Making Your Code Flexible with Visitor Pattern
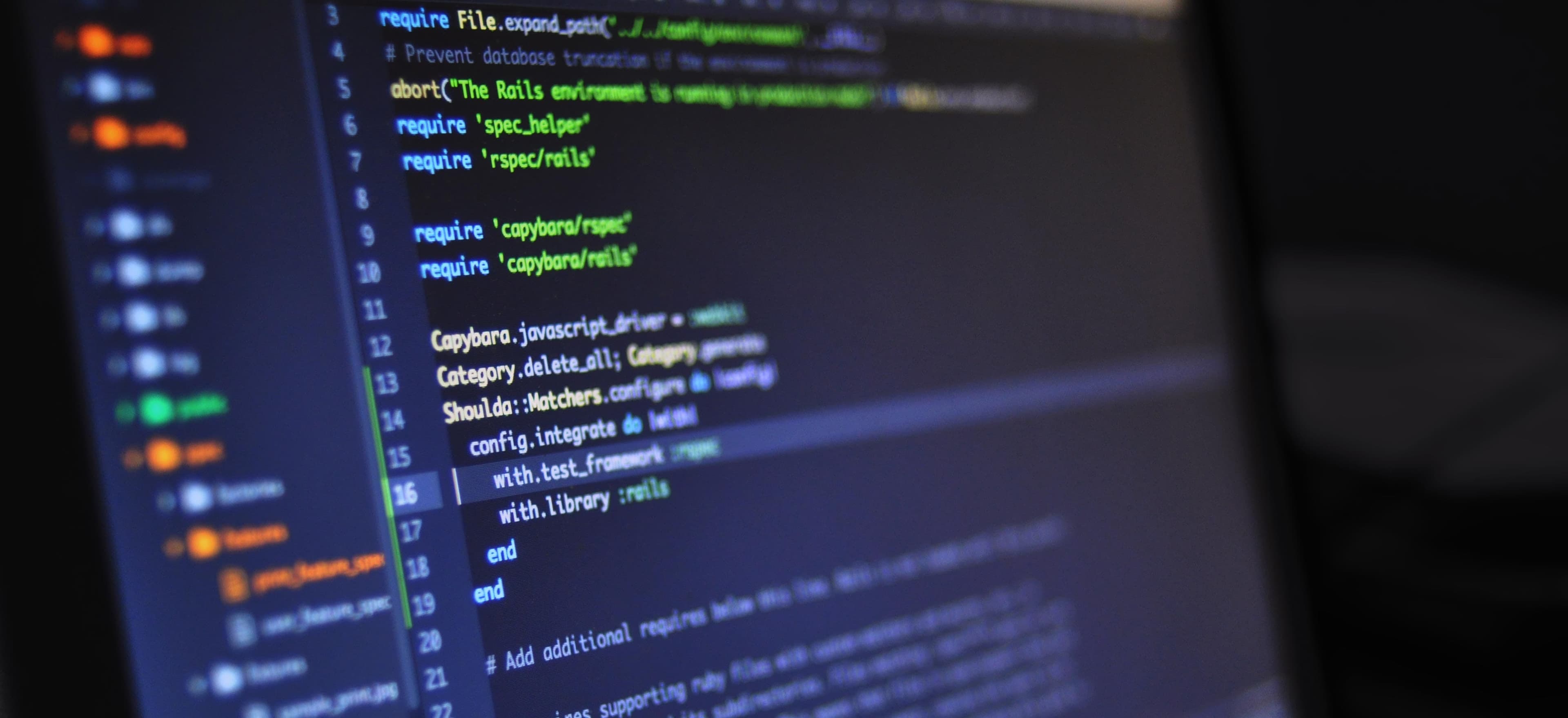
- Published on
Making Your Code Flexible with Visitor Pattern
In the world of software development, writing flexible and extensible code is paramount. One of the common challenges developers face is how to add new operations to classes without altering their structure. The Visitor pattern provides an elegant solution to this problem by separating the operations from the object structure on which they operate. This pattern allows for new operations to be added without modifying the classes of the elements on which they operate, promoting code flexibility and maintainability.
What is the Visitor Pattern?
The Visitor pattern, a behavioral design pattern, enables the definition of new operations on a collection of objects without changing their structure. It achieves this by separating the algorithm from the object structure on which it operates. This pattern is particularly useful when dealing with a set of related classes and the operations that need to be performed on them.
How does the Visitor Pattern Work?
At the core of the Visitor pattern are the elements that need to be visited, the visitor interface, and concrete implementations of the visitor interface. The elements accept visits from visitors, and the visitors perform operations on these elements.
