Optimizing Servlet 3.0 CRUD Operations with Redis and CDI
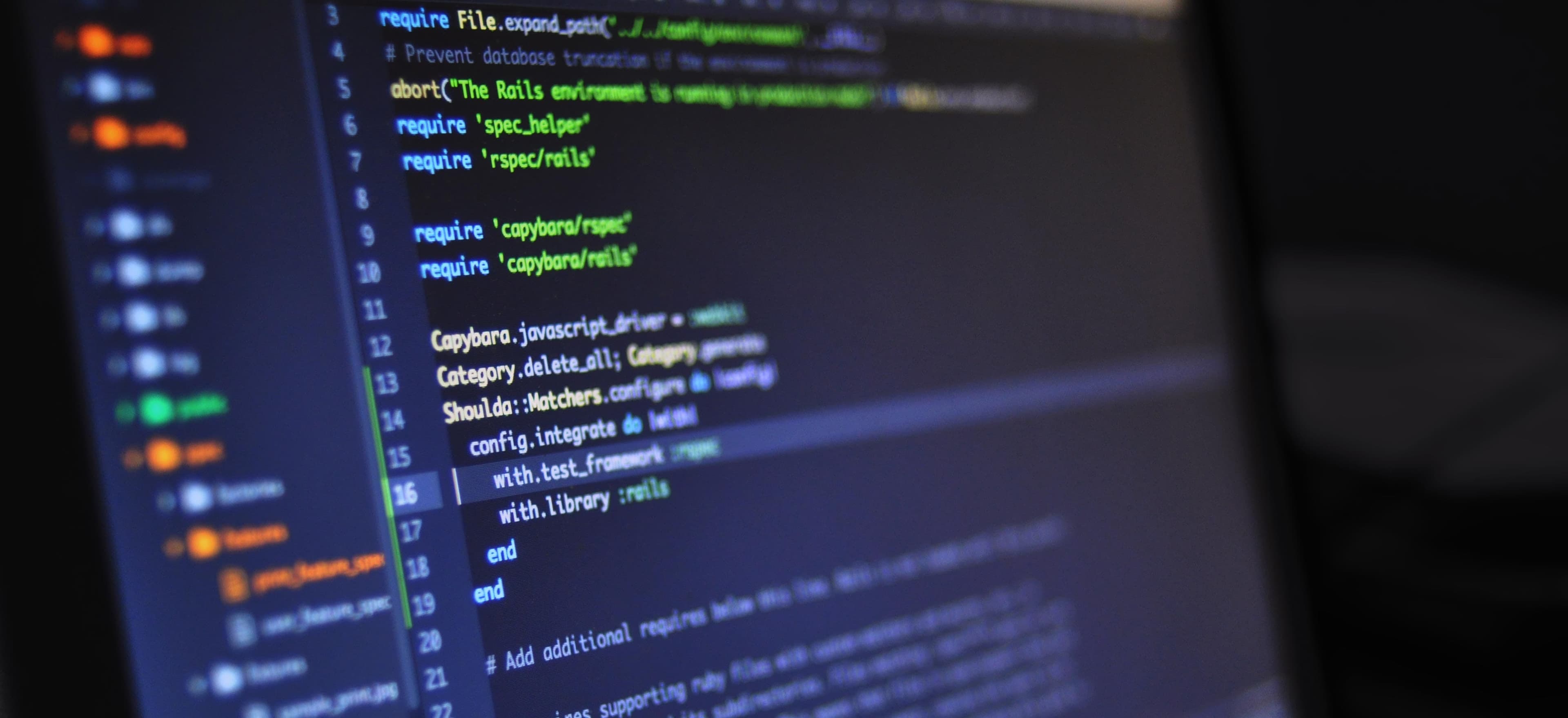
- Published on
Optimizing Servlet 3.0 CRUD Operations with Redis and CDI
As a Java developer, you're likely familiar with the Servlet 3.0 specification, which introduced a more flexible and powerful way to handle web requests. In this blog post, we'll explore how to optimize CRUD (Create, Read, Update, Delete) operations in a Servlet 3.0 application by integrating Redis for fast, in-memory data storage and Contexts and Dependency Injection (CDI) for managing dependencies.
Why Redis?
