Optimizing Garbage Collection in Java
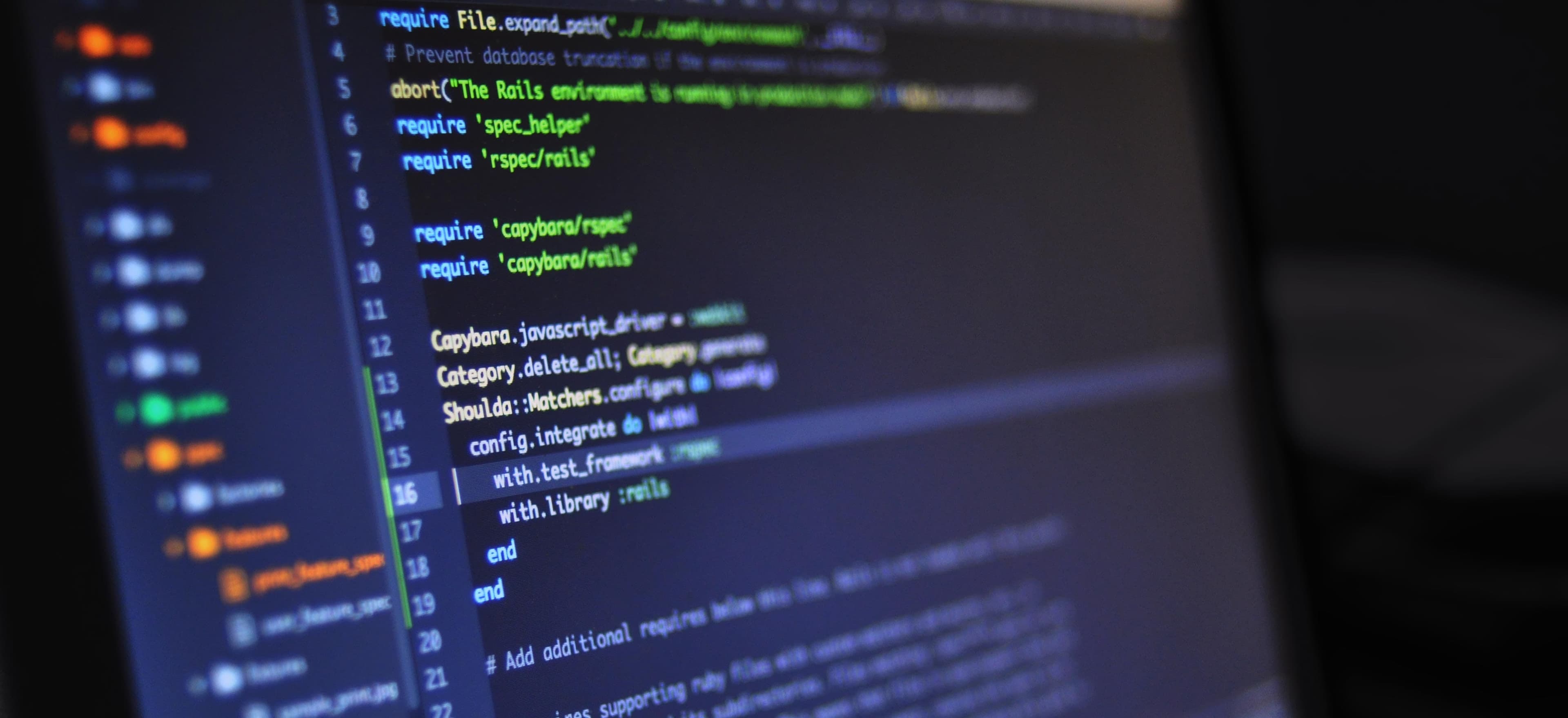
- Published on
Optimizing Garbage Collection in Java
Garbage collection is a fundamental feature of Java that automatically reclaims memory occupied by objects that are no longer in use. While this eliminates the need for manual memory management, inefficient garbage collection can still lead to performance issues. In this blog post, we'll delve into strategies for optimizing garbage collection in Java to enhance application performance.
Understanding Garbage Collection in Java
Before diving into optimization techniques, it's crucial to understand how garbage collection works in Java. When an object is no longer referenced, it becomes eligible for garbage collection. The Java Virtual Machine (JVM) identifies and reclaims such objects, freeing up memory for new allocations.