UML Diagram of the Visitor Pattern
Let's look at a simple UML diagram representing the Visitor pattern:
```plantuml
@startuml
interface Visitor {
{abstract} +visit(ElementA a)
{abstract} +visit(ElementB b)
}
class ConcreteVisitorA {
+visit(ElementA a)
+visit(ElementB b)
}
class ConcreteVisitorB {
+visit(ElementA a)
+visit(ElementB b)
}
interface Element {
{abstract} +accept(Visitor v)
}
class ElementA {
+accept(Visitor v)
}
class ElementB {
+accept(Visitor v)
}
Visitor <|-- ConcreteVisitorA
Visitor <|-- ConcreteVisitorB
Element <|-- ElementA
Element <|-- ElementB
ConcreteVisitorA ..> ElementA
ConcreteVisitorA ..> ElementB
ConcreteVisitorB ..> ElementA
ConcreteVisitorB ..> ElementB
@enduml
```plaintext
In this UML representation:
- The
Visitor
interface declares a visit method for each concrete element type. - The
ConcreteVisitorA
andConcreteVisitorB
classes implement theVisitor
interface and provide their own implementation for each visit method. - The
Element
interface declares an accept method that takes aVisitor
as an argument. - The
ElementA
andElementB
classes implement theElement
interface and provide an implementation for the accept method, forwarding the call to the appropriate visit method of the visitor.
Implementing the Visitor Pattern in Java
Let's explore an example to illustrate the implementation of the Visitor pattern in Java. We will create a simple scenario involving two elements, Circle
and Square
, and two visitors, AreaCalculator
and PerimeterCalculator
. The Visitors will calculate area and perimeter for the shapes.
Define the Visitor Interface
We start by defining the Visitor interface that declares the visit method for each element type.
public interface ShapeVisitor {
void visit(Circle circle);
void visit(Square square);
}
Implement the Concrete Visitors
Next, we implement the concrete visitor classes, AreaCalculator
and PerimeterCalculator
, which will provide the concrete implementation for the visit method for each shape.
public class AreaCalculator implements ShapeVisitor {
@Override
public void visit(Circle circle) {
// Calculate area for circle
}
@Override
public void visit(Square square) {
// Calculate area for square
}
}
public class PerimeterCalculator implements ShapeVisitor {
@Override
public void visit(Circle circle) {
// Calculate perimeter for circle
}
@Override
public void visit(Square square) {
// Calculate perimeter for square
}
}
Define the Element Interface
We then define the Element interface that declares the accept
method which takes a ShapeVisitor
as an argument.
public interface Shape {
void accept(ShapeVisitor visitor);
}
Implement the Concrete Elements
Next, we implement the concrete elements, Circle
and Square
, which will provide the implementation for the accept method and forward the call to the appropriate visit method of the visitor.
public class Circle implements Shape {
@Override
public void accept(ShapeVisitor visitor) {
visitor.visit(this);
}
}
public class Square implements Shape {
@Override
public void accept(ShapeVisitor visitor) {
visitor.visit(this);
}
}
Using the Visitor Pattern
Now, let's see how we can use the implemented Visitor pattern to calculate the area and perimeter of shapes.
public class Main {
public static void main(String[] args) {
Shape circle = new Circle();
Shape square = new Square();
ShapeVisitor areaCalculator = new AreaCalculator();
ShapeVisitor perimeterCalculator = new PerimeterCalculator();
circle.accept(areaCalculator);
square.accept(areaCalculator);
circle.accept(perimeterCalculator);
square.accept(perimeterCalculator);
}
}
In the example above, the Main
class creates instances of Circle
and Square
and visitor instances of AreaCalculator
and PerimeterCalculator
. The accept
method of each shape is called with the respective visitor, allowing the visitors to perform the required calculations without modifying the shape classes.
Advantages of Using the Visitor Pattern
The Visitor pattern offers several advantages, including:
- Separation of concerns: Operations are isolated within visitor classes, promoting the single responsibility principle.
- Adding new operations: New operations can be added without altering the classes of the elements. This makes it easy to extend the functionality of the elements without modifying their structure.
- Maintainability: The Visitor pattern helps in keeping the codebase maintainable by allowing changes in operations to be confined to specific visitor classes.
My Closing Thoughts on the Matter
In conclusion, the Visitor pattern is a powerful tool for making code more flexible and extensible. By separating operations from the elements they operate on, the pattern helps in adding new operations to classes without modifying their structure. This not only promotes code flexibility and maintainability but also adheres to the principle of separation of concerns. While the Visitor pattern may introduce additional classes and interfaces, the benefits it brings in terms of code flexibility and maintainability make it a valuable addition to a developer's toolkit.
Using the Visitor pattern can significantly improve the design of your code, making it easier to add new operations and maintain existing ones. By achieving a clear separation between the algorithm and the object structure, the Visitor pattern is a great choice for situations where the design may evolve, and new operations need to be added without altering the existing classes.
In summary, the Visitor pattern is indeed a valuable asset in a developer's arsenal for writing flexible and maintainable code.
Remember, when faced with the challenge of adding new operations to existing classes without altering their structure, the Visitor pattern might just be the elegant solution you need.
So, why not start embracing the power of the Visitor pattern in your Java projects today?
Start making your code more flexible with the Visitor pattern!