Optimizing Insertion Sort Algorithm for Efficient Sorting
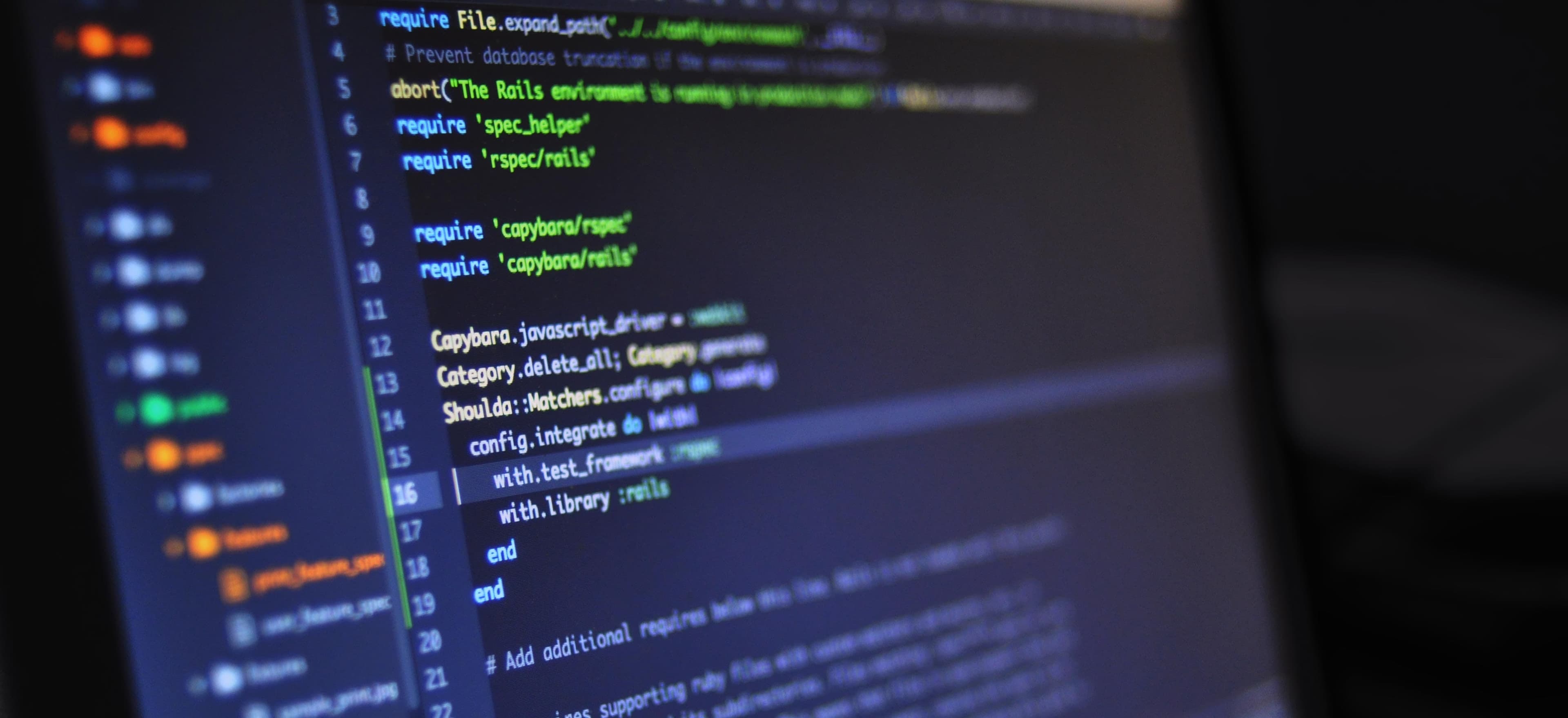
- Published on
Optimizing Insertion Sort Algorithm for Efficient Sorting
When it comes to sorting algorithms, Insertion Sort is a simple and intuitive option. While it is not as efficient as some other sorting algorithms, it still has its place and can be optimized to improve its performance. In this post, we will explore how to optimize the Insertion Sort algorithm in Java, making it more efficient for sorting.
Understanding Insertion Sort
Insertion Sort is a comparison-based sorting algorithm that builds the final sorted array (or list) one item at a time. It iterates through the list, removing one element at a time and finding the place it belongs within the sorted portion of the list.
The basic idea behind Insertion Sort is to divide the input list into a sorted and an unsorted region. It then takes elements from the unsorted region and places them in the correct position in the sorted region. This process continues until the unsorted region becomes empty.
Let's start with a simple implementation of Insertion Sort in Java:
public class InsertionSort {
public void sort(int[] arr) {
int n = arr.length;
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
}
In this basic implementation, we iterate through the array, placing each element in its correct position within the sorted region by shifting the elements greater than the key to the right.
Optimizing the Basic Insertion Sort
While the basic Insertion Sort implementation works, there are opportunities to optimize its performance. One common optimization is to reduce the number of assignments within the inner loop.
Optimized Implementation:
public class InsertionSort {
public void sort(int[] arr) {
int n = arr.length;
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
}
In this optimized version, we have minimized the number of assignments within the inner loop by taking the comparison arr[j] > key
out of the loop condition. This reduces the number of comparisons and assignments, improving the overall performance of the algorithm.
Analyzing the Time Complexity
Worst-Case Time Complexity
In the worst case, the input array is sorted in reverse order, and each element has to be moved to the beginning of the array. In this scenario, the worst-case time complexity is O(n^2).
Best-Case Time Complexity
In the best-case scenario, the input array is already sorted. In this case, the time complexity of Insertion Sort is O(n), as there is no need to shift elements around.
Average-Case Time Complexity
The average-case time complexity of Insertion Sort is also O(n^2). While it performs better than other O(n^2) algorithms like Bubble Sort and Selection Sort, it is still not as efficient as O(n log n) algorithms like Quick Sort or Merge Sort.
Utilizing Binary Search for Insertion
Another optimization technique for Insertion Sort is to use binary search to find the correct position for the key element within the sorted region. This can reduce the number of comparisons needed to place the key in its correct position.
Implementation Using Binary Search:
public class InsertionSort {
public void sort(int[] arr) {
int n = arr.length;
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
int position = binarySearch(arr, 0, j, key);
while (j >= position) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
private int binarySearch(int[] arr, int low, int high, int key) {
while (low < high) {
int mid = (low + high) / 2;
if (arr[mid] < key) {
low = mid + 1;
} else {
high = mid;
}
}
return low;
}
}
In this implementation, we use a binary search algorithm to find the correct position of the key element within the sorted region. This reduces the number of comparisons needed to place the key in its correct position, making the sorting process more efficient.
Closing Remarks
While Insertion Sort may not be the most efficient sorting algorithm, it still has its advantages, especially for small input sizes and nearly sorted arrays. By optimizing the basic Insertion Sort algorithm and utilizing techniques such as reducing assignments and employing binary search, we can improve its performance and make it more competitive with other sorting algorithms.
Optimizing Insertion Sort for efficiency involves understanding its basic implementation, analyzing its time complexity, and utilizing techniques such as binary search for insertion. By doing so, we can leverage its simplicity and make it a viable option for various sorting scenarios.
In summary, optimizing Insertion Sort is about finding the balance between simplicity and efficiency, making it a valuable addition to the toolbox of sorting algorithms.
As you delve into the world of sorting algorithms, understanding their intricacies and optimizations can lead to more informed decision-making in selecting the right algorithm for specific use cases. Happy coding!
Checkout our other articles