Understanding the Pitfalls of Over-Reliance on Caching
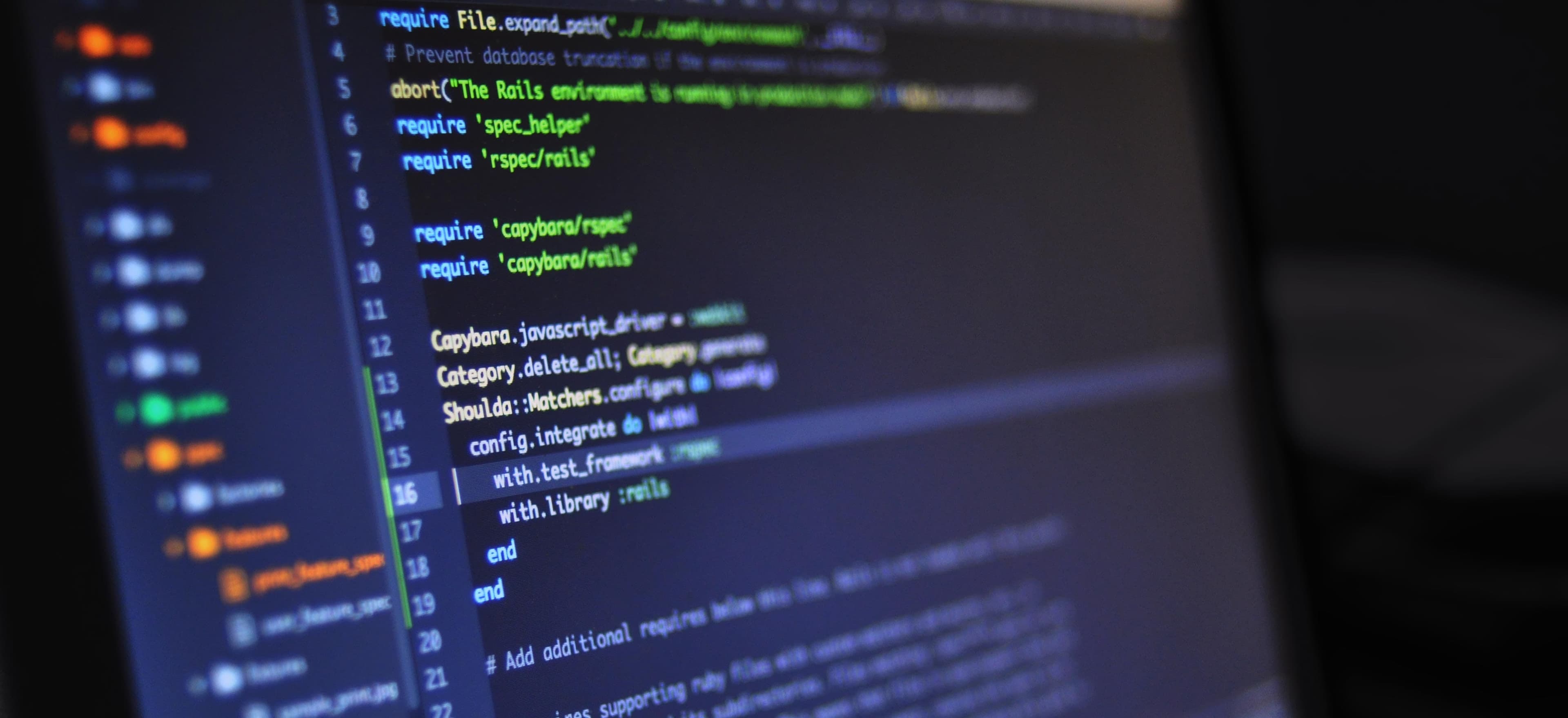
- Published on
The Pitfalls of Over-Reliance on Caching in Java
Caching is a key technique used in Java programming to improve application performance by storing frequently accessed data in memory. While caching offers significant benefits, over-reliance on it can lead to several pitfalls that developers need to be aware of. In this article, we'll explore the potential drawbacks of excessive caching in Java applications and discuss strategies to mitigate these pitfalls.
