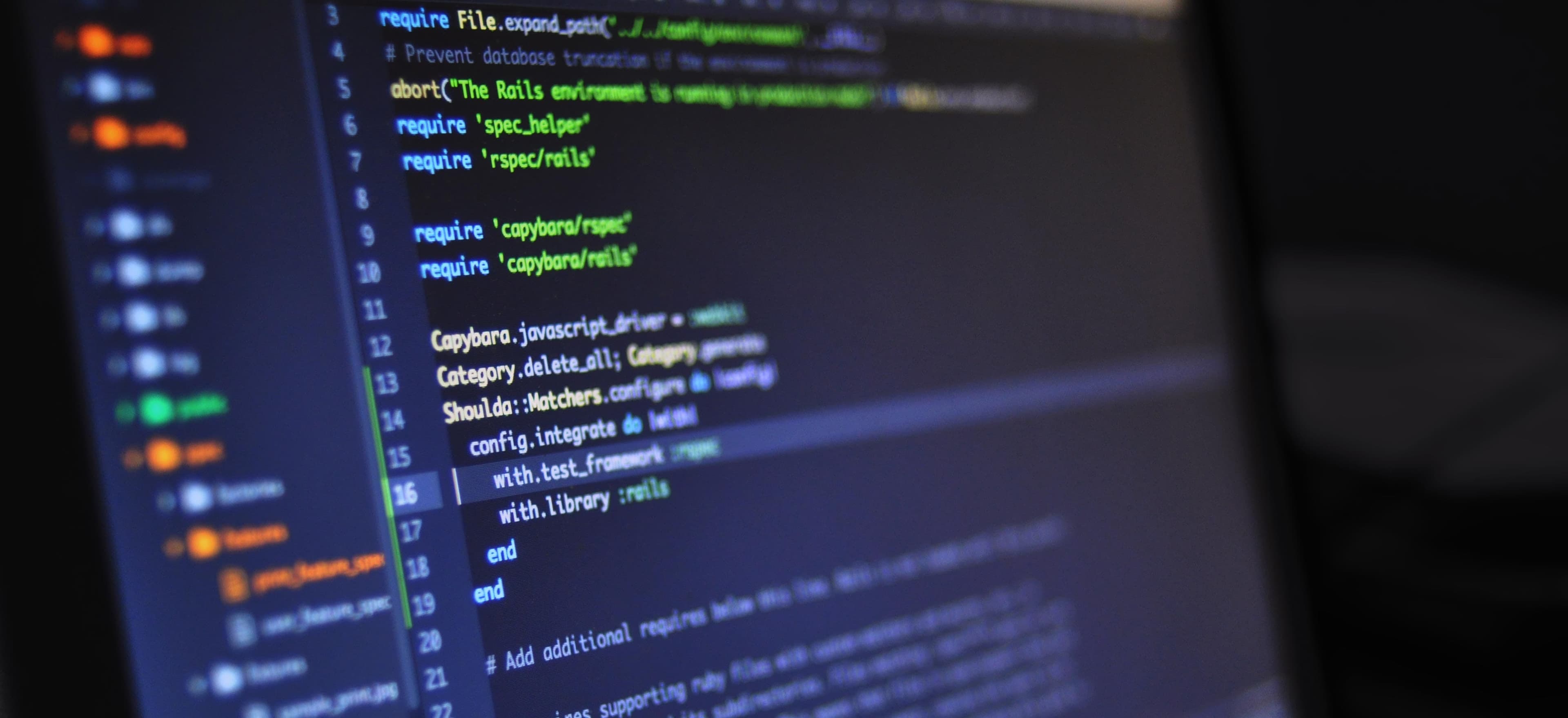
- Published on
Understanding the Challenges with Strong Encapsulation of JDK Internals
In the world of Java development, encapsulation is a fundamental principle of object-oriented programming. It allows for the hiding of internal details of a class from the outside world and enables better control over the accessibility of the class members. With the introduction of modules in Java 9, a strong emphasis was placed on encapsulating JDK internals to ensure a more reliable and secure platform. However, this move has brought with it a set of challenges that Java developers need to address when working with JDK internals.
The Evolution of Encapsulation in Java
Encapsulation has been a core tenet of Java programming since its inception. It allows developers to build robust and maintainable systems by controlling access to the internal state of objects. In the early days of Java, encapsulation was primarily achieved through the use of access modifiers such as public
, private
, and protected
.