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. Its blazingly fast read and write operations make it a popular choice for optimizing web applications that require low-latency data access. By integrating Redis into our Servlet application, we can significantly improve the performance of CRUD operations.
Setting Up Redis in a Servlet Application
First, let's add the Jedis library to our project. Jedis is a Java client library for Redis, and it provides a high-level API for interacting with Redis servers.
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.7.0</version>
</dependency>
With Jedis added to the project dependencies, we can now create a Redis client and use it to perform CRUD operations. Here's a basic example of how to set up a Redis client in a Servlet:
import redis.clients.jedis.Jedis;
public class RedisClient {
private Jedis jedis;
public RedisClient() {
this.jedis = new Jedis("localhost");
}
public void set(String key, String value) {
jedis.set(key, value);
}
public String get(String key) {
return jedis.get(key);
}
public void delete(String key) {
jedis.del(key);
}
}
In this example, we create a RedisClient
class and use the Jedis library to connect to a Redis server running on localhost
. We then define methods for setting, getting, and deleting keys in Redis.
Leveraging CDI for Dependency Injection
Next, let's leverage CDI to manage the dependencies in our Servlet application. CDI provides a powerful and flexible way to perform dependency injection, allowing us to decouple our code and make it more testable and maintainable.
First, we need to enable CDI in our Servlet application by adding the following servlet descriptor to our web.xml
file:
<web-app>
<listener>
<listener-class>org.jboss.weld.environment.servlet.Listener</listener-class>
</listener>
</web-app>
With CDI enabled, we can now use annotations to inject dependencies into our Servlet classes. Here's an example of how to inject the RedisClient
into a Servlet using CDI:
import javax.inject.Inject;
import javax.inject.Named;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/example")
public class ExampleServlet extends HttpServlet {
@Inject
private RedisClient redisClient;
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) {
// Use redisClient to perform CRUD operations
}
}
In this example, we use the @Inject
annotation to inject the RedisClient
into our ExampleServlet
class. With this setup, the RedisClient
instance will be automatically created and managed by the CDI container, allowing us to focus on our business logic without worrying about manual instantiation and management of dependencies.
Optimizing CRUD Operations with Redis and CDI
Now that we have Redis set up in our Servlet application and are leveraging CDI for dependency injection, let's optimize our CRUD operations. We'll focus on the following operations: creating a new record, reading an existing record, updating a record, and deleting a record.
Create (Insert) Operation
When creating a new record, we can utilize Redis's SET
command to store the data with a unique key. Here's an example of how to create a new record using the RedisClient
in a Servlet:
redisClient.set("user:1", "John Doe");
In this example, we use the set
method of the RedisClient
to store the name "John Doe" with the key "user:1" in Redis. This creates a new record in our data store.
Read (Retrieve) Operation
To retrieve an existing record, we can use Redis's GET
command to fetch the data associated with a specific key. Here's an example of how to read an existing record using the RedisClient
in a Servlet:
String userName = redisClient.get("user:1");
In this example, we use the get
method of the RedisClient
to retrieve the data associated with the key "user:1" in Redis. The value returned will be "John Doe", which is the name we previously stored.
Update Operation
To update an existing record, we can simply use the SET
command again with the same key to overwrite the existing data. Here's an example of how to update a record using the RedisClient
in a Servlet:
redisClient.set("user:1", "Jane Smith");
In this example, we use the set
method of the RedisClient
to update the name associated with the key "user:1" to "Jane Smith" in Redis.
Delete Operation
To delete a record, we can use Redis's DEL
command to remove the data associated with a specific key. Here's an example of how to delete a record using the RedisClient
in a Servlet:
redisClient.delete("user:1");
In this example, we use the delete
method of the RedisClient
to remove the data associated with the key "user:1" from Redis.
In Conclusion, Here is What Matters
In this blog post, we've explored how to optimize CRUD operations in a Servlet 3.0 application by integrating Redis for fast, in-memory data storage and leveraging CDI for dependency injection. By using Redis for storing and accessing data and CDI for managing dependencies, we can significantly improve the performance and maintainability of our Servlet applications.
As a Java developer, it's essential to stay updated with the latest trends and best practices. By embracing technologies like Redis and CDI, you can ensure that your Servlet applications are well-optimized for efficient CRUD operations.
Start integrating Redis and CDI in your Servlet applications today and experience the performance benefits firsthand!