Automating Tests with Selenium IDE: Common Pitfalls
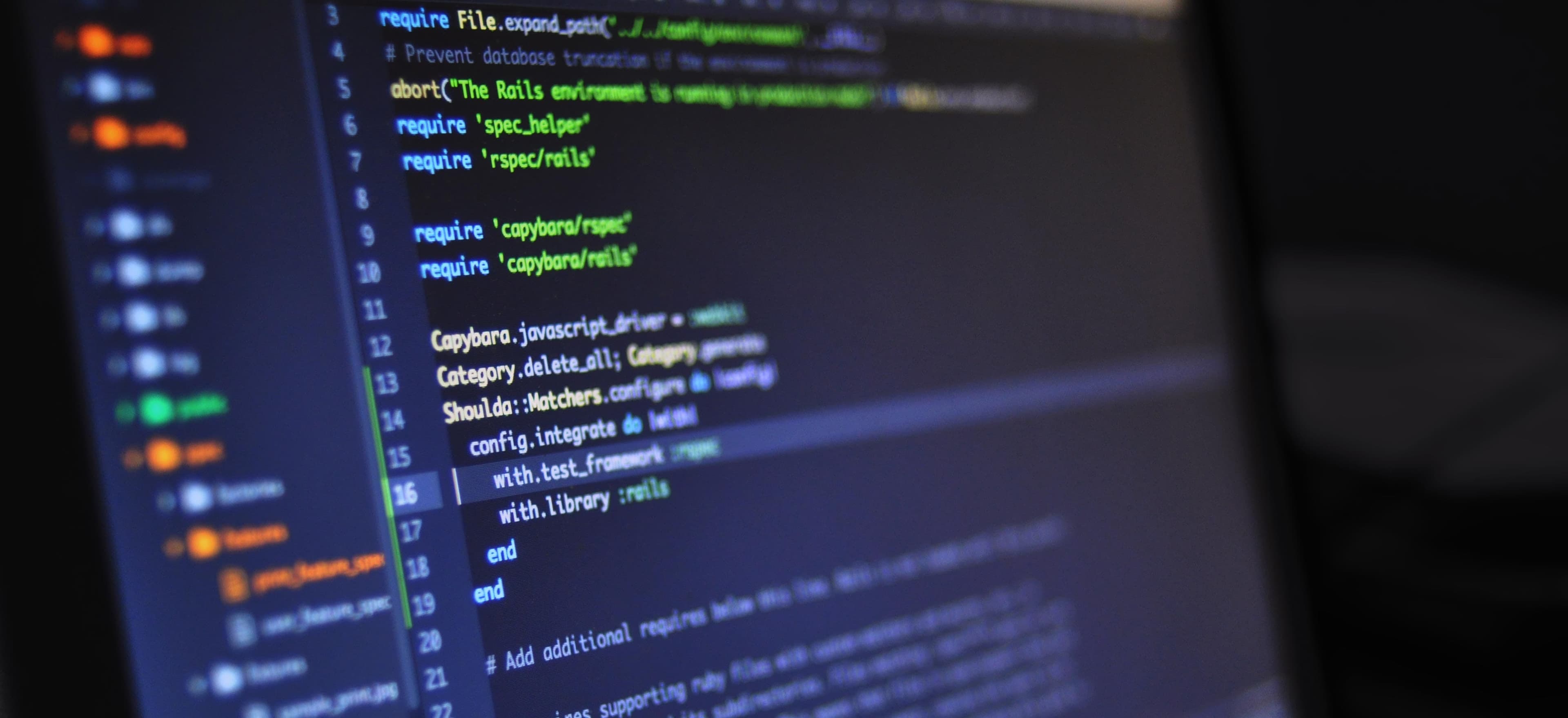
- Published on
Automating Tests with Selenium IDE: Common Pitfalls
Automated testing is an essential aspect of the software development process. Selenium IDE is a widely used tool for automating web applications testing. However, like any tool, it comes with its own set of challenges and common pitfalls that developers and testers may encounter. In this post, we'll explore some of the common pitfalls when using Selenium IDE for test automation, and discuss how to overcome them.
Pitfall 1: Unreliable Selectors
One of the most common pitfalls when using Selenium IDE is relying on unreliable selectors. Selectors are used to identify web elements, such as buttons, input fields, and dropdowns, that the test interacts with. Using selectors that are not unique or are prone to frequent changes can lead to test failures and flakiness.
