Handling WebSocket Backpressure in Akka Streams
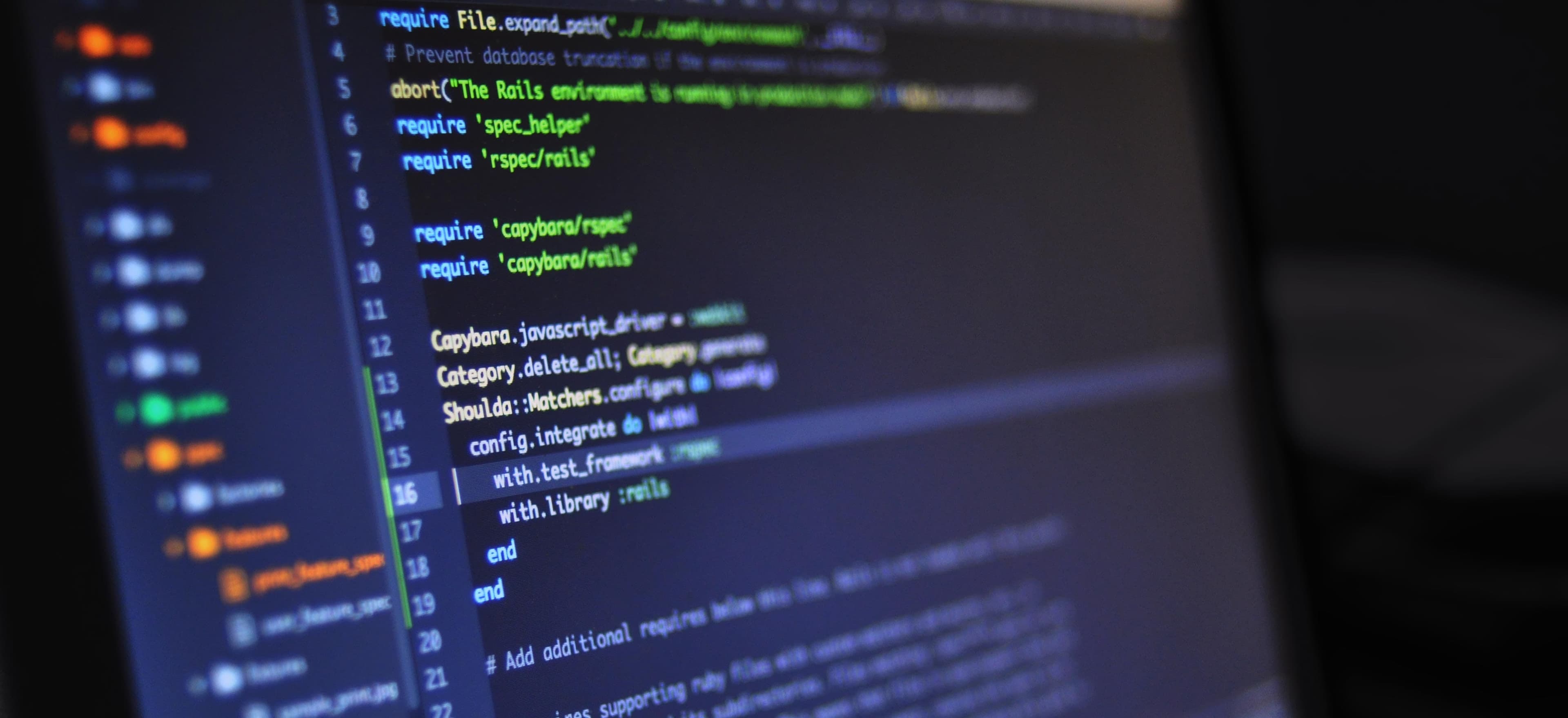
- Published on
Understanding Backpressure in WebSocket with Akka Streams
WebSocket is a powerful communication protocol that enables real-time, bidirectional communication between a client and a server. When implementing WebSocket in Java, it's crucial to consider the potential issue of backpressure. In this article, we'll delve into the concept of backpressure and explore how to handle it effectively in WebSocket communication using Akka Streams.