With the release of Java 9, the introduction of the Java Platform Module System (JPMS) brought a new dimension to encapsulation. It aimed to address the long-standing issue of the lack of strong encapsulation for JDK internals. The goal was to hide internal APIs and implementation details from the public and prevent developers from relying on non-standard APIs.
The Benefits of Strong Encapsulation
Strong encapsulation of JDK internals offers several benefits:
- Improved Security: By restricting access to internal APIs, the surface area for potential security vulnerabilities is reduced, making the platform more secure.
- Enhanced Stability: Strong encapsulation encourages developers to use only the public APIs, leading to more stable applications that are less prone to breaking due to changes in internal APIs.
- Better Modularization: Modules provide a better way to organize code and dependencies, leading to more maintainable and scalable systems.
Challenges with Strong Encapsulation
While strong encapsulation of JDK internals brings a host of benefits, it also presents several challenges for Java developers.
1. Migration from Deprecated APIs
With the strong encapsulation of JDK internals, many previously accessible APIs are now encapsulated, deprecated, or removed. This presents a challenge for existing codebases that rely on these internal APIs. Developers are required to refactor their code to use supported public APIs, which can be a time-consuming and error-prone process.
2. Limited Access for Legacy Code
Legacy code that depends on encapsulated JDK internals faces significant hurdles. The code may need to be restructured or refactored to align with the encapsulation requirements, which may not always be feasible or practical, especially for large codebases with intricate dependencies on internal APIs.
3. Testing and Mocking Encapsulated APIs
With encapsulation, it becomes more challenging to test code that relies on encapsulated internal APIs. Mocking and simulating behavior for encapsulated classes and methods becomes complex, often requiring the use of reflection or tooling that may be deemed unsafe or inappropriate for production code.
4. Debugging and Troubleshooting
Strong encapsulation can hinder the ability to introspect and debug JDK internals, making it more difficult to diagnose issues that stem from internal JDK functionality or interactions. This complicates the debugging process and may require alternative strategies or workarounds.
5. Overcoming Module Boundaries
While modules provide a clear boundary for encapsulation, there are scenarios where a certain level of access to encapsulated internals is necessary. Interoperability between modules or external tools may require controlled access to encapsulated APIs, necessitating careful management of module boundaries.
Strategies for Mitigating Encapsulation Challenges
To address the challenges posed by strong encapsulation of JDK internals, Java developers can employ a range of strategies and best practices.
1. Embracing Supported APIs
As deprecated and encapsulated APIs are phased out, developers need to embrace and utilize supported public APIs. This may involve updating dependencies, adopting new libraries, or seeking alternative approaches to achieve the desired functionality.
2. Modularity in Legacy Codebases
For legacy codebases, introducing modularity and refactoring existing code to work within module boundaries can help mitigate the impact of encapsulation. This may involve breaking down monolithic code into smaller, more manageable modules.
3. Testing Techniques
To address testing challenges, developers can explore techniques such as dependency injection, interface segregation, and the use of test doubles to decouple and isolate code from encapsulated internals. Additionally, frameworks and tools designed for testing within modular environments can provide valuable support.
4. Debugging Tools and Workarounds
When debugging encapsulated code, developers can leverage IDE features, logging, and debugging tools that offer insights into module interactions and runtime behavior. Understanding the boundaries of encapsulation and utilizing alternative debugging approaches can help circumvent limitations.
5. Establishing Communication Channels
In cases where controlled access to encapsulated APIs is necessary, establishing communication channels between modules or leveraging official extension mechanisms can facilitate the required interactions while adhering to encapsulation principles.
Final Considerations
Strong encapsulation of JDK internals has significantly impacted the way Java developers design, develop, and maintain their applications. While it introduces challenges, it also underscores the importance of writing modular, maintainable, and resilient code. By embracing best practices, leveraging new features, and fostering a modular mindset, developers can navigate the complexities of encapsulation to build robust and future-proof Java applications.
In the ever-evolving landscape of Java development, understanding and adapting to the principles of strong encapsulation is not just a necessity but an opportunity to create more secure, reliable, and scalable software systems.
By navigating the challenges of encapsulation, Java developers can continue to innovate and thrive in an environment that prioritizes security, stability, and modularity.
For further details on JDK encapsulation, refer to the official Java Platform, Standard Edition - JEP 261.
// Example of using a supported public API instead of an encapsulated internal API
// Encapsulated internal API (Java 8 and earlier)
sun.misc.BASE64Encoder encoder = new sun.misc.BASE64Encoder();
// Supported public API (Java 11 and later)
java.util.Base64.Encoder encoder = java.util.Base64.getEncoder();