Solution:
- Use unique and stable selectors, such as
id
orname
attributes, wherever possible. - Avoid using XPath selectors unless necessary, as they can be brittle and slow.
- Use the Selenium IDE's built-in locator strategies to identify elements more effectively.
// Example of using a stable id selector
WebElement element = driver.findElement(By.id("username"));
Pitfall 2: Hardcoded Waits
Another common pitfall is the excessive use of hardcoded waits in test scripts. Hardcoded waits, such as Thread.sleep()
or fixed Implicit
or Explicit
waits, can make tests slower and less reliable. They can also lead to unnecessary delays in test execution.
Solution:
- Use dynamic waits, such as
WebDriverWait
with Expected Conditions, to wait for specific conditions before proceeding with the test. - Set implicit waits at the beginning of the test to define a maximum amount of time to wait for an element to be available.
// Example of using WebDriverWait with Expected Conditions
WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("submit")));
Pitfall 3: Lack of Modularity
Creating monolithic and non-modular test scripts can lead to code duplication and maintenance challenges. Tests that are not modular are harder to maintain and extend, especially as the application grows and changes over time.
Solution:
- Use Page Object Model (POM) to create modular and reusable components for interacting with web pages.
- Structure tests into separate functions and classes for better readability and maintainability.
// Example of using Page Object Model
public class LoginPage {
private WebDriver driver;
By usernameLocator = By.id("username");
By passwordLocator = By.id("password");
By loginButtonLocator = By.id("login");
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void login(String username, String password) {
driver.findElement(usernameLocator).sendKeys(username);
driver.findElement(passwordLocator).sendKeys(password);
driver.findElement(loginButtonLocator).click();
}
}
Pitfall 4: Inadequate Error Handling
Another common pitfall is inadequate error handling in test scripts. Failing to handle errors and exceptions can result in test script failures that provide little insight into the underlying issue, making debugging and troubleshooting more challenging.
Solution:
- Implement proper error handling and reporting mechanisms in test scripts using try-catch blocks and logging frameworks like Log4j.
- Use assertion libraries, such as JUnit or TestNG, to assert and verify expected outcomes in test scripts.
// Example of using JUnit assertions
String actualTitle = driver.getTitle();
String expectedTitle = "Home Page";
assertEquals("Title mismatch", expectedTitle, actualTitle);
Pitfall 5: Limited Cross-Browser Compatibility
Failing to consider cross-browser compatibility during test automation can lead to issues when running tests on different web browsers. Tests that only work on a specific browser may fail when run on others, impacting the reliability and effectiveness of the test suite.
Solution:
- Perform cross-browser testing by executing tests on multiple browsers using Selenium Grid or cloud-based testing platforms, such as Sauce Labs or BrowserStack.
- Use browser-specific capabilities and configurations when setting up the WebDriver for different browsers.
// Example of setting up WebDriver for Chrome browser
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
Pitfall 6: Insufficient Data Handling
Inadequate data handling in test scripts can lead to unreliable and non-repeatable test results. Tests that rely on hardcoded test data or fail to manage test data effectively can be difficult to maintain and scale as the application evolves.
Solution:
- Use test data management strategies, such as data providers and external data sources, to provide test data for different test scenarios.
- Separate test data from test scripts to enable easy maintenance and updates.
// Example of using TestNG data provider
@DataProvider(name = "loginData")
public Object[][] testData() {
return new Object[][] {
{"username1", "password1"},
{"username2", "password2"}
};
}
Closing the Chapter
In conclusion, while Selenium IDE is a powerful tool for automated testing, it's essential to be mindful of the common pitfalls associated with test automation. By addressing these pitfalls and adopting best practices, such as using stable selectors, dynamic waits, modularity, error handling, cross-browser compatibility, and effective data handling, teams can build reliable and maintainable test automation suites using Selenium IDE.
By being aware of these pitfalls and implementing the recommended solutions, teams can significantly improve the effectiveness and reliability of their test automation efforts, ultimately contributing to the overall quality and success of the software development process.
Remember, automation is not just about writing test scripts; it's about writing effective, maintainable, and reliable test scripts.
For more in-depth insight and best practices on Selenium IDE and test automation, check out the official Selenium website: SeleniumHQ.
Happy testing!