What is Backpressure?
Backpressure is a mechanism used to regulate the flow of data in a system to prevent overwhelming the downstream components with more data than they can handle. In the context of WebSocket, backpressure is essential to ensure that the server and the client communicate at a pace that both can handle, avoiding potential bottlenecks or out-of-memory errors.
Akka Streams and WebSocket
Akka Streams is a powerful library for building asynchronous, non-blocking, and backpressure-aware stream processing applications. It provides a high-level DSL for defining data transformation pipelines and comes with built-in support for handling backpressure.
When working with WebSocket in a Java application, Akka Streams offers a convenient way to integrate WebSocket communication into a stream processing pipeline while automatically managing backpressure.
Handling Backpressure in WebSocket with Akka Streams
Let's consider a scenario where we have a WebSocket server built using Akka HTTP, and we want to process incoming WebSocket messages using Akka Streams. Our goal is to ensure that backpressure is handled efficiently to prevent overwhelming the system with excessive data.
Below is an example of how to handle WebSocket backpressure in a Java application using Akka Streams:
Setting up the WebSocket
First, we need to set up the WebSocket server using Akka HTTP:
import akka.actor.ActorSystem;
import akka.http.javadsl.*;
import akka.http.javadsl.model.*;
import akka.http.javadsl.server.*;
import akka.stream.javadsl.*;
public class WebSocketServer {
public static void main(String[] args) {
ActorSystem system = ActorSystem.create();
Materializer materializer = ActorMaterializer.create(system);
Http.get(system).newServerAt("localhost", 8080)
.bind(WebSocketServer.createRoute())
.thenCompose(binding -> System.out.println("WebSocket server running on " + binding.localAddress()))
.exceptionally(ex -> {
System.err.println("Failed to bind WebSocket server route: " + ex.getMessage());
system.terminate();
return null;
});
}
private static Route createRoute() {
return path("websocket", () ->
handleWebSocketMessages(WebSocketHandler.create()));
}
}
In this code snippet, we create a WebSocket server running on localhost
at port 8080
and define a route to handle WebSocket messages using handleWebSocketMessages
.
Implementing the WebSocketHandler
Next, we'll implement the WebSocketHandler
to process incoming WebSocket messages using Akka Streams:
import akka.NotUsed;
import akka.stream.javadsl.Flow;
import akka.stream.javadsl.Sink;
import akka.stream.javadsl.Source;
public class WebSocketHandler {
public static Flow<Message, Message, NotUsed> create() {
return Flow.of(Message.class)
.map(message -> processMessage(message))
.buffer(10, OverflowStrategy.backpressure())
.mapAsyncUnordered(5, message -> CompletableFuture.supplyAsync(() -> heavyProcessing(message)));
}
private static Message processMessage(Message message) {
// Process the incoming message
return message;
}
private static Message heavyProcessing(Message message) {
// Simulate heavy processing
return message;
}
}
Here, we define a Flow
to handle incoming WebSocket messages. We use the buffer
operator with backpressure strategy to control the buffer size, and mapAsyncUnordered
to asyncronously process the messages. These operators help ensure that backpressure is applied and the system can handle the incoming data without overwhelming the downstream components.
Lessons Learned
In this article, we explored the concept of backpressure in WebSocket communication and how to handle it effectively using Akka Streams in Java. By leveraging Akka Streams' backpressure-aware processing capabilities, we can build robust and responsive WebSocket applications that gracefully handle varying loads of data.
It's essential to understand the importance of backpressure in stream processing and utilize the appropriate tools and techniques to manage it effectively, ensuring the stability and reliability of our WebSocket applications.
By applying the principles outlined in this article, you can confidently implement WebSocket communication with Akka Streams in your Java applications, knowing that backpressure is handled efficiently, contributing to the overall responsiveness and performance of your system.