The Benefits of Caching in Java
Caching is an essential tool for optimizing the performance of Java applications. By storing frequently accessed data in memory, caching reduces the need to retrieve that data from the original source, such as a database or external API. This results in faster response times and improved overall application performance. Additionally, caching can help reduce the load on backend systems, leading to better scalability and cost-efficiency.
Pitfalls of Over-Reliance on Caching
1. Increased Memory Usage
While caching can improve performance, it comes at the cost of increased memory usage. Storing large amounts of data in memory can lead to memory pressure and potential out-of-memory errors, especially in applications running on resource-constrained environments such as cloud-based or containerized deployments.
2. Stale Data
Another significant pitfall of over-reliance on caching is the risk of serving stale data. As data is cached for performance reasons, there is a potential for the cached data to become outdated or inconsistent with the original source. This can lead to incorrect application behavior and erode the integrity of the data.
3. Complexity and Maintenance
Maintaining a robust caching layer adds complexity to the application codebase. As caching logic gets intertwined with business logic, it becomes harder to maintain and reason about the code. Additionally, developers need to ensure cache consistency, handle cache eviction strategies, and deal with cache invalidation, adding further complexity to the application.
4. Cache Invalidation Challenges
Cache invalidation, or the process of ensuring that the cached data is updated or removed when the original data changes, is a non-trivial problem. Over-reliance on caching can lead to challenges in implementing effective cache invalidation strategies, potentially resulting in the serving of outdated or incorrect data.
5. Performance Degradation
Contrary to the intended goal, over-reliance on caching can lead to performance degradation. In scenarios where the cache size grows significantly or the cache eviction strategy is not efficient, the overhead of managing the cache can outweigh the performance benefits, resulting in degraded application performance.
Mitigating the Pitfalls
1. Use Cache with a Strategy
When implementing caching in Java applications, it's crucial to have a clear strategy for what data to cache and for how long. Not all data is suitable for caching, and indiscriminate caching of all data can lead to the pitfalls mentioned earlier. Carefully selecting the data to cache based on access patterns and business requirements is essential.
2. Employ Cache Eviction Policies
Choosing appropriate cache eviction policies, such as least recently used (LRU) or least frequently used (LFU), can help in managing the cache size and ensuring that only the most relevant data is retained in the cache. Understanding the data access patterns and tailoring the eviction policies accordingly is crucial for efficient cache management.
3. Implement Cache Invalidation Mechanisms
Effective cache invalidation mechanisms, such as using cache keys based on the data's unique identifiers and employing event-driven cache invalidation strategies, can help in keeping the cached data synchronized with the original source. This ensures that stale data is minimized, mitigating the risk of serving incorrect information from the cache.
4. Monitor and Tune Cache Performance
Regularly monitoring the cache performance, including hit/miss ratios, eviction rates, and memory consumption, is essential for identifying potential issues. Tuning the cache configuration based on ongoing performance evaluations can help in optimizing the caching layer for the specific needs of the application.
Example of Caching in Java
Let's consider an example of how caching can be implemented in a Java application using the popular Guava Cache library.
import com.google.common.cache.Cache;
import com.google.common.cache.CacheBuilder;
public class DataCache {
private Cache<String, Data> cache;
public DataCache() {
cache = CacheBuilder.newBuilder()
.maximumSize(100)
.build();
}
public Data getData(String key) {
Data data = cache.getIfPresent(key);
if (data == null) {
// Fetch data from the original source
data = fetchDataFromSource(key);
// Cache the data
cache.put(key, data);
}
return data;
}
private Data fetchDataFromSource(String key) {
// Logic to fetch data from the original source
return new Data(key, /* other data */);
}
}
In this example, the DataCache
class utilizes the Guava Cache to store and retrieve Data
objects based on a unique key. The cache is configured with a maximum size, and when retrieving data, it first checks the cache and fetches the data from the original source if not present in the cache.
Wrapping Up
While caching offers significant benefits in improving the performance and scalability of Java applications, over-reliance on caching can lead to several pitfalls, including increased memory usage, serving stale data, complexity, cache invalidation challenges, and performance degradation. To mitigate these pitfalls, developers need to employ a thoughtful caching strategy, utilize appropriate cache eviction policies, implement effective cache invalidation mechanisms, and closely monitor and tune cache performance. By understanding and addressing these pitfalls, developers can leverage caching effectively to enhance the performance and responsiveness of their Java applications.