Types of Garbage Collectors in Java
Java employs different types of garbage collectors, each designed for specific use cases:
-
Serial Garbage Collector: Suitable for single-threaded environments, the serial garbage collector pauses all application threads during garbage collection.
-
Parallel Garbage Collector: Also known as the throughput collector, this collector is designed for multi-threaded applications. It utilizes multiple threads to perform garbage collection, reducing pause times.
-
Concurrent Mark-Sweep (CMS) Garbage Collector: This collector aims to minimize pause times by performing most of its work concurrently with the application threads. However, it can cause fragmentation due to its incremental approach.
-
G1 Garbage Collector: Introduced in Java 7, the G1 collector is designed to provide more predictable pause times by dividing the heap into regions and using a mix of parallel, concurrent, and parallel phases for garbage collection.
Each garbage collector has its own strengths and weaknesses, and choosing the right one depends on the nature of the application and the performance requirements.
Optimizing Garbage Collection
Now, let's explore some effective strategies for optimizing garbage collection in Java.
1. Tweak Heap Sizing Parameters
One of the fundamental aspects of garbage collection optimization is tuning the heap sizing parameters. The heap size, along with the size of the young and old generations, plays a crucial role in garbage collection performance.
-
Xms and Xmx: These parameters control the initial and maximum heap sizes, respectively. Setting them to appropriate values prevents frequent resizing of the heap, reducing overhead.
-
Xmn: This parameter specifies the size of the young generation. A larger young generation can reduce the frequency of minor garbage collections.
Example:
java -Xms1G -Xmx2G -Xmn512M MyApp
In this example, we set the initial heap size to 1GB, the maximum heap size to 2GB, and the size of the young generation to 512MB.
Fine-tuning these parameters based on the application's memory usage patterns can significantly improve garbage collection efficiency.
2. Choose the Right Garbage Collector
Selecting the appropriate garbage collector based on the application's behavior and performance requirements is crucial for optimization.
-
For latency-sensitive applications, the G1 garbage collector is a good choice due to its emphasis on predictable pause times.
-
Throughput-sensitive applications benefit from the parallel garbage collector, which focuses on maximizing overall throughput at the cost of longer pause times.
Example:
java -XX:+UseG1GC MyApp
In this example, we enable the G1 garbage collector for the application.
By aligning the choice of garbage collector with the application's requirements, we can minimize the impact of garbage collection on the application's overall performance.
3. Minimize Object Creation
Excessive object creation can lead to frequent garbage collection cycles. It's essential to minimize unnecessary object allocations, especially within loops and frequently executed code paths.
By reusing objects or using object pooling techniques, we can reduce the burden on the garbage collector and improve overall memory utilization.
Example:
Consider the following code snippet:
List<String> names = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
String name = new String("John");
names.add(name);
}
In this example, the 'new String("John")' statement inside the loop creates a new String object in each iteration, leading to unnecessary memory allocation. By reusing a single instance of the String object, we can optimize memory usage and reduce the impact on garbage collection.
4. Use Profiling Tools
Utilizing profiling tools such as Java Mission Control, VisualVM, or YourKit can provide valuable insights into garbage collection behavior, memory usage, and object allocation patterns.
By analyzing the profiling results, we can identify areas of improvement and fine-tune the application to reduce unnecessary memory overhead and optimize garbage collection.
Example:
By using Java Mission Control to analyze garbage collection logs, we can identify long pause times or excessive memory churn, enabling us to pinpoint areas for optimization.
Key Takeaways
Optimizing garbage collection in Java is essential for enhancing application performance and ensuring efficient memory management. By understanding the intricacies of garbage collection and employing optimization techniques such as heap tuning, appropriate garbage collector selection, object reuse, and profiling, developers can minimize the impact of garbage collection on their applications.
Incorporating these optimization strategies not only improves application responsiveness and scalability but also contributes to a more efficient resource utilization, resulting in a smoother and more responsive user experience.
To delve deeper into Java garbage collection optimization, check out the in-depth guide on Java Garbage Collection Tuning provided by Oracle.
Implementing these strategies in your Java applications will undoubtedly lead to more efficient garbage collection and enhanced overall performance.
Remember, optimizing garbage collection is a continuous process, and staying abreast of the best practices and tools is crucial for maintaining high-performance Java applications.